
ex
Fork of mbed-os-example-mbed5-blinky by
Embed:
(wiki syntax)
Show/hide line numbers
fs.h
00001 00002 00003 #ifndef _MBED_FS_H_ 00004 #define _MBED_FS_H_ 00005 00006 /* sl_FsOpen options */ 00007 /* Open for Read */ 00008 #define FS_MODE_OPEN_READ 0 00009 /* Open for Write (in case file exist) */ 00010 #define FS_MODE_OPEN_WRITE 1 00011 00012 00013 /*****************************************************************************/ 00014 /* Function prototypes */ 00015 /*****************************************************************************/ 00016 00017 /*! 00018 \brief open file for read or write from/to storage device 00019 00020 \param[in] pFileName File Name buffer pointer 00021 \param[in] AccessModeAndMaxSize Options: As described below 00022 \param[in] pToken Reserved for future use. Use NULL for this field 00023 \param[out] pFileHandle Pointing on the file and used for read and write commands to the file 00024 00025 AccessModeAndMaxSize possible input \n 00026 FS_MODE_OPEN_READ - Read a file \n 00027 FS_MODE_OPEN_WRITE - Open for write for an existing file \n 00028 FS_MODE_OPEN_CREATE(maxSizeInBytes,accessModeFlags) - Open for creating a new file. Max file size is defined in bytes. \n 00029 For optimal FS size, use max size in 4K-512 bytes steps (e.g. 3584,7680,117760) \n 00030 Several access modes bits can be combined together from SlFileOpenFlags_e enum 00031 00032 \return On success, zero is returned. On error, an error code is returned 00033 00034 \sa sl_FsRead sl_FsWrite sl_FsClose 00035 \note belongs to \ref basic_api 00036 \warning 00037 \par Example: 00038 \code 00039 char* DeviceFileName = "MyFile.txt"; 00040 unsigned long MaxSize = 63 * 1024; //62.5K is max file size 00041 long DeviceFileHandle = -1; 00042 long RetVal; //negative retval is an error 00043 unsigned long Offset = 0; 00044 unsigned char InputBuffer[100]; 00045 00046 // Create a file and write data. The file in this example is secured, without signature and with a fail safe commit 00047 RetVal = sl_FsOpen((unsigned char *)DeviceFileName, 00048 FS_MODE_OPEN_CREATE(MaxSize , _FS_FILE_OPEN_FLAG_NO_SIGNATURE_TEST | _FS_FILE_OPEN_FLAG_COMMIT ), 00049 NULL, &DeviceFileHandle); 00050 00051 Offset = 0; 00052 //Preferred in secure file that the Offset and the length will be aligned to 16 bytes. 00053 RetVal = sl_FsWrite( DeviceFileHandle, Offset, (unsigned char *)"HelloWorld", strlen("HelloWorld")); 00054 00055 RetVal = sl_FsClose(DeviceFileHandle, NULL, NULL , 0); 00056 00057 // open the same file for read, using the Token we got from the creation procedure above 00058 RetVal = sl_FsOpen((unsigned char *)DeviceFileName, 00059 FS_MODE_OPEN_READ, 00060 NULL, &DeviceFileHandle); 00061 00062 Offset = 0; 00063 RetVal = sl_FsRead( DeviceFileHandle, Offset, (unsigned char *)InputBuffer, strlen("HelloWorld")); 00064 00065 RetVal = sl_FsClose(DeviceFileHandle, NULL, NULL , 0); 00066 00067 \endcode 00068 */ 00069 int32_t sl_FsOpen(const uint8_t *pFileName,const uint32_t AccessModeAndMaxSize,uint32_t *pToken,int32_t *pFileHandle); 00070 00071 /*! 00072 \brief close file in storage device 00073 00074 \param[in] FileHdl Pointer to the file (assigned from sl_FsOpen) 00075 \param[in] pCeritificateFileName Reserved for future use. Use NULL. 00076 \param[in] pSignature Reserved for future use. Use NULL. 00077 \param[in] SignatureLen Reserved for future use. Use 0. 00078 00079 00080 \return On success, zero is returned. On error, an error code is returned 00081 00082 \sa sl_FsRead sl_FsWrite sl_FsOpen 00083 \note Call the fs_Close with signature = 'A' signature len = 1 for activating an abort action 00084 \warning 00085 \par Example: 00086 \code 00087 sl_FsClose(FileHandle,0,0,0); 00088 \endcode 00089 */ 00090 int16_t sl_FsClose(const int32_t FileHdl,const uint8_t* pCeritificateFileName,const uint8_t* pSignature,const uint32_t SignatureLen); 00091 00092 /*! 00093 \brief Read block of data from a file in storage device 00094 00095 \param[in] FileHdl Pointer to the file (assigned from sl_FsOpen) 00096 \param[in] Offset Offset to specific read block 00097 \param[out] pData Pointer for the received data 00098 \param[in] Len Length of the received data 00099 00100 \return On success, returns the number of read bytes. On error, negative number is returned 00101 00102 \sa sl_FsClose sl_FsWrite sl_FsOpen 00103 \note belongs to \ref basic_api 00104 \warning 00105 \par Example: 00106 \code 00107 Status = sl_FsRead(FileHandle, 0, &readBuff[0], readSize); 00108 \endcode 00109 */ 00110 int32_t sl_FsRead(const int32_t FileHdl, uint32_t Offset , uint8_t* pData, uint32_t Len); 00111 00112 /*! 00113 \brief write block of data to a file in storage device 00114 00115 \param[in] FileHdl Pointer to the file (assigned from sl_FsOpen) 00116 \param[in] Offset Offset to specific block to be written 00117 \param[in] pData Pointer the transmitted data to the storage device 00118 \param[in] Len Length of the transmitted data 00119 00120 \return On success, returns the number of written bytes. On error, an error code is returned 00121 00122 \sa 00123 \note belongs to \ref basic_api 00124 \warning 00125 \par Example: 00126 \code 00127 Status = sl_FsWrite(FileHandle, 0, &buff[0], readSize); 00128 \endcode 00129 */ 00130 int32_t sl_FsWrite(const int32_t FileHdl, uint32_t Offset, uint8_t* pData, uint32_t Len); 00131 00132 /*! 00133 \brief get info on a file 00134 00135 \param[in] pFileName File name 00136 \param[in] Token Reserved for future use. Use 0 00137 \param[out] pFsFileInfo Returns the File's Information: flags,file size, allocated size and Tokens 00138 00139 \return On success, zero is returned. On error, an error code is returned 00140 00141 \sa sl_FsOpen 00142 \note belongs to \ref basic_api 00143 \warning 00144 \par Example: 00145 \code 00146 Status = sl_FsGetInfo("FileName.html",0,&FsFileInfo); 00147 \endcode 00148 */ 00149 00150 00151 00152 #endif /* __FS_H__ */ 00153
Generated on Tue Jul 12 2022 16:28:53 by
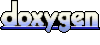