
ex
Fork of mbed-os-example-mbed5-blinky by
Embed:
(wiki syntax)
Show/hide line numbers
coder.h
00001 /* ***** BEGIN LICENSE BLOCK ***** 00002 * Version: RCSL 1.0/RPSL 1.0 00003 * 00004 * Portions Copyright (c) 1995-2002 RealNetworks, Inc. All Rights Reserved. 00005 * 00006 * The contents of this file, and the files included with this file, are 00007 * subject to the current version of the RealNetworks Public Source License 00008 * Version 1.0 (the "RPSL") available at 00009 * http://www.helixcommunity.org/content/rpsl unless you have licensed 00010 * the file under the RealNetworks Community Source License Version 1.0 00011 * (the "RCSL") available at http://www.helixcommunity.org/content/rcsl, 00012 * in which case the RCSL will apply. You may also obtain the license terms 00013 * directly from RealNetworks. You may not use this file except in 00014 * compliance with the RPSL or, if you have a valid RCSL with RealNetworks 00015 * applicable to this file, the RCSL. Please see the applicable RPSL or 00016 * RCSL for the rights, obligations and limitations governing use of the 00017 * contents of the file. 00018 * 00019 * This file is part of the Helix DNA Technology. RealNetworks is the 00020 * developer of the Original Code and owns the copyrights in the portions 00021 * it created. 00022 * 00023 * This file, and the files included with this file, is distributed and made 00024 * available on an 'AS IS' basis, WITHOUT WARRANTY OF ANY KIND, EITHER 00025 * EXPRESS OR IMPLIED, AND REALNETWORKS HEREBY DISCLAIMS ALL SUCH WARRANTIES, 00026 * INCLUDING WITHOUT LIMITATION, ANY WARRANTIES OF MERCHANTABILITY, FITNESS 00027 * FOR A PARTICULAR PURPOSE, QUIET ENJOYMENT OR NON-INFRINGEMENT. 00028 * 00029 * Technology Compatibility Kit Test Suite(s) Location: 00030 * http://www.helixcommunity.org/content/tck 00031 * 00032 * Contributor(s): 00033 * 00034 * ***** END LICENSE BLOCK ***** */ 00035 00036 /************************************************************************************** 00037 * Fixed-point MP3 decoder 00038 * Jon Recker (jrecker@real.com), Ken Cooke (kenc@real.com) 00039 * June 2003 00040 * 00041 * coder.h - private, implementation-specific header file 00042 **************************************************************************************/ 00043 00044 #ifndef _CODER_H 00045 #define _CODER_H 00046 00047 #include "mp3common.h" 00048 00049 #if defined(_WIN32) && defined(_M_IX86) && (defined (_DEBUG) || defined (REL_ENABLE_ASSERTS)) 00050 #define ASSERT(x) if (!(x)) __asm int 3; 00051 #else 00052 #define ASSERT(x) /* do nothing */ 00053 #endif 00054 00055 #ifndef MAX 00056 #define MAX(a,b) ((a) > (b) ? (a) : (b)) 00057 #endif 00058 00059 #ifndef MIN 00060 #define MIN(a,b) ((a) < (b) ? (a) : (b)) 00061 #endif 00062 00063 /* clip to range [-2^n, 2^n - 1] */ 00064 #define CLIP_2N(y, n) { \ 00065 int sign = (y) >> 31; \ 00066 if (sign != (y) >> (n)) { \ 00067 (y) = sign ^ ((1 << (n)) - 1); \ 00068 } \ 00069 } 00070 00071 #define SIBYTES_MPEG1_MONO 17 00072 #define SIBYTES_MPEG1_STEREO 32 00073 #define SIBYTES_MPEG2_MONO 9 00074 #define SIBYTES_MPEG2_STEREO 17 00075 00076 /* number of fraction bits for pow43Tab (see comments there) */ 00077 #define POW43_FRACBITS_LOW 22 00078 #define POW43_FRACBITS_HIGH 12 00079 00080 #define DQ_FRACBITS_OUT 25 /* number of fraction bits in output of dequant */ 00081 #define IMDCT_SCALE 2 /* additional scaling (by sqrt(2)) for fast IMDCT36 */ 00082 00083 #define HUFF_PAIRTABS 32 00084 #define BLOCK_SIZE 18 00085 #define NBANDS 32 00086 #define MAX_REORDER_SAMPS ((192-126)*3) /* largest critical band for short blocks (see sfBandTable) */ 00087 #define VBUF_LENGTH (17 * 2 * NBANDS) /* for double-sized vbuf FIFO */ 00088 00089 /* additional external symbols to name-mangle for static linking */ 00090 #define SetBitstreamPointer STATNAME(SetBitstreamPointer) 00091 #define GetBits STATNAME(GetBits) 00092 #define CalcBitsUsed STATNAME(CalcBitsUsed) 00093 #define DequantChannel STATNAME(DequantChannel) 00094 #define MidSideProc STATNAME(MidSideProc) 00095 #define IntensityProcMPEG1 STATNAME(IntensityProcMPEG1) 00096 #define IntensityProcMPEG2 STATNAME(IntensityProcMPEG2) 00097 #define PolyphaseMono STATNAME(PolyphaseMono) 00098 #define PolyphaseStereo STATNAME(PolyphaseStereo) 00099 #define FDCT32 STATNAME(FDCT32) 00100 00101 #define ISFMpeg1 STATNAME(ISFMpeg1) 00102 #define ISFMpeg2 STATNAME(ISFMpeg2) 00103 #define ISFIIP STATNAME(ISFIIP) 00104 #define uniqueIDTab STATNAME(uniqueIDTab) 00105 #define coef32 STATNAME(coef32) 00106 #define polyCoef STATNAME(polyCoef) 00107 #define csa STATNAME(csa) 00108 #define imdctWin STATNAME(imdctWin) 00109 00110 #define huffTable STATNAME(huffTable) 00111 #define huffTabOffset STATNAME(huffTabOffset) 00112 #define huffTabLookup STATNAME(huffTabLookup) 00113 #define quadTable STATNAME(quadTable) 00114 #define quadTabOffset STATNAME(quadTabOffset) 00115 #define quadTabMaxBits STATNAME(quadTabMaxBits) 00116 00117 /* map these to the corresponding 2-bit values in the frame header */ 00118 typedef enum { 00119 Stereo = 0x00, /* two independent channels, but L and R frames might have different # of bits */ 00120 Joint = 0x01, /* coupled channels - layer III: mix of M-S and intensity, Layers I/II: intensity and direct coding only */ 00121 Dual = 0x02, /* two independent channels, L and R always have exactly 1/2 the total bitrate */ 00122 Mono = 0x03 /* one channel */ 00123 } StereoMode; 00124 00125 typedef struct _BitStreamInfo { 00126 unsigned char *bytePtr; 00127 unsigned int iCache; 00128 int cachedBits; 00129 int nBytes; 00130 } BitStreamInfo; 00131 00132 typedef struct _FrameHeader { 00133 MPEGVersion ver; /* version ID */ 00134 int layer; /* layer index (1, 2, or 3) */ 00135 int crc; /* CRC flag: 0 = disabled, 1 = enabled */ 00136 int brIdx; /* bitrate index (0 - 15) */ 00137 int srIdx; /* sample rate index (0 - 2) */ 00138 int paddingBit; /* padding flag: 0 = no padding, 1 = single pad byte */ 00139 int privateBit; /* unused */ 00140 StereoMode sMode; /* mono/stereo mode */ 00141 int modeExt; /* used to decipher joint stereo mode */ 00142 int copyFlag; /* copyright flag: 0 = no, 1 = yes */ 00143 int origFlag; /* original flag: 0 = copy, 1 = original */ 00144 int emphasis; /* deemphasis mode */ 00145 int CRCWord; /* CRC word (16 bits, 0 if crc not enabled) */ 00146 00147 const SFBandTable *sfBand; 00148 } FrameHeader; 00149 00150 typedef struct _SideInfoSub { 00151 int part23Length; /* number of bits in main data */ 00152 int nBigvals; /* 2x this = first set of Huffman cw's (maximum amplitude can be > 1) */ 00153 int globalGain; /* overall gain for dequantizer */ 00154 int sfCompress; /* unpacked to figure out number of bits in scale factors */ 00155 int winSwitchFlag; /* window switching flag */ 00156 int blockType; /* block type */ 00157 int mixedBlock; /* 0 = regular block (all short or long), 1 = mixed block */ 00158 int tableSelect[3]; /* index of Huffman tables for the big values regions */ 00159 int subBlockGain[3]; /* subblock gain offset, relative to global gain */ 00160 int region0Count; /* 1+region0Count = num scale factor bands in first region of bigvals */ 00161 int region1Count; /* 1+region1Count = num scale factor bands in second region of bigvals */ 00162 int preFlag; /* for optional high frequency boost */ 00163 int sfactScale; /* scaling of the scalefactors */ 00164 int count1TableSelect; /* index of Huffman table for quad codewords */ 00165 } SideInfoSub; 00166 00167 typedef struct _SideInfo { 00168 int mainDataBegin; 00169 int privateBits; 00170 int scfsi[MAX_NCHAN][MAX_SCFBD]; /* 4 scalefactor bands per channel */ 00171 00172 SideInfoSub sis[MAX_NGRAN][MAX_NCHAN]; 00173 } SideInfo; 00174 00175 typedef struct { 00176 int cbType; /* pure long = 0, pure short = 1, mixed = 2 */ 00177 int cbEndS[3]; /* number nonzero short cb's, per subbblock */ 00178 int cbEndSMax; /* max of cbEndS[] */ 00179 int cbEndL; /* number nonzero long cb's */ 00180 } CriticalBandInfo; 00181 00182 typedef struct _DequantInfo { 00183 int workBuf[MAX_REORDER_SAMPS]; /* workbuf for reordering short blocks */ 00184 CriticalBandInfo cbi[MAX_NCHAN]; /* filled in dequantizer, used in joint stereo reconstruction */ 00185 } DequantInfo; 00186 00187 typedef struct _HuffmanInfo { 00188 int huffDecBuf[MAX_NCHAN][MAX_NSAMP]; /* used both for decoded Huffman values and dequantized coefficients */ 00189 int nonZeroBound[MAX_NCHAN]; /* number of coeffs in huffDecBuf[ch] which can be > 0 */ 00190 int gb[MAX_NCHAN]; /* minimum number of guard bits in huffDecBuf[ch] */ 00191 } HuffmanInfo; 00192 00193 typedef enum _HuffTabType { 00194 noBits, 00195 oneShot, 00196 loopNoLinbits, 00197 loopLinbits, 00198 quadA, 00199 quadB, 00200 invalidTab 00201 } HuffTabType; 00202 00203 typedef struct _HuffTabLookup { 00204 int linBits; 00205 HuffTabType tabType; 00206 } HuffTabLookup; 00207 00208 typedef struct _IMDCTInfo { 00209 int outBuf[MAX_NCHAN][BLOCK_SIZE][NBANDS]; /* output of IMDCT */ 00210 int overBuf[MAX_NCHAN][MAX_NSAMP / 2]; /* overlap-add buffer (by symmetry, only need 1/2 size) */ 00211 int numPrevIMDCT[MAX_NCHAN]; /* how many IMDCT's calculated in this channel on prev. granule */ 00212 int prevType[MAX_NCHAN]; 00213 int prevWinSwitch[MAX_NCHAN]; 00214 int gb[MAX_NCHAN]; 00215 } IMDCTInfo; 00216 00217 typedef struct _BlockCount { 00218 int nBlocksLong; 00219 int nBlocksTotal; 00220 int nBlocksPrev; 00221 int prevType; 00222 int prevWinSwitch; 00223 int currWinSwitch; 00224 int gbIn; 00225 int gbOut; 00226 } BlockCount; 00227 00228 /* max bits in scalefactors = 5, so use char's to save space */ 00229 typedef struct _ScaleFactorInfoSub { 00230 char l[23]; /* [band] */ 00231 char s[13][3]; /* [band][window] */ 00232 } ScaleFactorInfoSub; 00233 00234 /* used in MPEG 2, 2.5 intensity (joint) stereo only */ 00235 typedef struct _ScaleFactorJS { 00236 int intensityScale; 00237 int slen[4]; 00238 int nr[4]; 00239 } ScaleFactorJS; 00240 00241 typedef struct _ScaleFactorInfo { 00242 ScaleFactorInfoSub sfis[MAX_NGRAN][MAX_NCHAN]; 00243 ScaleFactorJS sfjs; 00244 } ScaleFactorInfo; 00245 00246 /* NOTE - could get by with smaller vbuf if memory is more important than speed 00247 * (in Subband, instead of replicating each block in FDCT32 you would do a memmove on the 00248 * last 15 blocks to shift them down one, a hardware style FIFO) 00249 */ 00250 typedef struct _SubbandInfo { 00251 int vbuf[MAX_NCHAN * VBUF_LENGTH]; /* vbuf for fast DCT-based synthesis PQMF - double size for speed (no modulo indexing) */ 00252 int vindex; /* internal index for tracking position in vbuf */ 00253 } SubbandInfo; 00254 00255 /* bitstream.c */ 00256 void SetBitstreamPointer(BitStreamInfo *bsi, int nBytes, unsigned char *buf); 00257 unsigned int GetBits(BitStreamInfo *bsi, int nBits); 00258 int CalcBitsUsed(BitStreamInfo *bsi, unsigned char *startBuf, int startOffset); 00259 00260 /* dequant.c, dqchan.c, stproc.c */ 00261 int DequantChannel(int *sampleBuf, int *workBuf, int *nonZeroBound, FrameHeader *fh, SideInfoSub *sis, 00262 ScaleFactorInfoSub *sfis, CriticalBandInfo *cbi); 00263 void MidSideProc(int x[MAX_NCHAN][MAX_NSAMP], int nSamps, int mOut[2]); 00264 void IntensityProcMPEG1(int x[MAX_NCHAN][MAX_NSAMP], int nSamps, FrameHeader *fh, ScaleFactorInfoSub *sfis, 00265 CriticalBandInfo *cbi, int midSideFlag, int mixFlag, int mOut[2]); 00266 void IntensityProcMPEG2(int x[MAX_NCHAN][MAX_NSAMP], int nSamps, FrameHeader *fh, ScaleFactorInfoSub *sfis, 00267 CriticalBandInfo *cbi, ScaleFactorJS *sfjs, int midSideFlag, int mixFlag, int mOut[2]); 00268 00269 /* dct32.c */ 00270 void FDCT32(int *x, int *d, int offset, int oddBlock, int gb); 00271 00272 /* hufftabs.c */ 00273 extern const HuffTabLookup huffTabLookup[HUFF_PAIRTABS]; 00274 extern const int huffTabOffset[HUFF_PAIRTABS]; 00275 extern const unsigned short huffTable[]; 00276 extern const unsigned char quadTable[64+16]; 00277 extern const int quadTabOffset[2]; 00278 extern const int quadTabMaxBits[2]; 00279 00280 /* polyphase.c (or asmpoly.s) 00281 * some platforms require a C++ compile of all source files, 00282 * so if we're compiling C as C++ and using native assembly 00283 * for these functions we need to prevent C++ name mangling. 00284 */ 00285 #ifdef __cplusplus 00286 extern "C" { 00287 #endif 00288 void PolyphaseMono(short *pcm, int *vbuf, const int *coefBase); 00289 void PolyphaseStereo(short *pcm, int *vbuf, const int *coefBase); 00290 #ifdef __cplusplus 00291 } 00292 #endif 00293 00294 /* trigtabs.c */ 00295 extern const int imdctWin[4][36]; 00296 extern const int ISFMpeg1[2][7]; 00297 extern const int ISFMpeg2[2][2][16]; 00298 extern const int ISFIIP[2][2]; 00299 extern const int csa[8][2]; 00300 extern const int coef32[31]; 00301 extern const int polyCoef[264]; 00302 00303 #endif /* _CODER_H */
Generated on Tue Jul 12 2022 16:28:53 by
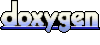