
ex
Fork of mbed-os-example-mbed5-blinky by
Embed:
(wiki syntax)
Show/hide line numbers
baidu_time_calculate.h
00001 #ifndef __BAIDU_TIME_CALCULATE_H__ 00002 #define __BAIDU_TIME_CALCULATE_H__ 00003 00004 #ifdef __cplusplus 00005 extern "C" { 00006 #endif 00007 00008 void add_measure_value(int key, int count); 00009 void update_measure_value(int key, int arg_value, int continuous_measure_flag); 00010 void output_measure_result(); 00011 00012 //**************************************************************************************// 00013 // KEY is number from 0 to 9, because the macor ArraySize is 10. You can enlarge it if you calcute more than 10 sites. 00014 // COUNTER is the biggest measurement times. 00015 // ARGUEMENT is used for speed as ARGUEMENT/TIME_AVERAGE. 00016 // 00017 // !!!!!! Take carefull: KEY in TIME_BEGIN and TIME_END must be the same. !!!!!! 00018 // 00019 // TIME_BEGIN(1, 10); 00020 // printf("ABC"); 00021 // TIME_END(1, 200, UNCONTINUOUS_TIME_MEASURE); 00022 // 00023 // You can call TIME_RESULT() anywhere to print the resutl. 00024 // 00025 //**************************************************************************************// 00026 00027 enum baidu_time_measure_params { 00028 // TIME_END will measure time no matter there is a one-to-one TIME_BEGIN or not 00029 CONTINUOUS_TIME_MEASURE = 0, 00030 // TIME_END will measure time only when there is a one-to-one TIME_BEGIN 00031 UNCONTINUOUS_TIME_MEASURE = 1, 00032 MAX_TIME_CALCULATE_COUNT = 0X7FFFFFFF, 00033 }; 00034 00035 #define TIME_BEGIN(KEY, COUNTER) do{\ 00036 add_measure_value(KEY, COUNTER);\ 00037 }while(0) 00038 00039 #define TIME_END(KEY, ARGUEMENT, FLAG) do{\ 00040 update_measure_value(KEY, ARGUEMENT, FLAG);\ 00041 }while(0) 00042 00043 #define TIME_RESULT() do{\ 00044 output_measure_result();\ 00045 }while(0) 00046 00047 //#define BAIDU_PERFORM_STATISTIC 00048 00049 enum baidu_perform_statistic_watch_point { 00050 PERFORM_STATISTIC_START_RECORD = 1, // from user press start button to start record 00051 PERFORM_STATISTIC_RECORDER_ON_DATA, // time consume of encode and send record data 00052 PERFORM_STATISTIC_READ_VOICE_DATA, // time consume of read voice data from arm 00053 PERFORM_STATISTIC_SOTP_RECORD, // from user stop record to the recorder thread stop 00054 PERFORM_STATISTIC_BASE64_ENCODE, // the time consume of mbedtls_base64_encode 00055 PERFORM_STATISTIC_GET_URL, // the time from user stop record to get media data from URL 00056 // time consume of recorder_output_handler(encode and pass to CA) 00057 PERFORM_STATISTIC_OUTPUT_RECORDER_DATA, 00058 // time consume of bdspx_speex_encode encode data(exclude output encoded data) 00059 PERFORM_STATISTIC_SPEEX_ENCODE, 00060 PERFORM_STATISTIC_GET_MEDIA_BY_URL, // time consume from get media data from url to play it 00061 PERFORM_STATISTIC_PLAY_URL_MEDIA, // time consume of mdm_media_data_out_handler(play url data) 00062 PERFORM_STATISTIC_GET_MEDIA_BY_PATH, // time consume of read local media 00063 PERFORM_STATISTIC_PLAY_LOCAL_MEDIA, // time consume of mdm_media_data_out_handler(play local data) 00064 }; 00065 00066 #ifdef BAIDU_PERFORM_STATISTIC 00067 #define PERFORM_STATISTIC_BEGIN(KEY, COUNTER) TIME_BEGIN(KEY, COUNTER) 00068 #define PERFORM_STATISTIC_END(KEY, ARGUEMENT, FLAG) TIME_END(KEY, ARGUEMENT, FLAG) 00069 #define PERFORM_STATISTIC_RESULT() TIME_RESULT() 00070 #else 00071 #define PERFORM_STATISTIC_BEGIN(KEY, COUNTER) 00072 #define PERFORM_STATISTIC_END(KEY, ARGUEMENT, FLAG) 00073 #define PERFORM_STATISTIC_RESULT() 00074 #endif 00075 00076 #ifdef __cplusplus 00077 } 00078 #endif 00079 00080 #endif //~__BAIDU_TIME_CALCULATE_H__
Generated on Tue Jul 12 2022 16:28:53 by
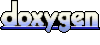