
ex
Fork of mbed-os-example-mbed5-blinky by
Embed:
(wiki syntax)
Show/hide line numbers
baidu_media_play_m4a.h
00001 // Copyright 2017 Baidu Inc. All Rights Reserved. 00002 // Author: Chen Xihao (chenxihao@baidu.com) 00003 // 00004 // Description: Play m4a type file 00005 00006 #ifndef BAIDU_TINYDU_IOT_OS_SRC_MEDIA_PLAYER_BAIDU_MEDIA_PLAY_M4A_H 00007 #define BAIDU_TINYDU_IOT_OS_SRC_MEDIA_PLAYER_BAIDU_MEDIA_PLAY_M4A_H 00008 00009 #include "mp4ff.h" 00010 #include "rda_mp4.h" 00011 #include "baidu_media_type.h" 00012 00013 namespace duer { 00014 00015 const int M4AERR_OK = 0; 00016 const int M4AERR_MAC = -1; 00017 const int M4AERR_ARG = -2; 00018 const int M4AERR_VAL = -3; 00019 00020 class MediaAdapter; 00021 00022 class MediaPlayM4A { 00023 public: 00024 MediaPlayM4A(MediaAdapter* media_adapter); 00025 00026 ~MediaPlayM4A(); 00027 00028 int m4a_file_start(MediaType type, const unsigned char* p_buff, int buff_sz); 00029 00030 int m4a_file_play(const unsigned char* p_buff, int buff_sz); 00031 00032 int m4a_file_end(const unsigned char* p_buff, int buff_sz); 00033 00034 int get_m4a_file_pos(); 00035 00036 private: 00037 MediaPlayM4A(const MediaPlayM4A&); 00038 00039 MediaPlayM4A& operator=(const MediaPlayM4A&); 00040 00041 int m4a_header_save(const unsigned char* p_buff, int buff_sz); 00042 00043 int m4a_header_parse(); 00044 00045 int m4a_frame_to_codec(const unsigned char* p_buff, int buff_sz); 00046 00047 static uint32_t read_callback(void* user_data, void* buffer, uint32_t length); 00048 00049 static uint32_t seek_callback(void* user_data, uint64_t position); 00050 00051 static mp4ff_callback_t _s_mp4cb; 00052 00053 FILE* _p_file_sd; 00054 int _m4a_header_size; 00055 int _buff_data_size; 00056 unsigned char* _p_buff_data; 00057 int _m4a_file_pos; 00058 bool _m4a_parse_done; 00059 00060 int _num_samples; 00061 int _track; 00062 int _sample_id; 00063 mp4AudioSpecificConfig _mp4_asc; 00064 mp4ff_t _infile; 00065 00066 MediaAdapter* _media_adapter; 00067 }; 00068 00069 } // namespace duer 00070 00071 #endif // BAIDU_TINYDU_IOT_OS_SRC_MEDIA_PLAYER_BAIDU_MEDIA_PLAY_M4A_H
Generated on Tue Jul 12 2022 16:28:53 by
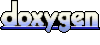