
ex
Fork of mbed-os-example-mbed5-blinky by
Embed:
(wiki syntax)
Show/hide line numbers
baidu_ca_object.h
00001 // Copyright 2017 Baidu Inc. All Rights Reserved. 00002 // Author: Su Hao (suhao@baidu.com) 00003 // 00004 // Description: Object 00005 00006 #ifndef BAIDU_IOT_TINYDU_IOT_OS_SRC_IOT_BAIDU_CA_SCHEDULER_BAIDU_CA_OBJECT_H 00007 #define BAIDU_IOT_TINYDU_IOT_OS_SRC_IOT_BAIDU_CA_SCHEDULER_BAIDU_CA_OBJECT_H 00008 00009 #include <stddef.h> 00010 00011 struct baidu_json; 00012 00013 namespace duer { 00014 00015 class Object { 00016 public: 00017 00018 Object(); 00019 00020 ~Object(); 00021 00022 void reset(); 00023 00024 void putInt(const char* key, int value); 00025 00026 void putDouble(const char* key, double value); 00027 00028 void putString(const char* key, const char* value); 00029 00030 // Note: the life time of value should be longer or equal this 00031 void putObject(const char* key, const Object& value); 00032 00033 void putIntArray(const char* key, int value[], size_t length); 00034 00035 void putDoubleArray(const char* key, double value[], size_t length); 00036 00037 void putStringArray(const char* key, const char* value[], size_t length); 00038 00039 void putObjectArray(const char* key, Object value[], size_t length); 00040 00041 // the owner of object will be transfered to this. 00042 // there should no other Object control the life of object 00043 void receiveObject(const char* key, baidu_json* object); 00044 00045 // give up the ownership of _data, and reset _data to a new one 00046 baidu_json* releaseObject(); 00047 00048 const char* toString(); 00049 00050 private: 00051 00052 Object(const Object& other); 00053 00054 Object& operator =(const Object& other); 00055 00056 baidu_json* _data; 00057 char* _string; 00058 }; 00059 00060 } // namespace duer 00061 00062 #endif // BAIDU_IOT_TINYDU_IOT_OS_SRC_IOT_BAIDU_CA_SCHEDULER_BAIDU_CA_OBJECT_H
Generated on Tue Jul 12 2022 16:28:52 by
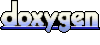