
ex
Fork of mbed-os-example-mbed5-blinky by
Embed:
(wiki syntax)
Show/hide line numbers
baidu_ca_memory.h
00001 // Copyright 2017 Baidu Inc. All Rights Reserved. 00002 // Author: Su Hao (suhao@baidu.com) 00003 // 00004 // Description: The APIs for memory management. 00005 00006 #ifndef BAIDU_IOT_TINYDU_IOT_OS_SRC_IOT_BAIDU_CA_SOURCE_BAIDU_CA_MEMORY_H 00007 #define BAIDU_IOT_TINYDU_IOT_OS_SRC_IOT_BAIDU_CA_SOURCE_BAIDU_CA_MEMORY_H 00008 00009 #include "baidu_ca_types.h" 00010 00011 /* 00012 * malloc function 00013 * 00014 * @Param size, bca_size_t, the expected size of the memory 00015 * @Return the alloced memory pointer 00016 */ 00017 BCA_INT void* bca_malloc(bca_size_t size); 00018 00019 /* 00020 * realloc function 00021 * 00022 * @Param ptr, void *, the old alloced memory 00023 * @Param size, bca_size_t, the expected size of the memory 00024 * @Return the new alloced memory pointer 00025 */ 00026 BCA_INT void* bca_realloc(void* ptr, bca_size_t size); 00027 00028 /* 00029 * free function 00030 * 00031 * @Param ptr, void *, the alloced memory 00032 */ 00033 BCA_INT void bca_free(void* ptr); 00034 00035 /* 00036 * malloc function 00037 * 00038 * @Param size, bca_size_t, the expected size of the memory 00039 * @Param file, const char *, the file name when alloc the memory 00040 * @Param line, bca_u32_t, the line number of the file when alloc the memory 00041 * @Return the alloced memory pointer 00042 */ 00043 BCA_INT void* bca_malloc_ext(bca_size_t size, const char* file, bca_u32_t line); 00044 00045 /* 00046 * realloc function 00047 * 00048 * @Param ptr, void *, the old alloced memory 00049 * @Param size, bca_size_t, the expected size of the memory 00050 * @Param file, const char *, the file name when alloc the memory 00051 * @Param line, bca_u32_t, the line number of the file when alloc the memory 00052 * @Return the new alloced memory pointer 00053 */ 00054 BCA_INT void* bca_realloc_ext(void* ptr, bca_size_t size, const char* file, 00055 bca_u32_t line); 00056 00057 /* 00058 * free function 00059 * 00060 * @Param ptr, void *, the alloced memory 00061 * @Param file, const char *, the file name when alloc the memory 00062 * @Param line, bca_u32_t, the line number of the file when alloc the memory 00063 */ 00064 BCA_INT void bca_free_ext(void* ptr, const char* file, bca_u32_t line); 00065 00066 #ifdef BCA_MEMORY_USAGE 00067 BCA_INT void bca_memdbg_usage(); 00068 #define BCA_MEMDBG_USAGE(...) bca_memdbg_usage() 00069 #else 00070 #define BCA_MEMDBG_USAGE(...) 00071 #endif 00072 00073 #ifdef BCA_MEMORY_DEBUG 00074 #define BCA_MALLOC(_s) bca_malloc_ext(_s, __FILE__, __LINE__) 00075 #define BCA_REALLOC(_p, _s) bca_realloc_ext(_p, _s, __FILE__, __LINE__) 00076 #define BCA_FREE(_p) bca_free_ext(_p, __FILE__, __LINE__) 00077 #else/*BCA_MEMORY_DEBUG*/ 00078 #define BCA_MALLOC(_s) bca_malloc(_s) 00079 #define BCA_REALLOC(_p, _s) bca_realloc(_p, _s) 00080 #define BCA_FREE(_p) bca_free(_p) 00081 #endif/*BCA_MEMORY_DEBUG*/ 00082 00083 #define BCA_CALLOC(_s, _n) BCA_MALLOC((_s) * (_n)) 00084 00085 #endif // BAIDU_IOT_TINYDU_IOT_OS_SRC_IOT_BAIDU_CA_SOURCE_BAIDU_CA_MEMORY_H
Generated on Tue Jul 12 2022 16:28:52 by
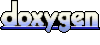