
ex
Fork of mbed-os-example-mbed5-blinky by
Embed:
(wiki syntax)
Show/hide line numbers
assembly.h
00001 /* ***** BEGIN LICENSE BLOCK ***** 00002 * Version: RCSL 1.0/RPSL 1.0 00003 * 00004 * Portions Copyright (c) 1995-2002 RealNetworks, Inc. All Rights Reserved. 00005 * 00006 * The contents of this file, and the files included with this file, are 00007 * subject to the current version of the RealNetworks Public Source License 00008 * Version 1.0 (the "RPSL") available at 00009 * http://www.helixcommunity.org/content/rpsl unless you have licensed 00010 * the file under the RealNetworks Community Source License Version 1.0 00011 * (the "RCSL") available at http://www.helixcommunity.org/content/rcsl, 00012 * in which case the RCSL will apply. You may also obtain the license terms 00013 * directly from RealNetworks. You may not use this file except in 00014 * compliance with the RPSL or, if you have a valid RCSL with RealNetworks 00015 * applicable to this file, the RCSL. Please see the applicable RPSL or 00016 * RCSL for the rights, obligations and limitations governing use of the 00017 * contents of the file. 00018 * 00019 * This file is part of the Helix DNA Technology. RealNetworks is the 00020 * developer of the Original Code and owns the copyrights in the portions 00021 * it created. 00022 * 00023 * This file, and the files included with this file, is distributed and made 00024 * available on an 'AS IS' basis, WITHOUT WARRANTY OF ANY KIND, EITHER 00025 * EXPRESS OR IMPLIED, AND REALNETWORKS HEREBY DISCLAIMS ALL SUCH WARRANTIES, 00026 * INCLUDING WITHOUT LIMITATION, ANY WARRANTIES OF MERCHANTABILITY, FITNESS 00027 * FOR A PARTICULAR PURPOSE, QUIET ENJOYMENT OR NON-INFRINGEMENT. 00028 * 00029 * Technology Compatibility Kit Test Suite(s) Location: 00030 * http://www.helixcommunity.org/content/tck 00031 * 00032 * Contributor(s): 00033 * 00034 * ***** END LICENSE BLOCK ***** */ 00035 00036 /************************************************************************************** 00037 * Fixed-point MP3 decoder 00038 * Jon Recker (jrecker@real.com), Ken Cooke (kenc@real.com) 00039 * June 2003 00040 * 00041 * assembly.h - assembly language functions and prototypes for supported platforms 00042 * 00043 * - inline rountines with access to 64-bit multiply results 00044 * - x86 (_WIN32) and ARM (ARM_ADS, _WIN32_WCE) versions included 00045 * - some inline functions are mix of asm and C for speed 00046 * - some functions are in native asm files, so only the prototype is given here 00047 * 00048 * MULSHIFT32(x, y) signed multiply of two 32-bit integers (x and y), returns top 32 bits of 64-bit result 00049 * FASTABS(x) branchless absolute value of signed integer x 00050 * CLZ(x) count leading zeros in x 00051 * MADD64(sum, x, y) (Windows only) sum [64-bit] += x [32-bit] * y [32-bit] 00052 * SHL64(sum, x, y) (Windows only) 64-bit left shift using __int64 00053 * SAR64(sum, x, y) (Windows only) 64-bit right shift using __int64 00054 */ 00055 00056 #ifndef _ASSEMBLY_H 00057 #define _ASSEMBLY_H 00058 00059 #include <stdint.h> 00060 00061 #define RDA5991H 00062 00063 #if defined(RDA5991H) 00064 00065 #if defined(__ICCARM__) 00066 #define __inline __INLINE 00067 #endif 00068 00069 #if 1 00070 static __inline int FASTABS(int x) 00071 { 00072 return((x > 0) ? x : -(x)); 00073 } 00074 #endif 00075 00076 #if 0 00077 static __inline int CLZ(int x) 00078 { 00079 return __CLZ(x); 00080 } 00081 #endif 00082 00083 #if 0 00084 static __inline int CLZ(int x) 00085 { 00086 int numZeros; 00087 00088 if (!x) 00089 return (sizeof(int) * 8); 00090 00091 numZeros = 0; 00092 while (!(x & 0x80000000)) { 00093 numZeros++; 00094 x <<= 1; 00095 } 00096 00097 return numZeros; 00098 } 00099 #endif 00100 00101 #if 1 00102 static __inline int CLZ(int x) 00103 { 00104 int numZeros; 00105 00106 if (!x) 00107 return (sizeof(int) * 8); 00108 00109 __asm{ 00110 CLZ numZeros, x 00111 } 00112 00113 return numZeros; 00114 } 00115 #endif 00116 00117 #if 1 00118 static __inline int64_t SAR64(int64_t x, int n) 00119 { 00120 return (x >> n); 00121 } 00122 #endif 00123 00124 #if 1 00125 static __inline int MULSHIFT32(int x, int y) 00126 { 00127 int64_t tmp; 00128 00129 tmp = ((int64_t)x * (int64_t)y); 00130 return (tmp>>32); 00131 } 00132 #endif 00133 00134 #if 0 00135 static int __inline MULSHIFT32(int x, int y) 00136 { 00137 int tmp; 00138 00139 __asm{ 00140 SMULL tmp, y, x, y 00141 } 00142 00143 return y; 00144 } 00145 #endif 00146 00147 #if 1 00148 static __inline int64_t MADD64(int64_t sum64, int x, int y) 00149 { 00150 return (sum64 + (int64_t)x * (int64_t)y); 00151 } 00152 #endif 00153 00154 #if 0 00155 static __inline int64_t MADD64(int64_t sum64, int x, int y) 00156 { 00157 unsigned int sum64Lo = ((unsigned int *)&sum64)[0]; 00158 int sum64Hi = ((int *)&sum64)[1]; 00159 00160 __asm{ 00161 SMLAL sum64Lo, sum64Hi, x, y 00162 } 00163 00164 sum64 = (((int64_t) sum64Hi) << 32) | sum64Lo; 00165 00166 return sum64; 00167 } 00168 #endif 00169 00170 #else 00171 #error No assembly defined. See valid options in assembly.h 00172 #endif /** RDA5991H */ 00173 00174 #endif /* _ASSEMBLY_H */
Generated on Tue Jul 12 2022 16:28:52 by
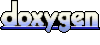