
This is a port of the mruby/c tutorial Chapter 03 to the mbed environment.
Embed:
(wiki syntax)
Show/hide line numbers
vm.h
Go to the documentation of this file.
00001 /*! @file 00002 @brief 00003 mruby bytecode executor. 00004 00005 <pre> 00006 Copyright (C) 2015 Kyushu Institute of Technology. 00007 Copyright (C) 2015 Shimane IT Open-Innovation Center. 00008 00009 This file is distributed under BSD 3-Clause License. 00010 00011 Fetch mruby VM bytecodes, decode and execute. 00012 00013 </pre> 00014 */ 00015 00016 #ifndef MRBC_SRC_VM_H_ 00017 #define MRBC_SRC_VM_H_ 00018 00019 #include <stdint.h> 00020 #include "value.h " 00021 #include "vm_config.h" 00022 00023 #ifdef __cplusplus 00024 extern "C" { 00025 #endif 00026 00027 00028 //================================================================ 00029 /*!@brief 00030 00031 */ 00032 typedef struct IREP { 00033 int16_t unused; //! unused flag 00034 struct IREP *next; //! irep linked list 00035 00036 uint8_t *code; 00037 mrb_object *ptr_to_pool; 00038 uint8_t *ptr_to_sym; 00039 00040 int16_t nlocals; 00041 int16_t nregs; 00042 int16_t rlen; 00043 int32_t ilen; 00044 00045 int16_t iseq; 00046 00047 } mrb_irep; 00048 00049 00050 //================================================================ 00051 /*!@brief 00052 00053 */ 00054 typedef struct CALLINFO { 00055 mrb_irep *pc_irep; 00056 uint32_t pc; 00057 uint32_t reg_top; 00058 uint8_t n_args; // num of args 00059 } mrb_callinfo; 00060 00061 00062 //================================================================ 00063 /*!@brief 00064 00065 */ 00066 typedef struct VM { 00067 mrb_irep *irep; // irep linked list 00068 00069 uint8_t vm_id; // vm_id : 1..n 00070 int16_t priority; // 00071 const uint8_t *mrb; // bytecode 00072 00073 mrb_irep *pc_irep; // PC 00074 int16_t pc; // PC 00075 00076 int reg_top; 00077 mrb_value regs[MAX_REGS_SIZE]; 00078 int callinfo_top; 00079 mrb_callinfo callinfo[MAX_CALLINFO_SIZE]; 00080 00081 mrb_class *target_class; 00082 mrb_object *top_self; // ? 00083 00084 int32_t error_code; 00085 00086 volatile int8_t flag_preemption; 00087 } mrb_vm; 00088 00089 00090 00091 mrb_irep *new_irep(mrb_vm *vm); 00092 struct VM *vm_open(void); 00093 void vm_close(struct VM *vm); 00094 void vm_boot(struct VM *vm); 00095 int vm_run(struct VM *vm); 00096 00097 00098 //================================================================ 00099 /*!@brief 00100 Get 32bit value from memory big endian. 00101 00102 @param s Pointer of memory. 00103 @return 32bit unsigned value. 00104 */ 00105 inline static uint32_t bin_to_uint32( const void *s ) 00106 { 00107 uint8_t *s1 = (uint8_t *)s; 00108 uint32_t ret; 00109 00110 ret = *s1++; 00111 ret = (ret << 8) + *s1++; 00112 ret = (ret << 8) + *s1++; 00113 ret = (ret << 8) + *s1; 00114 return ret; 00115 } 00116 00117 00118 //================================================================ 00119 /*!@brief 00120 Get 16bit value from memory big endian. 00121 00122 @param s Pointer of memory. 00123 @return 16bit unsigned value. 00124 */ 00125 inline static uint16_t bin_to_uint16( const void *s ) 00126 { 00127 uint8_t *s1 = (uint8_t *)s; 00128 uint16_t ret; 00129 00130 ret = *s1++; 00131 ret = (ret << 8) + *s1; 00132 return ret; 00133 } 00134 00135 00136 #ifdef __cplusplus 00137 } 00138 #endif 00139 #endif 00140
Generated on Tue Jul 12 2022 23:36:30 by
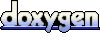