
This is a port of the mruby/c tutorial Chapter 03 to the mbed environment.
Embed:
(wiki syntax)
Show/hide line numbers
static.c
Go to the documentation of this file.
00001 /*! @file 00002 @brief 00003 Declare static data. 00004 00005 <pre> 00006 Copyright (C) 2015-2016 Kyushu Institute of Technology. 00007 Copyright (C) 2015-2016 Shimane IT Open-Innovation Center. 00008 00009 This file is distributed under BSD 3-Clause License. 00010 </pre> 00011 */ 00012 00013 #include "static.h" 00014 #include "vm_config.h" 00015 #include "class.h " 00016 #include "symbol.h" 00017 00018 /* Static Variables */ 00019 /* VM contains regs, stack, PC, and so on */ 00020 mrb_vm mrbc_vm[MAX_VM_COUNT]; 00021 00022 //static mrb_object mrbc_object[MAX_OBJECT_COUNT]; 00023 //mrb_object *mrbc_pool_object; 00024 00025 mrb_constobject mrbc_const[MAX_CONST_COUNT]; 00026 00027 mrb_globalobject mrbc_global[MAX_GLOBAL_OBJECT_SIZE]; 00028 00029 /* Class Tree */ 00030 mrb_class *mrbc_class_object; 00031 00032 /* Classes */ 00033 mrb_class *mrbc_class_false; 00034 mrb_class *mrbc_class_true; 00035 mrb_class *mrbc_class_nil; 00036 mrb_class *mrbc_class_array; 00037 mrb_class *mrbc_class_fixnum; 00038 mrb_class *mrbc_class_symbol; 00039 #if MRBC_USE_FLOAT 00040 mrb_class *mrbc_class_float; 00041 #endif 00042 #if MRBC_USE_STRING 00043 mrb_class *mrbc_class_string; 00044 #endif 00045 mrb_class *mrbc_class_range; 00046 mrb_class *mrbc_class_hash; 00047 00048 void init_static(void) 00049 { 00050 int i; 00051 00052 for( i=0 ; i<MAX_VM_COUNT ; i++ ){ 00053 mrbc_vm[i].vm_id = i+1; 00054 mrbc_vm[i].priority = -1; 00055 } 00056 00057 /* global objects */ 00058 for( i=0 ; i<MAX_GLOBAL_OBJECT_SIZE ; i++ ){ 00059 mrbc_global[i].sym_id = -1; 00060 } 00061 00062 for( i=0 ; i<MAX_CONST_COUNT; i++ ){ 00063 mrbc_const[i].sym_id = -1; 00064 } 00065 00066 /* init class */ 00067 mrbc_init_class(); 00068 } 00069
Generated on Tue Jul 12 2022 23:36:30 by
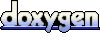