
This is a port of the mruby/c tutorial Chapter 03 to the mbed environment.
Embed:
(wiki syntax)
Show/hide line numbers
opcode.h
Go to the documentation of this file.
00001 /*! @file 00002 @brief 00003 00004 00005 <pre> 00006 Copyright (C) 2015 Kyushu Institute of Technology. 00007 Copyright (C) 2015 Shimane IT Open-Innovation Center. 00008 00009 This file is distributed under BSD 3-Clause License. 00010 00011 00012 </pre> 00013 */ 00014 00015 #ifndef MRBC_SRC_OPCODE_H_ 00016 #define MRBC_SRC_OPCODE_H_ 00017 00018 #ifdef __cplusplus 00019 extern "C" { 00020 #endif 00021 00022 00023 #define GET_OPCODE(code) ((code) & 0x7f) 00024 #define GETARG_A(code) (((code) >> 23) & 0x1ff) 00025 #define GETARG_B(code) (((code) >> 14) & 0x1ff) 00026 #define GETARG_C(code) (((code) >> 7) & 0x7f) 00027 #define GETARG_Ax(code) (((code) >> 7) & 0x1ffffff) 00028 00029 #define GETARG_b(code) GETARG_UNPACK_b(code,14,2) 00030 00031 #define GETARG_UNPACK_b(i,n1,n2) ((((code)) >> (7+(n2))) & (((1<<(n1))-1))) 00032 00033 00034 #define MAXARG_Bx (0xffff) 00035 #define MAXARG_sBx (MAXARG_Bx>>1) 00036 #define GETARG_Bx(code) (((code) >> 7) & 0xffff) 00037 #define GETARG_sBx(code) (GETARG_Bx(code)-MAXARG_sBx) 00038 #define GETARG_C(code) (((code) >> 7) & 0x7f) 00039 00040 00041 //================================================================ 00042 /*!@brief 00043 00044 */ 00045 enum OPCODE { 00046 OP_NOP = 0x00, 00047 OP_MOVE = 0x01, 00048 OP_LOADL = 0x02, 00049 OP_LOADI = 0x03, 00050 OP_LOADSYM = 0x04, 00051 OP_LOADNIL = 0x05, 00052 OP_LOADSELF = 0x06, 00053 OP_LOADT = 0x07, 00054 OP_LOADF = 0x08, 00055 OP_GETGLOBAL = 0x09, 00056 OP_SETGLOBAL = 0x0a, 00057 00058 OP_GETCONST = 0x11, 00059 OP_SETCONST = 0x12, 00060 00061 OP_JMP = 0x17, 00062 OP_JMPIF = 0x18, 00063 OP_JMPNOT = 0x19, 00064 OP_SEND = 0x20, 00065 00066 OP_ENTER = 0x26, 00067 00068 OP_RETURN = 0x29, 00069 00070 OP_ADD = 0x2c, 00071 OP_ADDI = 0x2d, 00072 OP_SUB = 0x2e, 00073 OP_SUBI = 0x2f, 00074 OP_MUL = 0x30, 00075 OP_DIV = 0x31, 00076 OP_EQ = 0x32, 00077 OP_LT = 0x33, 00078 OP_LE = 0x34, 00079 OP_GT = 0x35, 00080 OP_GE = 0x36, 00081 OP_ARRAY = 0x37, 00082 00083 OP_STRING = 0x3d, 00084 00085 OP_HASH = 0x3f, 00086 OP_LAMBDA = 0x40, 00087 OP_RANGE = 0x41, 00088 00089 OP_CLASS = 0x43, 00090 00091 OP_METHOD = 0x46, 00092 00093 OP_TCLASS = 0x48, 00094 00095 OP_STOP = 0x4a, 00096 }; 00097 00098 #ifdef __cplusplus 00099 } 00100 #endif 00101 #endif 00102
Generated on Tue Jul 12 2022 23:36:30 by
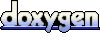