
haris semic haris spahic
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "N5110.h" 00003 #include "math.h" 00004 00005 #define MAXWIDTH 84 00006 #define MAXHEIGHT 48 00007 00008 Timer deb; 00009 DigitalOut enable(dp14); 00010 InterruptIn taster(dp1); 00011 int X=0;int Y=0; 00012 int xpoc=90,ypoc=90,x,y; 00013 AnalogIn VRx(dp11); 00014 AnalogIn VRy(dp10); 00015 DigitalIn SW(dp9); 00016 DigitalIn mytaster (dp1); 00017 00018 N5110 lcd (dp4, dp24, dp23, dp25, dp2, dp6, dp18); 00019 00020 void setCursor (int x, int y) 00021 { 00022 for (int i=x; i<=x+2; i++) 00023 { 00024 for (int j=y; j<=y+2; j++) 00025 { 00026 lcd.setPixel(i, j); 00027 lcd.refresh(); 00028 } 00029 } 00030 } 00031 00032 void obrisi (int x, int y) 00033 { 00034 for (int i=x; i<=x+2; i++) 00035 { 00036 for (int j=y; j<=y+2; j++) 00037 { 00038 lcd.clearPixel(i, j); 00039 lcd.refresh(); 00040 } 00041 } 00042 } 00043 00044 void pomjeriDesno (int &x, int &y) 00045 { 00046 if (x>75) 00047 { 00048 obrisi(x, y); 00049 x=0; 00050 00051 setCursor(x, y); 00052 } 00053 else { 00054 obrisi(x, y); 00055 x=x+1; 00056 setCursor(x, y); 00057 } 00058 } 00059 00060 void pomjeriLijevo (int &x, int &y) 00061 { 00062 if (x<5) 00063 { 00064 obrisi(x, y); 00065 x=75; 00066 setCursor(x, y); 00067 } 00068 else { 00069 obrisi(x, y); 00070 x=x-1; 00071 setCursor(x, y); 00072 } 00073 } 00074 00075 void pomjeriGore (int &x, int &y) 00076 { 00077 if (y>43) 00078 { 00079 obrisi(x, y); 00080 y=0; 00081 setCursor(x, y); 00082 } 00083 else { 00084 obrisi(x, y); 00085 y=y+1; 00086 setCursor(x, y); 00087 } 00088 } 00089 00090 void pomjeriDole (int &x, int &y) 00091 { 00092 if (y<5) 00093 { 00094 obrisi(x, y); 00095 y=75; 00096 setCursor(x, y); 00097 } 00098 else { 00099 obrisi(x, y); 00100 y=y-1; 00101 setCursor(x, y); 00102 } 00103 } 00104 00105 void iscrtajLiniju(int x1, int y1, int x2, int y2) 00106 { 00107 lcd.setXYAddress(0,0); 00108 int dx = abs(x2-x1), sx = x1<x2 ? 1 : -1; 00109 int dy = abs(y2-y1), sy = y1<y2 ? 1 : -1; 00110 int err = (dx>dy ? dx : -dy)/2, e2; 00111 x1=x2; y1=y2; 00112 for(;;){ 00113 setCursor(x1,y1); 00114 if (x1==x2 && y1==y2) break; 00115 e2 = err; 00116 if (e2 >-dx) { err -= dy; x1 += sx; } 00117 if (e2 < dy) { err += dx; y1 += sy; } 00118 } 00119 } 00120 00121 static int nesto =1; 00122 void tacka() 00123 { 00124 00125 if(deb.read_ms() > 400) 00126 if(nesto%2!=0) {xpoc=X; ypoc=Y;nesto++;} 00127 else{ 00128 x=X; y=Y; 00129 iscrtajLiniju(xpoc,ypoc,x,y); 00130 wait(5); 00131 lcd.clear(); 00132 xpoc=90; ypoc=90; lcd.setXYAddress(0,0); X=0; Y=0; nesto=1; 00133 } 00134 00135 deb.reset(); 00136 } 00137 00138 00139 00140 00141 00142 00143 00144 int main() 00145 { 00146 00147 enable=1; 00148 00149 SW.mode(PullUp); 00150 deb.start(); 00151 00152 // inicijalizacija displeja 00153 lcd.init(); 00154 lcd.setXYAddress(X,Y); 00155 00156 int x1=-1, y1=-1, x2, y2; 00157 00158 setCursor(X,Y); 00159 while (1) 00160 { 00161 if(VRx < 1.0/3.0) pomjeriDesno(X,Y); 00162 else if(VRx > 2.0/3.0) pomjeriLijevo(X,Y); 00163 00164 if(VRy < 1.0/3.0) pomjeriGore(X,Y); 00165 else if(VRy > 2.0/3.0) pomjeriDole(X,Y); 00166 00167 setCursor(xpoc,ypoc); 00168 if (mytaster) 00169 { 00170 if (x1==-1 && y1==-1) 00171 { 00172 x1=X, y1=Y; 00173 lcd.setXYAddress(X,Y); 00174 lcd.setPixel(x1, y1); 00175 } 00176 else 00177 { 00178 x2=X, y2=Y; 00179 lcd.setXYAddress(X,Y); 00180 lcd.setPixel(x2, y2); 00181 } 00182 } 00183 00184 } 00185 00186 } 00187
Generated on Tue Jul 19 2022 04:55:46 by
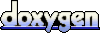