Opencv 3.1 project on GR-PEACH board
Fork of gr-peach-opencv-project by
ippasync.hpp
00001 /*M/////////////////////////////////////////////////////////////////////////////////////// 00002 // 00003 // IMPORTANT: READ BEFORE DOWNLOADING, COPYING, INSTALLING OR USING. 00004 // 00005 // By downloading, copying, installing or using the software you agree to this license. 00006 // If you do not agree to this license, do not download, install, 00007 // copy or use the software. 00008 // 00009 // 00010 // License Agreement 00011 // For Open Source Computer Vision Library 00012 // 00013 // Copyright (C) 2000-2015, Intel Corporation, all rights reserved. 00014 // Copyright (C) 2009, Willow Garage Inc., all rights reserved. 00015 // Copyright (C) 2013, OpenCV Foundation, all rights reserved. 00016 // Copyright (C) 2015, Itseez Inc., all rights reserved. 00017 // Third party copyrights are property of their respective owners. 00018 // 00019 // Redistribution and use in source and binary forms, with or without modification, 00020 // are permitted provided that the following conditions are met: 00021 // 00022 // * Redistribution's of source code must retain the above copyright notice, 00023 // this list of conditions and the following disclaimer. 00024 // 00025 // * Redistribution's in binary form must reproduce the above copyright notice, 00026 // this list of conditions and the following disclaimer in the documentation 00027 // and/or other materials provided with the distribution. 00028 // 00029 // * The name of the copyright holders may not be used to endorse or promote products 00030 // derived from this software without specific prior written permission. 00031 // 00032 // This software is provided by the copyright holders and contributors "as is" and 00033 // any express or implied warranties, including, but not limited to, the implied 00034 // warranties of merchantability and fitness for a particular purpose are disclaimed. 00035 // In no event shall the Intel Corporation or contributors be liable for any direct, 00036 // indirect, incidental, special, exemplary, or consequential damages 00037 // (including, but not limited to, procurement of substitute goods or services; 00038 // loss of use, data, or profits; or business interruption) however caused 00039 // and on any theory of liability, whether in contract, strict liability, 00040 // or tort (including negligence or otherwise) arising in any way out of 00041 // the use of this software, even if advised of the possibility of such damage. 00042 // 00043 //M*/ 00044 00045 #ifndef __OPENCV_CORE_IPPASYNC_HPP__ 00046 #define __OPENCV_CORE_IPPASYNC_HPP__ 00047 00048 #ifdef HAVE_IPP_A 00049 00050 #include "opencv2/core.hpp" 00051 #include <ipp_async_op.h> 00052 #include <ipp_async_accel.h> 00053 00054 namespace cv 00055 { 00056 00057 namespace hpp 00058 { 00059 00060 /** @addtogroup core_ipp 00061 This section describes conversion between OpenCV and [Intel® IPP Asynchronous 00062 C/C++](http://software.intel.com/en-us/intel-ipp-preview) library. [Getting Started 00063 Guide](http://registrationcenter.intel.com/irc_nas/3727/ipp_async_get_started.htm) help you to 00064 install the library, configure header and library build paths. 00065 */ 00066 //! @{ 00067 00068 //! convert OpenCV data type to hppDataType 00069 inline int toHppType(const int cvType) 00070 { 00071 int depth = CV_MAT_DEPTH(cvType); 00072 int hppType = depth == CV_8U ? HPP_DATA_TYPE_8U : 00073 depth == CV_16U ? HPP_DATA_TYPE_16U : 00074 depth == CV_16S ? HPP_DATA_TYPE_16S : 00075 depth == CV_32S ? HPP_DATA_TYPE_32S : 00076 depth == CV_32F ? HPP_DATA_TYPE_32F : 00077 depth == CV_64F ? HPP_DATA_TYPE_64F : -1; 00078 CV_Assert( hppType >= 0 ); 00079 return hppType; 00080 } 00081 00082 //! convert hppDataType to OpenCV data type 00083 inline int toCvType(const int hppType) 00084 { 00085 int cvType = hppType == HPP_DATA_TYPE_8U ? CV_8U : 00086 hppType == HPP_DATA_TYPE_16U ? CV_16U : 00087 hppType == HPP_DATA_TYPE_16S ? CV_16S : 00088 hppType == HPP_DATA_TYPE_32S ? CV_32S : 00089 hppType == HPP_DATA_TYPE_32F ? CV_32F : 00090 hppType == HPP_DATA_TYPE_64F ? CV_64F : -1; 00091 CV_Assert( cvType >= 0 ); 00092 return cvType; 00093 } 00094 00095 /** @brief Convert hppiMatrix to Mat. 00096 00097 This function allocates and initializes new matrix (if needed) that has the same size and type as 00098 input matrix. Supports CV_8U, CV_16U, CV_16S, CV_32S, CV_32F, CV_64F. 00099 @param src input hppiMatrix. 00100 @param dst output matrix. 00101 @param accel accelerator instance (see hpp::getHpp for the list of acceleration framework types). 00102 @param cn number of channels. 00103 */ 00104 inline void copyHppToMat(hppiMatrix* src, Mat& dst, hppAccel accel, int cn) 00105 { 00106 hppDataType type; 00107 hpp32u width, height; 00108 hppStatus sts; 00109 00110 if (src == NULL) 00111 return dst.release(); 00112 00113 sts = hppiInquireMatrix(src, &type, &width, &height); 00114 00115 CV_Assert( sts == HPP_STATUS_NO_ERROR); 00116 00117 int matType = CV_MAKETYPE(toCvType(type), cn); 00118 00119 CV_Assert(width%cn == 0); 00120 00121 width /= cn; 00122 00123 dst.create((int)height, (int)width, (int)matType); 00124 00125 size_t newSize = (size_t)(height*(hpp32u)(dst.step)); 00126 00127 sts = hppiGetMatrixData(accel,src,(hpp32u)(dst.step),dst.data,&newSize); 00128 00129 CV_Assert( sts == HPP_STATUS_NO_ERROR); 00130 } 00131 00132 /** @brief Create Mat from hppiMatrix. 00133 00134 This function allocates and initializes the Mat that has the same size and type as input matrix. 00135 Supports CV_8U, CV_16U, CV_16S, CV_32S, CV_32F, CV_64F. 00136 @param src input hppiMatrix. 00137 @param accel accelerator instance (see hpp::getHpp for the list of acceleration framework types). 00138 @param cn number of channels. 00139 @sa howToUseIPPAconversion, hpp::copyHppToMat, hpp::getHpp. 00140 */ 00141 inline Mat getMat(hppiMatrix* src, hppAccel accel, int cn) 00142 { 00143 Mat dst; 00144 copyHppToMat(src, dst, accel, cn); 00145 return dst; 00146 } 00147 00148 /** @brief Create hppiMatrix from Mat. 00149 00150 This function allocates and initializes the hppiMatrix that has the same size and type as input 00151 matrix, returns the hppiMatrix*. 00152 00153 If you want to use zero-copy for GPU you should to have 4KB aligned matrix data. See details 00154 [hppiCreateSharedMatrix](http://software.intel.com/ru-ru/node/501697). 00155 00156 Supports CV_8U, CV_16U, CV_16S, CV_32S, CV_32F, CV_64F. 00157 00158 @note The hppiMatrix pointer to the image buffer in system memory refers to the src.data. Control 00159 the lifetime of the matrix and don't change its data, if there is no special need. 00160 @param src input matrix. 00161 @param accel accelerator instance. Supports type: 00162 - **HPP_ACCEL_TYPE_CPU** - accelerated by optimized CPU instructions. 00163 - **HPP_ACCEL_TYPE_GPU** - accelerated by GPU programmable units or fixed-function 00164 accelerators. 00165 - **HPP_ACCEL_TYPE_ANY** - any acceleration or no acceleration available. 00166 @sa howToUseIPPAconversion, hpp::getMat 00167 */ 00168 inline hppiMatrix* getHpp(const Mat& src, hppAccel accel) 00169 { 00170 int htype = toHppType(src.type()); 00171 int cn = src.channels(); 00172 00173 CV_Assert(src.data); 00174 hppAccelType accelType = hppQueryAccelType(accel); 00175 00176 if (accelType!=HPP_ACCEL_TYPE_CPU) 00177 { 00178 hpp32u pitch, size; 00179 hppQueryMatrixAllocParams(accel, src.cols*cn, src.rows, htype, &pitch, &size); 00180 if (pitch!=0 && size!=0) 00181 if ((int)(src.data)%4096==0 && pitch==(hpp32u)(src.step)) 00182 { 00183 return hppiCreateSharedMatrix(htype, src.cols*cn, src.rows, src.data, pitch, size); 00184 } 00185 } 00186 00187 return hppiCreateMatrix(htype, src.cols*cn, src.rows, src.data, (hpp32s)(src.step));; 00188 } 00189 00190 //! @} 00191 }} 00192 00193 #endif 00194 00195 #endif 00196
Generated on Tue Jul 12 2022 15:17:26 by
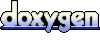