
simple data logger writing to internal RAM. No existing over-written. search for next file count
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "TextLCD.h" 00003 00004 //#include "MAX6662.h" 00005 // Create the local filesystem under the name "local" 00006 00007 LocalFileSystem local("local"); 00008 TextLCD lcd(p24, p25, p26, p27, p28, p29, p30); // rs, rw, e, d0, d1, d2, d3 00009 DigitalOut led1(LED1); 00010 DigitalOut led2(LED2); 00011 DigitalOut led3(LED3); 00012 DigitalIn button(p12); 00013 00014 AnalogIn grx(p16); // gyroscope x-axis 00015 AnalogIn gry(p17); // gyroscope y-axis 00016 AnalogIn acx(p18); // accelerometer x-axis 00017 AnalogIn acy(p19); // accelerometer y-axis 00018 AnalogIn acz(p20); // accelerometer z-axis 00019 00020 00021 00022 char direct[]= "local"; 00023 char file[20]; 00024 char next_file[20]; 00025 int file_no; 00026 int count; 00027 bool not_found; 00028 bool check; 00029 00030 //********************************************* 00031 //sub for filename search 00032 bool exists(char * root, char *filename) { 00033 00034 DIR *d; 00035 struct dirent *p; 00036 d = opendir("/local"); 00037 00038 //printf("\nList of files in the directory %s:\n", root); 00039 bool found = false; 00040 if ( d != NULL ) { 00041 while ( !found && (p = readdir(d)) != NULL ) { 00042 //printf(" - %s\n", p->d_name); 00043 if ( strcmp(p->d_name, filename) == 0 ) 00044 found = true; 00045 } 00046 } 00047 closedir(d); 00048 not_found=!found; 00049 return found; 00050 } 00051 00052 //********************************************* 00053 00054 // this routine could check the last number of file it is 00055 // last file _no = file_no - 1; 00056 00057 //not_found=false; // intial 00058 00059 00060 //********************************************* 00061 //sub to search for filename 00062 int search( void ) { 00063 00064 not_found=false; 00065 led1=button; 00066 file_no=1; 00067 count=0; 00068 00069 while (not_found==false) { 00070 sprintf(file, "TEMP%d.CSV", file_no); 00071 // wait(.1); 00072 if ( exists(direct, file) ) { 00073 00074 // lcd.cls(); 00075 //lcd.locate(0,0); 00076 //lcd.printf("Found %s",file); 00077 } else { 00078 00079 lcd.cls(); 00080 //lcd.locate(0,0); 00081 lcd.printf("Not %s", file); 00082 } 00083 file_no++; 00084 // lcd.locate(0,1); 00085 //lcd.printf("%d", file_no-1); 00086 } 00087 check=1; 00088 led2=check; 00089 return file_no; 00090 } 00091 00092 //*************************************************** 00093 00094 //************************************************** 00095 //sub to delay input button 00096 //avoid bouncing effect 00097 00098 int button_delay(bool b) { 00099 bool c = false; 00100 if (b==true) { 00101 wait(.5); 00102 c=true; 00103 } else { 00104 c=false; 00105 } 00106 return c; 00107 } 00108 00109 //******************************************************* 00110 //main program start here 00111 00112 bool sw; 00113 int no; 00114 00115 int main() { 00116 00117 while (1) { 00118 00119 00120 sw=button_delay(button); 00121 led1=sw; 00122 00123 if (sw == false and no == 0) { 00124 led3=1; 00125 no=search(); 00126 lcd.locate(0,1); 00127 lcd.printf("it is %d", no-1); 00128 sprintf(next_file, "/local/temp%d.csv", file_no-1); 00129 } 00130 00131 00132 if (sw == true and no != 0) { 00133 no=0; 00134 led3=0; 00135 00136 FILE *fp = fopen(next_file, "w"); 00137 //while (button) { 00138 while (button_delay(button)) { 00139 led2 = !led2; 00140 // time,data<cr> 00141 fprintf(fp,"%.2f,%.2f,%.2f,%.2f,%.2f\n",grx.read()*3.3, gry.read()*3.3,acx.read()*3.3,acy.read()*3.3,acz.read()*3.3); 00142 //pc.printf("%f,%f,%f\n",acx.read(),acy.read(),acz.read()); 00143 lcd.locate(0,0); 00144 lcd.printf("gx=%.2f,gy=%.2f",grx.read()*3.3, gry.read()*3.3); 00145 //lcd.printf("%.2f", f); 00146 lcd.locate(0,1); 00147 lcd.printf("ax=%.2f,ay=%.2f,az=%.2f",acx.read()*3.3,acy.read()*3.3,acz.read()*3.3); 00148 wait (.01); 00149 } 00150 fclose(fp); 00151 // lcd.cls(); 00152 //lcd.locate(0,0); 00153 ///lcd.printf("Press button to start"); 00154 // wait(.1); 00155 } 00156 } 00157 } 00158 00159 00160
Generated on Sun Jul 17 2022 03:52:56 by
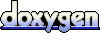