
ITEAD 2.4E LCD Interface 8 Bit Parallel Mode
Embed:
(wiki syntax)
Show/hide line numbers
lcd_driver.cpp
00001 #include "lcd_driver.h" 00002 00003 00004 #define LCD_DRIVER_C 00005 00006 #include "mbed.h" 00007 00008 //lsb .. msb 00009 BusOut LCD_DataBus(p13,p14,p15,p16,p17,p18,p19,p20); 00010 00011 00012 DigitalOut RS(p21); 00013 DigitalOut WR(p22); 00014 DigitalOut RD(p23); 00015 DigitalOut CS(p24); 00016 DigitalOut RST(p25); 00017 00018 00019 void Write_Command(unsigned int c) 00020 { 00021 RS = 0; 00022 Write(c); 00023 } 00024 00025 void Write_Data(unsigned int c) 00026 { 00027 RS = 1; 00028 Write(c); 00029 } 00030 00031 void Write(unsigned int c) 00032 { 00033 LCD_DataBus = c >> 8; 00034 WR = 0; 00035 WR = 1; 00036 00037 LCD_DataBus = c; 00038 WR = 0; 00039 WR = 1; 00040 00041 } 00042 00043 void Write_Command_Data(unsigned int cmd,unsigned int dat) 00044 { 00045 Write_Command(cmd); 00046 Write_Data(dat); 00047 } 00048 00049 00050 void Lcd_Init() 00051 { 00052 00053 digitalWrite(RD,HIGH); 00054 digitalWrite(CS,HIGH); 00055 digitalWrite(WR,HIGH); 00056 00057 digitalWrite(RST,HIGH); 00058 delay(1); 00059 digitalWrite(RST,LOW); 00060 delay(10); 00061 delay(1); 00062 digitalWrite(RST,HIGH); 00063 00064 CS = 0; 00065 00066 Write_Command_Data(0x0011,0x2004); 00067 Write_Command_Data(0x0013,0xCC00); 00068 Write_Command_Data(0x0015,0x2600); 00069 Write_Command_Data(0x0014,0x252A); 00070 // Write_Command_Data(0x14,0x002A); 00071 Write_Command_Data(0x0012,0x0033); 00072 Write_Command_Data(0x0013,0xCC04); 00073 //delayms(1); 00074 Write_Command_Data(0x0013,0xCC06); 00075 //delayms(1); 00076 Write_Command_Data(0x0013,0xCC4F); 00077 //delayms(1); 00078 Write_Command_Data(0x0013,0x674F); 00079 Write_Command_Data(0x0011,0x2003); 00080 //delayms(1); 00081 Write_Command_Data(0x0030,0x2609); 00082 Write_Command_Data(0x0031,0x242C); 00083 Write_Command_Data(0x0032,0x1F23); 00084 Write_Command_Data(0x0033,0x2425); 00085 Write_Command_Data(0x0034,0x2226); 00086 Write_Command_Data(0x0035,0x2523); 00087 Write_Command_Data(0x0036,0x1C1A); 00088 Write_Command_Data(0x0037,0x131D); 00089 Write_Command_Data(0x0038,0x0B11); 00090 Write_Command_Data(0x0039,0x1210); 00091 Write_Command_Data(0x003A,0x1315); 00092 Write_Command_Data(0x003B,0x3619); 00093 Write_Command_Data(0x003C,0x0D00); 00094 Write_Command_Data(0x003D,0x000D); 00095 Write_Command_Data(0x0016,0x0007); 00096 Write_Command_Data(0x0002,0x0013); 00097 Write_Command_Data(0x0003,0x0003); 00098 Write_Command_Data(0x0001,0x0127); 00099 //delayms(1); 00100 Write_Command_Data(0x0008,0x0303); 00101 Write_Command_Data(0x000A,0x000B); 00102 Write_Command_Data(0x000B,0x0003); 00103 Write_Command_Data(0x000C,0x0000); 00104 Write_Command_Data(0x0041,0x0000); 00105 Write_Command_Data(0x0050,0x0000); 00106 Write_Command_Data(0x0060,0x0005); 00107 Write_Command_Data(0x0070,0x000B); 00108 Write_Command_Data(0x0071,0x0000); 00109 Write_Command_Data(0x0078,0x0000); 00110 Write_Command_Data(0x007A,0x0000); 00111 Write_Command_Data(0x0079,0x0007); 00112 Write_Command_Data(0x0007,0x0051); 00113 //delayms(1); 00114 Write_Command_Data(0x0007,0x0053); 00115 Write_Command_Data(0x0079,0x0000); 00116 00117 Write_Command(0x0022); 00118 00119 00120 } 00121 00122 void SetXY(unsigned int x0,unsigned int x1,unsigned int y0,unsigned int y1) 00123 { 00124 Write_Command_Data(0x0046,(x1 << 8)| x0); 00125 //Write_Command_Data(0x0047,x1); 00126 Write_Command_Data(0x0047,y1); 00127 Write_Command_Data(0x0048,y0); 00128 Write_Command_Data(0x0020,x0); 00129 Write_Command_Data(0x0021,y0); 00130 Write_Command (0x0022);//LCD_WriteCMD(GRAMWR); 00131 } 00132 void Pant(unsigned int color) 00133 { 00134 int i,j; 00135 int color_test; 00136 color_test = 0; 00137 SetXY(0,239,0,319); 00138 //SetXY(0,120,0,120); 00139 00140 for(i=0;i<320;i++) 00141 { 00142 for (j=0;j<240;j++) 00143 { 00144 Write_Data(color_test); 00145 color_test+=10; 00146 } 00147 00148 } 00149 } 00150 void LCD_clear() 00151 { 00152 unsigned int i,j; 00153 SetXY(0,239,0,319); 00154 for(i=0;i<X_CONST;i++) 00155 { 00156 for(j=0;j<Y_CONST;j++) 00157 { 00158 Write_Data(0x0000); 00159 } 00160 } 00161 } 00162 00163 00164 #undef LCD_DRIVER_C
Generated on Mon Jul 18 2022 08:44:23 by
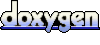