
A security system that is activated after the object is removed from the box.
Dependencies: 4DGL-uLCD-SE Camera_LS_Y201 FatFileSystem SDFileSystem mbed wave_player
main.cpp
00001 #include "mbed.h" 00002 #include "Camera_LS_Y201.h" 00003 #include "SDFileSystem.h" 00004 #include "wave_player.h" 00005 #include "uLCD_4DGL.h" 00006 #include <stdio.h> 00007 00008 #define DEBMSG printf 00009 #define DEBUG printf("Bug me") 00010 #define NEWLINE() printf("\r\n") 00011 00012 #define USE_SDCARD 0 00013 00014 00015 #define FILENAME "/sd/IMG_%04d.jpg" 00016 SDFileSystem fs(p5, p6, p7, p8, "sd"); 00017 00018 //#define FILENAME "/local/IMG_%04d.jpg" 00019 //LocalFileSystem fs("local"); 00020 00021 Camera_LS_Y201 cam1(p13, p14); 00022 00023 AnalogIn sensor(p19); 00024 DigitalOut led(LED1); 00025 uLCD_4DGL uLCD(p28,p27,p11); // serial tx, serial rx, reset pin; 00026 AnalogOut DACout(p18); 00027 wave_player waver(&DACout); 00028 Serial pc(USBTX, USBRX); 00029 Serial device(p9,p10); 00030 00031 typedef struct work { 00032 FILE *fp; 00033 } work_t; 00034 00035 work_t work; 00036 00037 void regularSub() { 00038 00039 } 00040 void microInterrupt() { 00041 int strikes = 0; 00042 char rchar=0; 00043 //wake up device - needs more work and a timeout 00044 device.putc('b'); 00045 while (device.getc()!='o') { 00046 DEBMSG("First While loop."); 00047 NEWLINE(); 00048 device.putc('b'); 00049 wait(0.2); 00050 } 00051 while (strikes<=3) { 00052 DEBMSG("Second While loop."); 00053 NEWLINE(); 00054 device.putc('i'); //Start Recognition 00055 device.putc('B'); //Use Wordset 3 - the numbers 1..10 00056 //Use built-in speaker independent numbers (0..10) and listen for a number 00057 while (device.readable()!=0) {DEBMSG("Third While loop."); 00058 NEWLINE();} 00059 if (device.getc()=='s') { 00060 device.putc(' '); 00061 rchar=device.getc(); 00062 if (rchar=='G') {DEBMSG("Correct Command."); 00063 //strikes--; 00064 NEWLINE(); 00065 uLCD.text_width(2); //4X size text 00066 uLCD.text_height(2); 00067 uLCD.cls(); 00068 uLCD.color(GREEN); 00069 uLCD.printf("Correct Command"); 00070 wait(5); 00071 uLCD.cls(); 00072 wait(2); 00073 uLCD.color(RED); 00074 uLCD.text_width(3); //4X size text 00075 uLCD.text_height(3); 00076 uLCD.printf("Alarm is turn off"); //Default Green on black text 00077 strikes = 4; 00078 } 00079 else { 00080 strikes++; 00081 DEBMSG("Wrong Command."); 00082 uLCD.cls(); 00083 uLCD.text_width(2); //4X size text 00084 uLCD.text_height(2); 00085 uLCD.color(RED); 00086 uLCD.printf("Wrong Command"); 00087 wait(5); 00088 uLCD.cls(); 00089 NEWLINE(); 00090 while(strikes==4){ 00091 DEBMSG("buzzing While loop."); 00092 NEWLINE(); 00093 FILE *wave_file; 00094 wave_file=fopen("/sd/buzzer2.wav","r"); 00095 waver.play(wave_file); 00096 fclose(wave_file); 00097 } 00098 } 00099 00100 } 00101 } 00102 } 00103 /** 00104 * Callback function for readJpegFileContent. 00105 * 00106 * @param buf A pointer to a buffer. 00107 * @param siz A size of the buffer. 00108 */ 00109 void callback_func(int done, int total, uint8_t *buf, size_t siz) { 00110 fwrite(buf, siz, 1, work.fp); 00111 00112 static int n = 0; 00113 int tmp = done * 100 / total; 00114 if (n != tmp) { 00115 n = tmp; 00116 DEBMSG("Writing...: %3d%%", n); 00117 NEWLINE(); 00118 } 00119 } 00120 00121 /** 00122 * Capture. 00123 * 00124 * @param cam A pointer to a camera object. 00125 * @param filename The file name. 00126 * 00127 * @return Return 0 if it succeed. 00128 */ 00129 int capture(Camera_LS_Y201 *cam, char *filename) { 00130 /* 00131 * Take a picture. 00132 */ 00133 if (cam->takePicture() != 0) { 00134 return -1; 00135 } 00136 DEBMSG("Captured."); 00137 NEWLINE(); 00138 00139 /* 00140 * Open file. 00141 */ 00142 work.fp = fopen(filename, "wb"); 00143 if (work.fp == NULL) { 00144 return -2; 00145 } 00146 00147 /* 00148 * Read the content. 00149 */ 00150 DEBMSG("%s", filename); 00151 NEWLINE(); 00152 if (cam->readJpegFileContent(callback_func) != 0) { 00153 fclose(work.fp); 00154 return -3; 00155 } 00156 fclose(work.fp); 00157 00158 /* 00159 * Stop taking pictures. 00160 */ 00161 cam->stopTakingPictures(); 00162 00163 return 0; 00164 } 00165 00166 /** 00167 * Entry point. 00168 */ 00169 int main(void) { 00170 while(1){ 00171 DEBMSG("Checking again"); 00172 NEWLINE(); 00173 if(sensor > 0.3) { 00174 //pc.printf("The distance is %d\n",sensor); 00175 uLCD.cls(); 00176 wait(2); 00177 uLCD.color(RED); 00178 uLCD.text_width(3); //4X size text 00179 uLCD.text_height(3); 00180 uLCD.printf("Alarm is turn off"); //Default Green on black text 00181 } else { 00182 uLCD.cls(); 00183 uLCD.color(GREEN); 00184 uLCD.text_width(3); //4X size text 00185 uLCD.text_height(3); 00186 uLCD.printf("Alarm is turn on"); 00187 uLCD.cls(); 00188 uLCD.printf("A picture is taken now"); 00189 wait(3); 00190 uLCD.cls(); 00191 uLCD.printf("Alarm is turn on"); 00192 DEBMSG("Camera module"); 00193 NEWLINE(); 00194 DEBMSG("Resetting..."); 00195 NEWLINE(); 00196 wait(1); 00197 00198 if (cam1.reset() == 0) { 00199 DEBMSG("Reset OK."); 00200 NEWLINE(); 00201 } else { 00202 DEBMSG("Reset fail."); 00203 NEWLINE(); 00204 error("Reset fail."); 00205 } 00206 wait(1); 00207 00208 int cnt = 0; 00209 char fname[64]; 00210 snprintf(fname, sizeof(fname) - 1, FILENAME, cnt); 00211 int r = capture(&cam1, fname); 00212 if (r == 0) { 00213 DEBMSG("[%04d]:OK.", cnt); 00214 NEWLINE(); 00215 } else { 00216 DEBMSG("[%04d]:NG. (code=%d)", cnt, r); 00217 NEWLINE(); 00218 error("Failure."); 00219 } 00220 cnt++; 00221 00222 microInterrupt(); 00223 } 00224 } 00225 00226 }
Generated on Sun Jul 24 2022 03:26:14 by
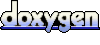