
Just testing things, based off of http-example-wnc
Dependencies: easy-connect mbed-http
Fork of http-example-wnc by
main-http.cpp
00001 #include "select-demo.h" 00002 00003 #if DEMO == DEMO_HTTP 00004 00005 #include "mbed.h" 00006 #include "easy-connect.h" 00007 #include "http_request.h" 00008 00009 Serial pc(USBTX, USBRX); 00010 00011 void dump_response(HttpResponse* res) { 00012 printf("Status: %d - %s\n", res->get_status_code(), res->get_status_message().c_str()); 00013 00014 printf("Headers:\n"); 00015 for (size_t ix = 0; ix < res->get_headers_length(); ix++) { 00016 printf("\t%s: %s\n", res->get_headers_fields()[ix]->c_str(), res->get_headers_values()[ix]->c_str()); 00017 } 00018 printf("\nBody (%d bytes):\n\n%s\n", res->get_body_length(), res->get_body_as_string().c_str()); 00019 } 00020 00021 int main() { 00022 pc.baud(115200); 00023 // Connect to the network (see mbed_app.json for the connectivity method used) 00024 while(1) { 00025 printf("Hello?\n"); 00026 } 00027 NetworkInterface *network = easy_connect(true); 00028 if (!network) { 00029 printf("Cannot connect to the network, see serial output"); 00030 return 1; 00031 } 00032 00033 printf("See if it gives any debug info"); 00034 00035 // Do a GET request to httpbin.org 00036 { 00037 // By default the body is automatically parsed and stored in a buffer, this is memory heavy. 00038 // To receive chunked response, pass in a callback as last parameter to the constructor. 00039 HttpRequest* get_req = new HttpRequest(network, HTTP_GET, "http://httpbin.org/status/418"); 00040 00041 HttpResponse* get_res = get_req->send(); 00042 if (!get_res) { 00043 printf("HttpRequest failed (error code %d)\n", get_req->get_error()); 00044 return 1; 00045 } 00046 00047 printf("\n----- HTTP GET response -----\n"); 00048 dump_response(get_res); 00049 00050 delete get_req; 00051 } 00052 00053 // POST request to httpbin.org 00054 { 00055 HttpRequest* post_req = new HttpRequest(network, HTTP_POST, "http://httpbin.org/post"); 00056 post_req->set_header("Content-Type", "application/json"); 00057 00058 const char body[] = "{\"hello\":\"world\"}"; 00059 00060 HttpResponse* post_res = post_req->send(body, strlen(body)); 00061 if (!post_res) { 00062 printf("HttpRequest failed (error code %d)\n", post_req->get_error()); 00063 return 1; 00064 } 00065 00066 printf("\n----- HTTP POST response -----\n"); 00067 dump_response(post_res); 00068 00069 delete post_req; 00070 } 00071 00072 Thread::wait(osWaitForever); 00073 } 00074 00075 #endif
Generated on Thu Jul 14 2022 09:37:19 by
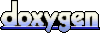