Revised Embedded Artists' MTK3339 library to save all raw GPS messages and enable optional decoding of RMC message.
Fork of MTK3339 by
MTK3339.h
00001 // Header to represent serial interface to MTK3339 GPS chip 00002 // Original author: Embedded Artists 00003 // Revised by T. Bronez, 2015-07-31 00004 00005 #ifndef MTK3339_H 00006 #define MTK3339_H 00007 #include <time.h> 00008 00009 /** 00010 * An interface to the MTK3339 GPS module. 00011 */ 00012 class MTK3339 { 00013 public: 00014 00015 enum NmeaSentence { 00016 NMEA_NONE = 0x00, 00017 NMEA_GGA = 0x01, 00018 NMEA_GSA = 0x02, 00019 NMEA_GSV = 0x04, 00020 NMEA_RMC = 0x08, 00021 NMEA_VTG = 0x10 00022 }; 00023 00024 struct RmcType { 00025 char status; // A valid, V invalid, blank unknown 00026 double lat_dd; // latitude in decimal degrees 00027 double lon_dd; // longitude in decimal degrees 00028 struct tm gps_tm; // date and time (to seconds) 00029 int msec; // millseconds 00030 }; 00031 00032 /** 00033 * Create an interface to the MTK3339 GPS module 00034 * 00035 * @param tx UART TX line pin 00036 * @param rx UART RX line pin 00037 */ 00038 MTK3339(PinName tx, PinName rx); 00039 00040 /** 00041 * Start to read data from the GPS module. 00042 * 00043 * @param fptr A pointer to a void function that will be called when there 00044 * is data available. 00045 * @param mask specifies which sentence types (NmeaSentence) that are of 00046 * interest. The callback function will only be called for messages 00047 * specified in this mask. 00048 */ 00049 void start(void (*fptr)(void), int mask); 00050 00051 /** 00052 * Start to read data from the GPS module. 00053 * 00054 * @param tptr pointer to the object to call the member function on 00055 * @param mptr pointer to the member function to be called 00056 * @param mask specifies which sentence types (NmeaSentence) that are of 00057 * interest. The member function will only be called for messages 00058 * specified in this mask. 00059 */ 00060 template<typename T> 00061 void start(T* tptr, void (T::*mptr)(void), int mask) { 00062 if((mptr != NULL) && (tptr != NULL) && mask) { 00063 _dataCallback.attach(tptr, mptr); 00064 _sentenceMask = mask; 00065 _serial.attach(this, &MTK3339::uartIrq, Serial::RxIrq); 00066 } 00067 } 00068 00069 /** 00070 * Stop to read data from GPS module 00071 */ 00072 void stop(); 00073 00074 /** 00075 * Get the type of the data reported in available data callback. 00076 * This method will only return a valid type when called within the 00077 * callback. 00078 */ 00079 NmeaSentence getAvailableDataType(); 00080 00081 /** 00082 * Time, position and date related data 00083 */ 00084 enum PubConstants { 00085 MSG_BUF_SZ = 100 00086 }; 00087 // Leave room for a terminating \0 in each buffer 00088 char ggaMsg[MSG_BUF_SZ+1]; // longest observed strlen = 72 00089 char gsaMsg[MSG_BUF_SZ+1]; // longest observed strlen = 56 00090 char gsvMsg[MSG_BUF_SZ+1]; // longest observed strlen = 68 00091 char rmcMsg[MSG_BUF_SZ+1]; // longest observed strlen = 70 00092 char vtgMsg[MSG_BUF_SZ+1]; // longest observed strlen = 37 00093 00094 RmcType rmc; 00095 void decodeRMC (); 00096 00097 private: 00098 00099 enum PrivConstants { 00100 MTK3339_BUF_SZ = 255 00101 }; 00102 00103 enum DataState { 00104 StateStart = 0, 00105 StateData 00106 }; 00107 00108 FunctionPointer _dataCallback; 00109 char _buf[MTK3339_BUF_SZ]; 00110 int _bufPos; 00111 DataState _state; 00112 int _sentenceMask; 00113 NmeaSentence _availDataType; 00114 Serial _serial; 00115 00116 void saveRMC(char* data, int dataLen); 00117 void saveGGA(char* data, int dataLen); 00118 void saveGSA(char* data, int dataLen); 00119 void saveGSV(char* data, int dataLen); 00120 void saveVTG(char* data, int dataLen); 00121 void saveData(char* data, int len); 00122 void uartIrq(); 00123 00124 }; 00125 00126 #endif 00127
Generated on Fri Jul 22 2022 20:10:17 by
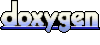