
lwIP Robustness Test.
Dependencies: EthernetInterface mbed-rtos mbed
main.cpp
00001 #include "mbed.h" 00002 #include "EthernetInterface.h" 00003 00004 #define msgdbg(...) 00005 00006 //#define msgdbg printf 00007 00008 00009 #define SENDING_RETRIES 5u 00010 #define READING_RETRIES 5u 00011 #define DHCP_RETRIES 10u 00012 #define BUFFER_SIZE 256 00013 00014 #define NUMBER_OF_HOSTS 3u 00015 00016 #define MBED_HOST "www.mbed.org" 00017 #define MBED_HOST_REQUEST "GET /media/uploads/mbed_official/hello.txt HTTP/1.0\n\n" 00018 00019 #define MEDIAFAX_HOST "www.mediafax.ro" 00020 #define MEDIAFAX_HOST_REQUEST "GET / HTTP/1.1\nHost: www.mediafax.ro\n\n" 00021 00022 #define NXP_HOST "www.nxp.com" 00023 #define NXP_HOST_REQUEST "GET /documents/user_manual/UM10360.pdf HTTP/1.0\nHost: www.nxp.com\n\n" 00024 00025 int totalTime = 0; 00026 extern uint32_t os_time; 00027 00028 struct WebStats 00029 { 00030 char request[70]; 00031 char host[20]; 00032 int avgDuration; 00033 int maxReceived; 00034 int minReceived; 00035 int snapshots; 00036 }; 00037 00038 struct WebStats webStatistics[NUMBER_OF_HOSTS]= 00039 { 00040 {MBED_HOST_REQUEST,MBED_HOST,0,0,0x7FFFFFFF,0}, 00041 {MEDIAFAX_HOST_REQUEST,MEDIAFAX_HOST,0,0,0x7FFFFFFF,0}, 00042 {NXP_HOST_REQUEST,NXP_HOST,0,0,0x7FFFFFFF,0} 00043 }; 00044 00045 void printStatistics(void) 00046 { 00047 printf("\nTotal Time : %f seconds\n",(float)totalTime/1000.0f); 00048 for(int i=0;i<NUMBER_OF_HOSTS;i++) 00049 { 00050 printf("Host number : %d | %s\n",i, webStatistics[i].host); 00051 printf("MaxRecv : %d\n",webStatistics[i].maxReceived); 00052 printf("MinRecv : %d\n",webStatistics[i].minReceived); 00053 printf("Avg duration : %d ms\n",webStatistics[i].avgDuration); 00054 printf("Total snapshots : %d\n\n",webStatistics[i].snapshots); 00055 } 00056 00057 } 00058 00059 int main() { 00060 00061 int retries = 0; 00062 EthernetInterface eth; 00063 TCPSocketConnection client; 00064 00065 printf("Initialising...\n"); 00066 00067 // use DHCP 00068 eth.init(); 00069 00070 // attempt DHCP and if timing out then try again 00071 while (eth.connect()) { 00072 retries++; 00073 printf("[%d] DHCP timeout %d\n",os_time,retries); 00074 if(retries >= DHCP_RETRIES) 00075 { 00076 printf("DHCP failed. Bailing out..\n"); 00077 goto failure; 00078 } 00079 }; 00080 00081 printf("[%d] Starting the robustness test...\n",os_time); 00082 00083 while(1) 00084 { 00085 for(int i=0;i<NUMBER_OF_HOSTS;i++,client.close()) 00086 { 00087 int retries = 0; 00088 int received = 0; 00089 int time = 0; 00090 char tmpBuffer[BUFFER_SIZE]; 00091 int ret; 00092 00093 printf("Mbed --> %s\n",webStatistics[i].host); 00094 00095 time = os_time; 00096 // connecting 00097 if (client.connect(webStatistics[i].host, 80)) { 00098 printf("Failed to connect to : %s\n",webStatistics[i].host); 00099 continue; 00100 } 00101 00102 retries = 0; 00103 00104 // sending request 00105 while(1) 00106 { 00107 if( (ret = client.send_all(webStatistics[i].request,strlen(webStatistics[i].request))) <= 0) 00108 { 00109 printf("Retry sending no %d, return :%d\n",retries,ret); 00110 retries++; 00111 } 00112 else break; 00113 00114 if(retries == SENDING_RETRIES+1) 00115 { 00116 printf("Failed sending. Bailing out this connection\n"); 00117 continue; 00118 } 00119 } 00120 retries = 0; 00121 00122 // start reading 00123 while(true) 00124 { 00125 if( (ret = client.receive(tmpBuffer,sizeof(tmpBuffer))) <= 0) 00126 retries++; 00127 else 00128 received += ret; 00129 00130 if(retries == READING_RETRIES) 00131 break; 00132 } 00133 00134 //Snapshot! 00135 time = os_time - time; 00136 00137 if(webStatistics[i].maxReceived < received) 00138 { 00139 webStatistics[i].maxReceived = received; 00140 } 00141 00142 if(webStatistics[i].minReceived > received) 00143 { 00144 webStatistics[i].minReceived = received; 00145 } 00146 printf("Received : %d bytes\n",received); 00147 webStatistics[i].avgDuration = ((webStatistics[i].avgDuration *(webStatistics[i].snapshots++) ) + time)/webStatistics[i].snapshots; 00148 totalTime += time; 00149 } 00150 00151 printStatistics(); 00152 Thread::wait(500); 00153 } 00154 failure: 00155 printf("Fatal error. Main thread is inactive\n"); 00156 while(1); 00157 }
Generated on Thu Jul 21 2022 07:53:08 by
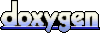