
A PicoTCP demo app testing the ethernet throughput on the lpc1768 mbed board.
Dependencies: PicoTCP lpc1768-picotcp-eth mbed-rtos mbed picotcp-demo-printf
main.cpp
00001 /****************************************************************************** 00002 PicoTCP. Copyright (c) 2012-2013 TASS Belgium NV. Some rights reserved. 00003 See LICENSE and COPYING for usage. https://github.com/tass-belgium/picotcp 00004 00005 This library is free software; you can redistribute it and/or 00006 modify it under the terms of the GNU General Public License Version 2 00007 as published by the Free Software Foundation; 00008 00009 Authors: Maxime Vincent 00010 00011 Purpose: This is a PicoTCP demo. It will try to connect a TCP socket at 00012 from 192.168.100.1 port 6666 to 192.168.100.2 port 4404 00013 and then send "TCPSIZ" a-characters there. 00014 When that succeeded, it prints the throughput on the serial output. 00015 00016 Libraries needed to get this up and running: 00017 PicoTCP, 00018 lpc1768-picotcp-demo-eth, 00019 picotcp-demo-printf, 00020 mbed-rtos, 00021 mbed 00022 00023 ******************************************************************************/ 00024 00025 #include "mbed.h" 00026 00027 extern "C" { 00028 // PicoTCP includes 00029 #include "pico_dev_mbed_emac.h" 00030 #include "pico_stack.h" 00031 #include "pico_ipv4.h" 00032 #include "pico_socket.h" 00033 // CMSIS OS includes 00034 #include "cmsis_os.h" 00035 // other includes 00036 #include "serial_api.h" 00037 #include <stdio.h> 00038 #include "printf.h" 00039 } 00040 00041 // Send 10 MBs 00042 #define TCPSIZ (10 * 1024 * 1024) 00043 00044 serial_t _serial; 00045 00046 DigitalOut myled1(LED1); /* Tick */ 00047 00048 void app_tcpbench(void); 00049 00050 void _putc(void *p, char ch) 00051 { 00052 serial_putc(&_serial, ch); 00053 } 00054 00055 int main() { 00056 struct pico_device *my_dev; 00057 struct pico_ip4 myaddr, mynetmask; 00058 00059 // init serial output 00060 serial_init(&_serial, p9, p10); 00061 init_printf(NULL,_putc); 00062 mprintf(">>> Welcome 2 PicoTCP <<<\r\n"); 00063 00064 // init PicoTCP stack 00065 pico_stack_init(); 00066 00067 pico_string_to_ipv4("192.168.100.1", &myaddr.addr); 00068 pico_string_to_ipv4("255.255.255.0", &mynetmask.addr); 00069 my_dev = pico_emac_create("MBED-EMAC"); 00070 00071 pico_ipv4_link_add(my_dev, myaddr, mynetmask); 00072 00073 mprintf("ip=192.168.100.1 - will connect to 192.168.100.2:4404 in 5 seconds...\r\n"); 00074 osDelay(5000); 00075 mprintf("PicoTCP Benchmark starting...\r\n"); 00076 app_tcpbench(); 00077 00078 // This is the IDLE task 00079 while(1) { 00080 static uint8_t led_tick; 00081 pico_stack_tick(); 00082 //wait_ms(1); // wait a millisec... 00083 00084 led_tick++; 00085 if (!(led_tick%200)) 00086 { 00087 myled1 = !myled1; 00088 led_tick = 0; 00089 } 00090 } 00091 } 00092 00093 /*** START TCP BENCH ***/ 00094 00095 #define TCP_BENCH_TX 1 00096 #define TCP_BENCH_RX 2 00097 00098 uint8_t buffer0[2000] __attribute__((section("AHBSRAM0"))); // To save some RAM 00099 00100 int tcpbench_mode = TCP_BENCH_TX; 00101 struct pico_socket *tcpbench_sock = NULL; 00102 static unsigned long tcpbench_time_start,tcpbench_time_end; 00103 00104 void cb_tcpbench(uint16_t ev, struct pico_socket *s) 00105 { 00106 static int closed = 0; 00107 static unsigned long count = 0; 00108 00109 static long tcpbench_wr_size = 0; 00110 long tcpbench_w = 0; 00111 double tcpbench_time = 0; 00112 unsigned int time_ms; 00113 00114 count++; 00115 00116 if (ev & PICO_SOCK_EV_RD) { 00117 } 00118 00119 if (ev & PICO_SOCK_EV_CONN) { 00120 if (tcpbench_mode == TCP_BENCH_TX) 00121 mprintf("tb> Connection established with server.\r\n"); 00122 } 00123 00124 if (ev & PICO_SOCK_EV_FIN) { 00125 mprintf("tb> Socket closed. Exit normally. \r\n"); 00126 } 00127 00128 if (ev & PICO_SOCK_EV_ERR) { 00129 mprintf("tb> Socket Error received: %s. Bailing out.\r\n", strerror(pico_err)); 00130 } 00131 00132 if (ev & PICO_SOCK_EV_CLOSE) { 00133 mprintf("tb> event close\r\n"); 00134 pico_socket_close(s); 00135 return; 00136 } 00137 00138 if (ev & PICO_SOCK_EV_WR) { 00139 00140 if (tcpbench_wr_size < TCPSIZ && tcpbench_mode == TCP_BENCH_TX) { 00141 do { 00142 tcpbench_w = pico_socket_write(tcpbench_sock, buffer0, TCPSIZ-tcpbench_wr_size); 00143 if (tcpbench_w > 0) { 00144 tcpbench_wr_size += tcpbench_w; 00145 //mprintf("Written : %d \n",tcpbench_wr_size); 00146 //mprintf("tb> SOCKET WRITTEN - %d\r\n",tcpbench_w); 00147 } 00148 if (tcpbench_w < 0) { 00149 mprintf("tb> Socket Error received: %s. Bailing out.\r\n", strerror(pico_err)); 00150 exit(5); 00151 } 00152 if (tcpbench_time_start == 0) 00153 tcpbench_time_start = PICO_TIME_MS(); 00154 } while(tcpbench_w > 0); 00155 //mprintf("tcpbench_wr_size = %d \r\n", tcpbench_wr_size); 00156 } else { 00157 if (!closed && tcpbench_mode == TCP_BENCH_TX) { 00158 tcpbench_time_end = PICO_TIME_MS(); 00159 pico_socket_shutdown(s, PICO_SHUT_WR); 00160 mprintf("tb> TCPSIZ written\r\n"); 00161 mprintf("tb> Called shutdown()\r\n"); 00162 tcpbench_time = (tcpbench_time_end - tcpbench_time_start)/1000.0; /* get number of seconds */ 00163 time_ms = (unsigned int)(tcpbench_time * 1000); 00164 mprintf("tb> Transmitted %u bytes in %u milliseconds\r\n",TCPSIZ, time_ms); 00165 mprintf("tb> average write throughput %u kbit/sec\r\n",( (TCPSIZ/time_ms) * 8) ); 00166 closed = 1; 00167 } 00168 } 00169 } 00170 } 00171 00172 void app_tcpbench(void) 00173 { 00174 struct pico_socket *s; 00175 uint16_t port_be = short_be(4404); 00176 uint16_t local_port; 00177 struct pico_ip4 server_addr; 00178 struct pico_ip4 local_addr; 00179 memset(buffer0,'a',2000); 00180 tcpbench_mode = TCP_BENCH_TX; 00181 00182 mprintf("Opening socket...\r\n"); 00183 s = pico_socket_open(PICO_PROTO_IPV4, PICO_PROTO_TCP, &cb_tcpbench); 00184 if (!s) 00185 { 00186 mprintf("Socket was not opened...\r\n"); 00187 exit(1); 00188 } 00189 00190 local_port = short_be(6666); 00191 pico_string_to_ipv4("192.168.100.1", &local_addr.addr); 00192 pico_string_to_ipv4("192.168.100.2", &server_addr.addr); 00193 pico_socket_bind(s, &local_addr, &local_port); 00194 00195 tcpbench_sock = s; 00196 pico_socket_connect(s, &server_addr, port_be); 00197 00198 return; 00199 }
Generated on Wed Jul 13 2022 20:47:50 by
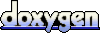