
Pour Mike le faible
Dependencies: BLE_API BMP085 mbed nRF51822
Fork of nRF51822_SimpleControls by
main.cpp
00001 /* 00002 00003 Copyright (c) 2012-2014 RedBearLab 00004 00005 Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00006 and associated documentation files (the "Software"), to deal in the Software without restriction, 00007 including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, 00009 subject to the following conditions: 00010 The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. 00011 00012 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, 00013 INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR 00014 PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE 00015 FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00016 ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 00018 */ 00019 00020 #include "mbed.h" 00021 #include "ble/BLE.h" 00022 #include "Servo.h" 00023 #include "GattCallbackParamTypes.h" 00024 #include "BMP085.h" 00025 00026 #define BLE_UUID_TXRX_SERVICE 0x0000 /**< The UUID of the Nordic UART Service. */ 00027 #define BLE_UUID_TX_CHARACTERISTIC 0x0002 /**< The UUID of the TX Characteristic. */ 00028 #define BLE_UUIDS_RX_CHARACTERISTIC 0x0003 /**< The UUID of the RX Characteristic. */ 00029 00030 #define TXRX_BUF_LEN 20 00031 00032 #define DIGITAL_OUT_PIN P0_17 //D7 00033 #define DIGITAL_IN_PIN P0_5 //A4 00034 #define PWM_PIN P0_16 //D6 00035 #define SERVO_PIN P0_14 //D10 00036 #define ANALOG_IN_PIN P0_6 //A5 00037 00038 BLE ble; 00039 00040 DigitalOut LED_SET(DIGITAL_OUT_PIN); 00041 DigitalIn BUTTON(DIGITAL_IN_PIN); 00042 PwmOut PWM(PWM_PIN); 00043 AnalogIn ANALOG(ANALOG_IN_PIN); 00044 Servo MYSERVO(SERVO_PIN); 00045 00046 Serial pc(USBTX, USBRX); 00047 00048 static uint8_t analog_enabled = 0; 00049 static uint8_t old_state = 0; 00050 00051 // The Nordic UART Service 00052 static const uint8_t uart_base_uuid[] = {0x71, 0x3D, 0, 0, 0x50, 0x3E, 0x4C, 0x75, 0xBA, 0x94, 0x31, 0x48, 0xF1, 0x8D, 0x94, 0x1E}; 00053 static const uint8_t uart_tx_uuid[] = {0x71, 0x3D, 0, 3, 0x50, 0x3E, 0x4C, 0x75, 0xBA, 0x94, 0x31, 0x48, 0xF1, 0x8D, 0x94, 0x1E}; 00054 static const uint8_t uart_rx_uuid[] = {0x71, 0x3D, 0, 2, 0x50, 0x3E, 0x4C, 0x75, 0xBA, 0x94, 0x31, 0x48, 0xF1, 0x8D, 0x94, 0x1E}; 00055 static const uint8_t uart_base_uuid_rev[] = {0x1E, 0x94, 0x8D, 0xF1, 0x48, 0x31, 0x94, 0xBA, 0x75, 0x4C, 0x3E, 0x50, 0, 0, 0x3D, 0x71}; 00056 00057 00058 uint8_t txPayload[TXRX_BUF_LEN] = {0,}; 00059 uint8_t rxPayload[TXRX_BUF_LEN] = {0,}; 00060 00061 //static uint8_t rx_buf[TXRX_BUF_LEN]; 00062 //static uint8_t rx_len=0; 00063 00064 00065 GattCharacteristic txCharacteristic (uart_tx_uuid, txPayload, 1, TXRX_BUF_LEN, GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_WRITE | GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_WRITE_WITHOUT_RESPONSE); 00066 00067 GattCharacteristic rxCharacteristic (uart_rx_uuid, rxPayload, 1, TXRX_BUF_LEN, GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_NOTIFY); 00068 00069 GattCharacteristic *uartChars[] = {&txCharacteristic, &rxCharacteristic}; 00070 00071 GattService uartService(uart_base_uuid, uartChars, sizeof(uartChars) / sizeof(GattCharacteristic *)); 00072 00073 00074 00075 void disconnectionCallback(Gap::Handle_t handle, Gap::DisconnectionReason_t reason) 00076 { 00077 pc.printf("Disconnected \r\n"); 00078 pc.printf("Restart advertising \r\n"); 00079 ble.startAdvertising(); 00080 } 00081 00082 void WrittenHandler(const GattWriteCallbackParams *Handler) 00083 { 00084 uint8_t buf[TXRX_BUF_LEN]; 00085 uint16_t bytesRead, index; 00086 00087 00088 if (Handler->handle == txCharacteristic.getValueAttribute().getHandle()) 00089 { 00090 ble.readCharacteristicValue(txCharacteristic.getValueAttribute().getHandle(), buf, &bytesRead); 00091 memset(txPayload, 0, TXRX_BUF_LEN); 00092 memcpy(txPayload, buf, TXRX_BUF_LEN); 00093 00094 for(index=0; index<bytesRead; index++) 00095 pc.putc(buf[index]); 00096 00097 if(buf[0] == 0x01) 00098 { 00099 if(buf[1] == 0x01){ 00100 while(buf[1] == 0x01){ 00101 // on relit ce qui est envoyé pour être sur qu'on veut toujours faire clignoter la LED VIOLETTE 00102 ble.readCharacteristicValue(txCharacteristic.getValueAttribute().getHandle(), buf, &bytesRead); 00103 memset(txPayload, 0, TXRX_BUF_LEN); 00104 memcpy(txPayload, buf, TXRX_BUF_LEN); 00105 00106 for(index=0; index<bytesRead; index++) 00107 pc.putc(buf[index]); 00108 // Pour ne pas refaire clignoter alors que l'ordre a été donnée d'arreter 00109 if(buf[1] == 0x01){ 00110 LED_SET = 1; 00111 wait_ms(100); 00112 LED_SET = 0; 00113 wait_ms(100); 00114 } 00115 else; 00116 } 00117 } 00118 else 00119 LED_SET = 0; 00120 } 00121 else if(buf[0] == 0xA0) 00122 { 00123 if(buf[1] == 0x01) 00124 analog_enabled = 1; 00125 else 00126 analog_enabled = 0; 00127 } 00128 else if(buf[0] == 0x02) 00129 { 00130 float value = (float)buf[1]/255; 00131 PWM = value; 00132 } 00133 else if(buf[0] == 0x03) 00134 { 00135 MYSERVO.write(buf[1]); 00136 } 00137 else if(buf[0] == 0x04) 00138 { 00139 analog_enabled = 0; 00140 PWM = 0; 00141 MYSERVO.write(0); 00142 LED_SET = 0; 00143 old_state = 0; 00144 } 00145 00146 } 00147 } 00148 /* 00149 void uartCB(void) 00150 { 00151 while(pc.readable()) 00152 { 00153 rx_buf[rx_len++] = pc.getc(); 00154 if(rx_len>=20 || rx_buf[rx_len-1]=='\0' || rx_buf[rx_len-1]=='\n') 00155 { 00156 ble.updateCharacteristicValue(rxCharacteristic.getValueAttribute().getHandle(), rx_buf, rx_len); 00157 pc.printf("RecHandler \r\n"); 00158 pc.printf("Length: "); 00159 pc.putc(rx_len); 00160 pc.printf("\r\n"); 00161 rx_len = 0; 00162 break; 00163 } 00164 } 00165 } 00166 */ 00167 void m_status_check_handle(void) 00168 { 00169 uint8_t buf[3]; 00170 if (analog_enabled) // if analog reading enabled 00171 { 00172 // Read and send out 00173 float s = ANALOG; 00174 uint16_t value = s*1024; 00175 buf[0] = (0x0B); 00176 buf[1] = (value >> 8); 00177 buf[2] = (value); 00178 ble.updateCharacteristicValue(rxCharacteristic.getValueAttribute().getHandle(), buf, 3); 00179 } 00180 00181 // If digital in changes, report the state 00182 if (BUTTON != old_state) 00183 { 00184 old_state = BUTTON; 00185 00186 if (BUTTON == 1) 00187 { 00188 buf[0] = (0x0A); 00189 buf[1] = (0x01); 00190 buf[2] = (0x00); 00191 ble.updateCharacteristicValue(rxCharacteristic.getValueAttribute().getHandle(), buf, 3); 00192 } 00193 else 00194 { 00195 buf[0] = (0x0A); 00196 buf[1] = (0x00); 00197 buf[2] = (0x00); 00198 ble.updateCharacteristicValue(rxCharacteristic.getValueAttribute().getHandle(), buf, 3); 00199 } 00200 } 00201 } 00202 00203 00204 int main(void) 00205 { 00206 BMP085 barometre(D4,D5); 00207 float temp,press; 00208 Ticker ticker; 00209 ticker.attach_us(m_status_check_handle, 200000); 00210 00211 ble.init(); 00212 ble.onDisconnection(disconnectionCallback); 00213 ble.onDataWritten(WrittenHandler); 00214 00215 pc.baud(9600); 00216 pc.printf("SimpleChat Init \r\n"); 00217 00218 //pc.attach( uartCB , pc.RxIrq); 00219 00220 // setup advertising 00221 ble.accumulateAdvertisingPayload(GapAdvertisingData::BREDR_NOT_SUPPORTED); 00222 ble.setAdvertisingType(GapAdvertisingParams::ADV_CONNECTABLE_UNDIRECTED); 00223 ble.accumulateAdvertisingPayload(GapAdvertisingData::SHORTENED_LOCAL_NAME, 00224 (const uint8_t *)"MALOU", sizeof("MALOU") - 1); 00225 ble.accumulateAdvertisingPayload(GapAdvertisingData::COMPLETE_LIST_128BIT_SERVICE_IDS, 00226 (const uint8_t *)uart_base_uuid_rev, sizeof(uart_base_uuid)); 00227 // 100ms; in multiples of 0.625ms. 00228 ble.setAdvertisingInterval(160); 00229 00230 ble.addService(uartService); 00231 00232 ble.startAdvertising(); 00233 00234 pc.printf("Advertising Start \r\n"); 00235 00236 while(1) 00237 { 00238 ble.waitForEvent(); 00239 barometre.update (); 00240 temp = barometre.get_temperature(); 00241 press = barometre.get_pressure() ; 00242 printf("Temperature = %f C et Pression = %f Pa",temp,press); 00243 } 00244 } 00245 00246 00247 00248 00249 00250 00251 00252 00253 00254 00255 00256 00257 00258 00259 00260 00261 00262 00263 00264 00265 00266 00267 00268 00269 00270 00271 00272 00273 00274 00275 00276
Generated on Wed Aug 17 2022 01:40:23 by
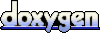