
This program is designed to work with RedBearLab BLE Controller App - Chat App. Type something from the Terminal to send to the BLEController App or vice verse. Characteristics received from App will print on Terminal. This Program scans some analog and digital inputs and save them in flash with timestamp. A RTC has been implemented. Using chat application (available for smart phones) some commands can be send to BLE Nano in order to perform some tasks. Other types of commands can be send via serial interface from a PC, especially data extraction from flash.
Dependencies: BLE_API mbed nRF51822
Fork of nRF51822_SimpleChat_VT by
myFunctions.cpp
00001 #include "myData.h" 00002 00003 00004 uint8_t eNrDaysPerMonth[12]= {31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31}; 00005 static uint8_t g_currPage = MAX_PAGE_NUM; // current page in Flash to be write 00006 00007 uint8_t flash_currPage(){ 00008 return g_currPage; 00009 } 00010 00011 uint8_t flash_go_nextPage(){ 00012 g_currPage --; 00013 if (g_currPage < MIN_PAGE_NUM) { 00014 g_currPage = MAX_PAGE_NUM; 00015 } 00016 return g_currPage; 00017 } 00018 00019 00020 uint8_t flash_prev_N_Page(uint8_t nr_of_pages){ 00021 uint8_t retVal; 00022 retVal = g_currPage + nr_of_pages; 00023 00024 if (retVal > MAX_PAGE_NUM ) { 00025 retVal = MIN_PAGE_NUM + (retVal % MAX_PAGE_NUM - 1); 00026 } 00027 return retVal; 00028 } 00029 00030 void search_latest_in_flash(mdate_time_t * outDateTime){ 00031 uint8_t page_nr, sizeB,temp[6]; 00032 uint16_t max_page=(uint16_t)MAX_PAGE_NUM+1u; 00033 int retVal; 00034 mdate_time_t max_datetime={16,1,1,0,0,0}, inv_datetime={255,255,255,255,255,255}; 00035 00036 sizeB=sizeof(mdate_time_t); 00037 uint32_t* p_curr_addr; 00038 00039 for (page_nr = MAX_PAGE_NUM; page_nr>= MIN_PAGE_NUM; page_nr --){ 00040 p_curr_addr= (uint32_t *)((uint16_t)BLE_FLASH_PAGE_SIZE * page_nr); 00041 p_curr_addr += 2; // skip the magic number and the word count 00042 00043 memcpy(temp, p_curr_addr, sizeB); 00044 retVal = memcmp(&temp, &inv_datetime, sizeB); 00045 if (retVal!=0) { 00046 retVal = memcmp(&temp, &max_datetime, sizeB); 00047 if (retVal >0) { 00048 memcpy(&max_datetime, &temp, sizeB); 00049 max_page= page_nr; 00050 } 00051 } 00052 } 00053 00054 memcpy(outDateTime, &max_datetime, sizeB); 00055 g_currPage= (uint8_t)(max_page-1u); 00056 } 00057 00058 void update_time(mdatetime_manager_t* myDateTimeVar, uint16_t tseconds){ 00059 //memcpy(&myDateTimeVar->newDateTime, &myDateTimeVar->currentDateTime, sizeof(mdate_time_t)); 00060 if (myDateTimeVar->updateDateTime ==false){ 00061 myDateTimeVar->newDateTime.seconds = (myDateTimeVar->currentDateTime.seconds + tseconds)% 60; 00062 myDateTimeVar->newDateTime.minutes = (myDateTimeVar->currentDateTime.minutes + ((tseconds + myDateTimeVar->currentDateTime.seconds) / 60))%60; 00063 if (myDateTimeVar->newDateTime.minutes< myDateTimeVar->currentDateTime.minutes ) { 00064 myDateTimeVar->currentDateTime.hours++; 00065 } 00066 myDateTimeVar->newDateTime.hours = (myDateTimeVar->currentDateTime.hours + (tseconds / 3600+myDateTimeVar->newDateTime.minutes/60))%24; 00067 if (myDateTimeVar->newDateTime.hours < myDateTimeVar->currentDateTime.hours){ 00068 myDateTimeVar->newDateTime.day = (myDateTimeVar->currentDateTime.day + 1)%(eNrDaysPerMonth[myDateTimeVar->currentDateTime.month+1]+1); 00069 if (myDateTimeVar->newDateTime.day < myDateTimeVar->currentDateTime.day ){ 00070 myDateTimeVar->newDateTime.month = (myDateTimeVar->currentDateTime.month+ 1)%13+1; 00071 if (myDateTimeVar->newDateTime.month< myDateTimeVar->currentDateTime.month){ 00072 myDateTimeVar->newDateTime.year = (myDateTimeVar->currentDateTime.year+ 1); 00073 } 00074 } 00075 } 00076 } else { 00077 myDateTimeVar->updateDateTime =false; 00078 } 00079 00080 if (myDateTimeVar->updateDateTime ==true){ // there is a new Date ? 00081 myDateTimeVar->updateDateTime =true; 00082 } 00083 memcpy(&myDateTimeVar->currentDateTime,&myDateTimeVar->newDateTime, sizeof(mdate_time_t)); 00084 } 00085 00086 00087 int buzz_int(PwmOut* buzzer, uint8_t period, uint8_t duty_cycle){ 00088 int retVal = 0; 00089 if ((duty_cycle<10)&&(period<10)){ 00090 if (period!=0) { 00091 buzzer->period_ms(period); 00092 *buzzer = (10.0 - (float)duty_cycle)/9.0; 00093 } else { 00094 *buzzer = 0; 00095 } 00096 } else { 00097 retVal=-1; 00098 } 00099 return retVal; 00100 } 00101 00102 void assert_error_app(bool condition, Serial *pc, uint16_t error, uint16_t line, const char* file){ 00103 if (condition) { 00104 pc->printf("App err = %d, line = %d, file = %s\r\n",error, line, file); 00105 } 00106 }
Generated on Tue Jul 19 2022 06:49:25 by
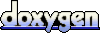