
This program is designed to work with RedBearLab BLE Controller App - Chat App. Type something from the Terminal to send to the BLEController App or vice verse. Characteristics received from App will print on Terminal. This Program scans some analog and digital inputs and save them in flash with timestamp. A RTC has been implemented. Using chat application (available for smart phones) some commands can be send to BLE Nano in order to perform some tasks. Other types of commands can be send via serial interface from a PC, especially data extraction from flash.
Dependencies: BLE_API mbed nRF51822
Fork of nRF51822_SimpleChat_VT by
myData.h
00001 #include "ble_flash.h" 00002 #include "mbed.h" 00003 00004 #define MAXBUFFER 99u //(((BLE_FLASH_PAGE_SIZE - 4 - sizeof(myData_t))/sizeof(myDataL_t)) 00005 #define MAX_WORD_PAGE_DATA 254u // to be computed as round_next_int(MAXBUFFER*sizeof(myDataL_t)+sizeof(myData_t)) 00006 00007 #define MAX_PAGE_NUM (NRF_FICR->CODESIZE - 1) // 255 00008 #define MIN_PAGE_NUM 155u 00009 00010 00011 typedef struct{ 00012 uint8_t year; // 20_XX, 2016 => 16; 00013 uint8_t month; // 1..12 00014 uint8_t day; // 1..31 00015 uint8_t hours; 00016 uint8_t minutes; 00017 uint8_t seconds; 00018 } mdate_time_t; 00019 00020 00021 typedef struct{ 00022 mdate_time_t currentDateTime; 00023 mdate_time_t newDateTime; 00024 bool updateDateTime; // true if currentTime needs to be updated with newTime; 00025 } mdatetime_manager_t; 00026 00027 00028 typedef enum { 00029 eStartAdvertising =0, 00030 eStopAdvertising =1, 00031 eDisconnect =2 00032 } connection_update_t; 00033 00034 typedef struct { 00035 uint16_t light; 00036 uint16_t gndV; 00037 uint16_t temp; 00038 bool led_on; 00039 bool led2_on; 00040 } myPayload_t; 00041 00042 00043 typedef struct { 00044 myPayload_t data; 00045 uint8_t seconds; 00046 uint8_t minutes; 00047 } myDataL_t; 00048 00049 typedef struct { 00050 mdate_time_t datetime; // 6 bytes length 00051 myPayload_t data; // 8 bytes lenth 00052 } myData_t; 00053 00054 typedef struct { 00055 myData_t startData; 00056 myDataL_t myData[MAXBUFFER]; 00057 } myDataLog_t; 00058 00059 typedef struct { 00060 myData_t startData; 00061 myDataL_t myData; 00062 } myDataLogShort_t; 00063 00064 /* Return current page to be written in Flash */ 00065 uint8_t flash_currPage(); 00066 00067 /* Return next page to be written in Flash */ 00068 uint8_t flash_go_nextPage(); 00069 00070 /* Return page-N (thas has been written in Flash) */ 00071 uint8_t flash_prev_N_Page(uint8_t nr_of_pages); 00072 00073 /*search the latest page written and extract the page_nr, date, and time*/ 00074 void search_latest_in_flash(mdate_time_t * outDateTime); 00075 00076 /* Update current date and time with tseconds and also if there is a new date/time given */ 00077 void update_time(mdatetime_manager_t* myDateTimeVar, uint16_t tseconds); 00078 00079 /* Update buzzer with period in ms =0..9 and duty cycle = 0..9*/ 00080 int buzz_int(PwmOut* buzzer, uint8_t period, uint8_t duty_cycle); 00081 00082 /* Print on serial some error from main app*/ 00083 void assert_error_app(bool condition, Serial *pc, uint16_t error, uint16_t line, const char* file);
Generated on Tue Jul 19 2022 06:49:25 by
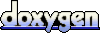