
App for BLE Nano to monitor the power consumption for a specific location, by intercepting the led flashes of a standard power meter. It counts and log the flashes for each second. It works with RedBear App for smart phone (Simple Chat App).
Dependencies: BLE_API lib_mma8451q mbed nRF51822
Fork of nRF51822_DataLogger_with_Chat by
myData.h
00001 #include "ble_flash.h" 00002 #include "mbed.h" 00003 00004 //#define MAXBUFFER 99u //(((BLE_FLASH_PAGE_SIZE - 4 - sizeof(myData_t))/sizeof(myDataL_t)) 00005 #define MAXBUFFER 251u //(((BLE_FLASH_PAGE_SIZE - 4 - sizeof(myData_t))/sizeof(myDataL_t)) 00006 //#define MAX_WORD_PAGE_DATA 254u // to be computed as round_next_int(MAXBUFFER*sizeof(myDataL_t)+sizeof(myData_t)) 00007 00008 #define MAX_PAGE_NUM (NRF_FICR->CODESIZE - 1) // 255 00009 #define MIN_PAGE_NUM 151u 00010 00011 00012 typedef struct{ 00013 uint8_t year; // 20_XX, 2016 => 16; 00014 uint8_t month; // 1..12 00015 uint8_t day; // 1..31 00016 uint8_t hours; 00017 uint8_t minutes; 00018 uint8_t seconds; 00019 } mdate_time_t; 00020 00021 00022 typedef struct{ 00023 mdate_time_t currentDateTime; 00024 mdate_time_t newDateTime; 00025 bool updateDateTime; // true if currentTime needs to be updated with newTime; 00026 } mdatetime_manager_t; 00027 00028 00029 typedef enum { 00030 eStartAdvertising =0, 00031 eStopAdvertising =1, 00032 eDisconnect =2 00033 } connection_update_t; 00034 00035 typedef struct { 00036 uint16_t hits; 00037 } myPayload_t; 00038 00039 00040 typedef struct { 00041 myPayload_t data; 00042 uint8_t seconds; 00043 uint8_t minutes; 00044 } myDataL_t; 00045 00046 typedef struct { 00047 mdate_time_t datetime; // 6 bytes length 00048 myPayload_t data; // 2 bytes lenth 00049 } myData_t; 00050 00051 typedef struct { 00052 myData_t startData; 00053 myDataL_t myData[MAXBUFFER]; 00054 } myDataLog_t; 00055 00056 typedef struct { 00057 myData_t startData; // 8 bytes 00058 myDataL_t myData; // 4 bytes 00059 } myDataLogShort_t; 00060 00061 /* Return current page to be written in Flash */ 00062 uint8_t flash_currPage(); 00063 00064 /* Return next page to be written in Flash */ 00065 uint8_t flash_go_nextPage(); 00066 00067 /* Return page-N (thas has been written in Flash) */ 00068 uint8_t flash_prev_N_Page(uint8_t nr_of_pages); 00069 00070 /*search the latest page written and extract the page_nr, date, and time*/ 00071 void search_latest_in_flash(mdate_time_t * outDateTime); 00072 00073 /* Update current date and time with tseconds and also if there is a new date/time given */ 00074 void update_time(mdatetime_manager_t* myDateTimeVar, uint16_t tseconds); 00075 00076 /* Update buzzer with period in ms =0..9 and duty cycle = 0..9*/ 00077 int buzz_int(PwmOut* buzzer, uint8_t period, uint8_t duty_cycle); 00078 00079 /* Print on serial some error from main app*/ 00080 void assert_error_app(bool condition, Serial *pc, uint16_t error, uint16_t line, const char* file);
Generated on Sat Jul 23 2022 06:55:25 by
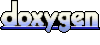