
Adafruit LED Matrix Display program
Embed:
(wiki syntax)
Show/hide line numbers
RGBmatrixPanel.h
00001 #include "mbed.h" 00002 #include "Adafruit_GFX.h" 00003 00004 #ifndef _BV 00005 #define _BV(bit) (1<<(bit)) 00006 #endif 00007 00008 #ifdef DEBUG 00009 #define log_debug(format,...) std::printf(format,##__VA_ARGS__) 00010 #else 00011 #define log_debug(...) 00012 #endif 00013 00014 //** RGBmatrixPanel is class for full color LED matrix 00015 00016 #include "mbed.h" 00017 #include "RGBmatrixPanel.h" // Hardware-specific library 00018 00019 PinName ub1=p6; 00020 PinName ug1=p7; 00021 PinName ur1=p8; 00022 PinName lb2=p9; 00023 PinName lg2=p10; 00024 PinName lr2=p11; 00025 RGBmatrixPanel matrix(ur1,ug1,ub1,lr2,lg2,lb2,p12,p13,p14,p15,p16,p17,false); 00026 //RGBmatrixPanel(r1, g1, b1, r2, g2, b2, a, b, c, sclk, latch, oe, enable double_buffer); 00027 00028 int main() 00029 { 00030 matrix.begin(); 00031 while(1) { 00032 // fill the screen with 'black' 00033 matrix.fillScreen(matrix.Color333(0, 0, 0)); 00034 // draw a pixel in solid white 00035 matrix.drawPixel(0, 0, matrix.Color333(7, 7, 7)); 00036 wait_ms(500); 00037 00038 // fix the screen with green 00039 matrix.fillRect(0, 0, 32, 16, matrix.Color333(0, 7, 0)); 00040 wait_ms(500); 00041 00042 // draw a box in yellow 00043 matrix.drawRect(0, 0, 32, 16, matrix.Color333(7, 7, 0)); 00044 wait_ms(500); 00045 00046 // draw an 'X' in red 00047 matrix.drawLine(0, 0, 31, 15, matrix.Color333(7, 0, 0)); 00048 matrix.drawLine(31, 0, 0, 15, matrix.Color333(7, 0, 0)); 00049 wait_ms(500); 00050 00051 // draw a blue circle 00052 matrix.drawCircle(7, 7, 7, matrix.Color333(0, 0, 7)); 00053 wait_ms(500); 00054 00055 // fill a violet circle 00056 matrix.fillCircle(23, 7, 7, matrix.Color333(7, 0, 7)); 00057 wait_ms(500); 00058 00059 // fill the screen with 'black' 00060 matrix.fillScreen(matrix.Color333(0, 0, 0)); 00061 00062 // draw some text! 00063 matrix.setCursor(1, 0); // start at top left, with one pixel of spacing 00064 matrix.setTextSize(1); // size 1 == 8 pixels high 00065 00066 // printff each letter with a rainbow color 00067 matrix.setTextColor(matrix.Color333(7,0,0)); 00068 matrix.putc('1'); 00069 matrix.setTextColor(matrix.Color333(7,4,0)); 00070 matrix.putc('6'); 00071 matrix.setTextColor(matrix.Color333(7,7,0)); 00072 matrix.putc('x'); 00073 matrix.setTextColor(matrix.Color333(4,7,0)); 00074 matrix.putc('3'); 00075 matrix.setTextColor(matrix.Color333(0,7,0)); 00076 matrix.putc('2'); 00077 00078 matrix.setCursor(1, 9); // next line 00079 matrix.setTextColor(matrix.Color333(0,7,7)); 00080 matrix.putc('*'); 00081 matrix.setTextColor(matrix.Color333(0,4,7)); 00082 matrix.putc('R'); 00083 matrix.setTextColor(matrix.Color333(0,0,7)); 00084 matrix.putc('G'); 00085 matrix.setTextColor(matrix.Color333(4,0,7)); 00086 matrix.putc('B'); 00087 matrix.setTextColor(matrix.Color333(7,0,4)); 00088 matrix.putc('*'); 00089 wait_ms(500); 00090 } 00091 } 00092 00093 00094 class RGBmatrixPanel : public Adafruit_GFX 00095 { 00096 00097 public: 00098 // Constructor for 16x32 panel: 00099 RGBmatrixPanel(PinName r1,PinName g1,PinName b1,PinName r2,PinName g2,PinName b2,PinName a,PinName b,PinName c,PinName sclk,PinName latch,PinName oe, bool dbuf); 00100 00101 // Constructor for 32x32 panel (adds 'd' pin): 00102 RGBmatrixPanel(PinName r1,PinName r2,PinName g1,PinName g2,PinName b1,PinName b2,PinName a,PinName b,PinName c,PinName d,PinName sclk, PinName latch,PinName oe,bool dbuf); 00103 00104 /** @fn void RGBmatrixPanel::begin(void) 00105 * @bref Attach a updateDisplay() to be called by the Ticker(every 100us) 00106 */ 00107 void begin(void); 00108 /** @fn void RGBmatrixPanel::drawPixel(int16_t x, int16_t y, uint16_t c) 00109 * @bref drawPixel 00110 */ 00111 virtual void drawPixel(int16_t x,int16_t y,uint16_t c); 00112 /** @fn void RGBmatrixPanel::fillScreen(uint16_t c) 00113 * @bref fillScreen 00114 * @param c fill screen 16bit color 0x0000 ~ 0xFFFF 00115 */ 00116 virtual void fillScreen(uint16_t c); 00117 /** @fn void RGBmatrixPanel::updateDisplay(void) 00118 * @param c updateDisplay\n 00119 * This method is called by the interrupt start at begin(). 00120 */ 00121 void updateDisplay(void); 00122 /** @fn void RGBmatrixPanel::swapBuffers(bool copy) 00123 * @param copy swap buffer (if you use double-buffer) 00124 */ 00125 void swapBuffers(bool copy); 00126 /** @fn void RGBmatrixPanel::dumpMatrix(void) 00127 * @bref dump to default USB Serial\n 00128 * Declaration is required to use.(#define DEBUG) 00129 */ 00130 void dumpMatrix(void); 00131 00132 uint8_t *backBuffer(void); 00133 00134 /** @fn uint16_t RGBmatrixPanel::Color333(uint8_t r, uint8_t g, uint8_t b) 00135 * @bref up convert to 16bit color from 9bit color. 00136 * @return 16bit(uint16_t) color value 00137 */ 00138 uint16_t Color333(uint8_t r, uint8_t g, uint8_t b); 00139 /** @fn uint16_t RGBmatrixPanel::Color444(uint8_t r, uint8_t g, uint8_t b) 00140 * @bref up convert to 16bit color from 12bit color. 00141 * @param r 0~7 00142 * @param g 0~7 00143 * @param b 0~7 00144 * @return 16bit(uint16_t) color value 00145 */ 00146 uint16_t Color444(uint8_t r, uint8_t g, uint8_t b); 00147 /** @fn uint16_t RGBmatrixPanel::Color888(uint8_t r, uint8_t g, uint8_t b) 00148 * @bref down convert to 16bit color from 24bit color. 00149 * @return 16bit(uint16_t) color value 00150 */ 00151 uint16_t Color888(uint8_t r, uint8_t g, uint8_t b); 00152 /** @fn uint16_t RGBmatrixPanel::Color888(uint8_t r, uint8_t g, uint8_t b, bool gflag) 00153 * @bref down convert to 16bit color from 24bit color using the gamma value table. 00154 * @return 16bit(uint16_t) color value 00155 */ 00156 uint16_t Color888(uint8_t r, uint8_t g, uint8_t b, bool gflag); 00157 /** @fn uint16_t RGBmatrixPanel::ColorHSV(long hue, uint8_t sat, uint8_t val, bool gflag) 00158 * @bref convert to 16bit color from (unsigned integer)HSV color using the gamma value table. 00159 * @param hue 0~1536(decimal value) 00160 * @param sat 0~255(decimal value) Does not make sense that it is not a multiple of 32. 00161 * @param val 0~255(decimal value) Does not make sense that it is not a multiple of 32. 00162 * @return 16bit(uint16_t) color value 00163 */ 00164 uint16_t ColorHSV(long hue, uint8_t sat, uint8_t val, bool gflag) 00165 ; 00166 /** @fn uint16_t RGBmatrixPanel::ColorHSV(float hue, float sat, float val, bool gflag) 00167 * @bref convert to 16bit color from (float)HSV color using the gamma value table. 00168 * @param hue Normalized value from 0.0 to 1.0 00169 * @param sat Normalized value from 0.0 to 1.0 00170 * @param val Normalized value from 0.0 to 1.0 00171 * @return 16bit(uint16_t) color value 00172 */ 00173 uint16_t ColorHSV(float hue, float sat, float val, bool gflag); 00174 private: 00175 uint8_t *matrixbuff[2]; 00176 uint8_t nRows; 00177 uint8_t backindex; 00178 bool swapflag; 00179 00180 // Init/alloc code common to both constructors: 00181 void init(uint8_t rows, bool dbuf); 00182 00183 BusOut _dataBus; 00184 BusOut _rowBus; 00185 DigitalOut _d,_sclk, _latch, _oe; 00186 Ticker _refresh; 00187 // Counters/pointers for interrupt handler: 00188 uint8_t row, plane; 00189 uint8_t *buffptr; 00190 };
Generated on Mon Aug 15 2022 09:37:08 by
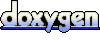