
Adafruit LED Matrix Display program
Embed:
(wiki syntax)
Show/hide line numbers
RGBmatrixPanel.cpp
00001 /* 00002 This is the core graphics library for all our displays, 00003 providing a common set of graphics primitives (points, lines, circles, etc.). 00004 It needs to be paired with a hardware-specific library for each display device we carry (to handle the lower-level functions). 00005 00006 Adafruit invests time and resources providing this open source code, 00007 please support Adafruit and open-source hardware by purchasing products from Adafruit! 00008 00009 Written by Limor Fried/Ladyada for Adafruit Industries. 00010 BSD license, check license.txt for more information. 00011 All text above must be included in any redistribution. 00012 */ 00013 00014 /* 00015 * Modified by Tatsuki Fukuda 25/5/2014 for use in mbed 00016 */ 00017 00018 #include "Adafruit_GFX.h" 00019 00020 // Draw a circle outline 00021 void Adafruit_GFX::drawCircle(int16_t x0, int16_t y0, int16_t r, 00022 uint16_t color) 00023 { 00024 int16_t f = 1 - r; 00025 int16_t ddF_x = 1; 00026 int16_t ddF_y = -2 * r; 00027 int16_t x = 0; 00028 int16_t y = r; 00029 00030 drawPixel(x0 , y0+r, color); 00031 drawPixel(x0 , y0-r, color); 00032 drawPixel(x0+r, y0 , color); 00033 drawPixel(x0-r, y0 , color); 00034 00035 while (x<y) { 00036 if (f >= 0) { 00037 y--; 00038 ddF_y += 2; 00039 f += ddF_y; 00040 } 00041 x++; 00042 ddF_x += 2; 00043 f += ddF_x; 00044 00045 drawPixel(x0 + x, y0 + y, color); 00046 drawPixel(x0 - x, y0 + y, color); 00047 drawPixel(x0 + x, y0 - y, color); 00048 drawPixel(x0 - x, y0 - y, color); 00049 drawPixel(x0 + y, y0 + x, color); 00050 drawPixel(x0 - y, y0 + x, color); 00051 drawPixel(x0 + y, y0 - x, color); 00052 drawPixel(x0 - y, y0 - x, color); 00053 } 00054 } 00055 00056 void Adafruit_GFX::drawCircleHelper( int16_t x0, int16_t y0, 00057 int16_t r, uint8_t cornername, uint16_t color) 00058 { 00059 int16_t f = 1 - r; 00060 int16_t ddF_x = 1; 00061 int16_t ddF_y = -2 * r; 00062 int16_t x = 0; 00063 int16_t y = r; 00064 00065 while (x<y) { 00066 if (f >= 0) { 00067 y--; 00068 ddF_y += 2; 00069 f += ddF_y; 00070 } 00071 x++; 00072 ddF_x += 2; 00073 f += ddF_x; 00074 if (cornername & 0x4) { 00075 drawPixel(x0 + x, y0 + y, color); 00076 drawPixel(x0 + y, y0 + x, color); 00077 } 00078 if (cornername & 0x2) { 00079 drawPixel(x0 + x, y0 - y, color); 00080 drawPixel(x0 + y, y0 - x, color); 00081 } 00082 if (cornername & 0x8) { 00083 drawPixel(x0 - y, y0 + x, color); 00084 drawPixel(x0 - x, y0 + y, color); 00085 } 00086 if (cornername & 0x1) { 00087 drawPixel(x0 - y, y0 - x, color); 00088 drawPixel(x0 - x, y0 - y, color); 00089 } 00090 } 00091 } 00092 00093 void Adafruit_GFX::fillCircle(int16_t x0, int16_t y0, int16_t r, 00094 uint16_t color) 00095 { 00096 drawFastVLine(x0, y0-r, 2*r+1, color); 00097 fillCircleHelper(x0, y0, r, 3, 0, color); 00098 } 00099 00100 // Used to do circles and roundrects 00101 void Adafruit_GFX::fillCircleHelper(int16_t x0, int16_t y0, int16_t r, 00102 uint8_t cornername, int16_t delta, uint16_t color) 00103 { 00104 00105 int16_t f = 1 - r; 00106 int16_t ddF_x = 1; 00107 int16_t ddF_y = -2 * r; 00108 int16_t x = 0; 00109 int16_t y = r; 00110 00111 while (x<y) { 00112 if (f >= 0) { 00113 y--; 00114 ddF_y += 2; 00115 f += ddF_y; 00116 } 00117 x++; 00118 ddF_x += 2; 00119 f += ddF_x; 00120 00121 if (cornername & 0x1) { 00122 drawFastVLine(x0+x, y0-y, 2*y+1+delta, color); 00123 drawFastVLine(x0+y, y0-x, 2*x+1+delta, color); 00124 } 00125 if (cornername & 0x2) { 00126 drawFastVLine(x0-x, y0-y, 2*y+1+delta, color); 00127 drawFastVLine(x0-y, y0-x, 2*x+1+delta, color); 00128 } 00129 } 00130 } 00131 00132 // Bresenham's algorithm - thx wikpedia 00133 void Adafruit_GFX::drawLine(int16_t x0, int16_t y0, 00134 int16_t x1, int16_t y1, 00135 uint16_t color) 00136 { 00137 int16_t steep = abs(y1 - y0) > abs(x1 - x0); 00138 if (steep) { 00139 swap(x0, y0); 00140 swap(x1, y1); 00141 } 00142 00143 if (x0 > x1) { 00144 swap(x0, x1); 00145 swap(y0, y1); 00146 } 00147 00148 int16_t dx, dy; 00149 dx = x1 - x0; 00150 dy = abs(y1 - y0); 00151 00152 int16_t err = dx / 2; 00153 int16_t ystep; 00154 00155 if (y0 < y1) { 00156 ystep = 1; 00157 } else { 00158 ystep = -1; 00159 } 00160 00161 for (; x0<=x1; x0++) { 00162 if (steep) { 00163 drawPixel(y0, x0, color); 00164 } else { 00165 drawPixel(x0, y0, color); 00166 } 00167 err -= dy; 00168 if (err < 0) { 00169 y0 += ystep; 00170 err += dx; 00171 } 00172 } 00173 } 00174 00175 // Draw a rectangle 00176 void Adafruit_GFX::drawRect(int16_t x, int16_t y, 00177 int16_t w, int16_t h, 00178 uint16_t color) 00179 { 00180 drawFastHLine(x, y, w, color); 00181 drawFastHLine(x, y+h-1, w, color); 00182 drawFastVLine(x, y, h, color); 00183 drawFastVLine(x+w-1, y, h, color); 00184 } 00185 00186 void Adafruit_GFX::drawFastVLine(int16_t x, int16_t y, 00187 int16_t h, uint16_t color) 00188 { 00189 // Update in subclasses if desired! 00190 drawLine(x, y, x, y+h-1, color); 00191 } 00192 00193 void Adafruit_GFX::drawFastHLine(int16_t x, int16_t y, 00194 int16_t w, uint16_t color) 00195 { 00196 // Update in subclasses if desired! 00197 drawLine(x, y, x+w-1, y, color); 00198 } 00199 00200 void Adafruit_GFX::fillRect(int16_t x, int16_t y, int16_t w, int16_t h, 00201 uint16_t color) 00202 { 00203 // Update in subclasses if desired! 00204 for (int16_t i=x; i<x+w; i++) { 00205 drawFastVLine(i, y, h, color); 00206 } 00207 } 00208 00209 void Adafruit_GFX::fillScreen(uint16_t color) 00210 { 00211 fillRect(0, 0, _width, _height, color); 00212 } 00213 00214 // Draw a rounded rectangle 00215 void Adafruit_GFX::drawRoundRect(int16_t x, int16_t y, int16_t w, 00216 int16_t h, int16_t r, uint16_t color) 00217 { 00218 // smarter version 00219 drawFastHLine(x+r , y , w-2*r, color); // Top 00220 drawFastHLine(x+r , y+h-1, w-2*r, color); // Bottom 00221 drawFastVLine(x , y+r , h-2*r, color); // Left 00222 drawFastVLine(x+w-1, y+r , h-2*r, color); // Right 00223 // draw four corners 00224 drawCircleHelper(x+r , y+r , r, 1, color); 00225 drawCircleHelper(x+w-r-1, y+r , r, 2, color); 00226 drawCircleHelper(x+w-r-1, y+h-r-1, r, 4, color); 00227 drawCircleHelper(x+r , y+h-r-1, r, 8, color); 00228 } 00229 00230 // Fill a rounded rectangle 00231 void Adafruit_GFX::fillRoundRect(int16_t x, int16_t y, int16_t w, 00232 int16_t h, int16_t r, uint16_t color) 00233 { 00234 // smarter version 00235 fillRect(x+r, y, w-2*r, h, color); 00236 00237 // draw four corners 00238 fillCircleHelper(x+w-r-1, y+r, r, 1, h-2*r-1, color); 00239 fillCircleHelper(x+r , y+r, r, 2, h-2*r-1, color); 00240 } 00241 00242 // Draw a triangle 00243 void Adafruit_GFX::drawTriangle(int16_t x0, int16_t y0, 00244 int16_t x1, int16_t y1, 00245 int16_t x2, int16_t y2, uint16_t color) 00246 { 00247 drawLine(x0, y0, x1, y1, color); 00248 drawLine(x1, y1, x2, y2, color); 00249 drawLine(x2, y2, x0, y0, color); 00250 } 00251 00252 // Fill a triangle 00253 void Adafruit_GFX::fillTriangle ( int16_t x0, int16_t y0, 00254 int16_t x1, int16_t y1, 00255 int16_t x2, int16_t y2, uint16_t color) 00256 { 00257 00258 int16_t a, b, y, last; 00259 00260 // Sort coordinates by Y order (y2 >= y1 >= y0) 00261 if (y0 > y1) { 00262 swap(y0, y1); 00263 swap(x0, x1); 00264 } 00265 if (y1 > y2) { 00266 swap(y2, y1); 00267 swap(x2, x1); 00268 } 00269 if (y0 > y1) { 00270 swap(y0, y1); 00271 swap(x0, x1); 00272 } 00273 00274 if(y0 == y2) { // Handle awkward all-on-same-line case as its own thing 00275 a = b = x0; 00276 if(x1 < a) a = x1; 00277 else if(x1 > b) b = x1; 00278 if(x2 < a) a = x2; 00279 else if(x2 > b) b = x2; 00280 drawFastHLine(a, y0, b-a+1, color); 00281 return; 00282 } 00283 00284 int16_t 00285 dx01 = x1 - x0, 00286 dy01 = y1 - y0, 00287 dx02 = x2 - x0, 00288 dy02 = y2 - y0, 00289 dx12 = x2 - x1, 00290 dy12 = y2 - y1, 00291 sa = 0, 00292 sb = 0; 00293 00294 // For upper part of triangle, find scanline crossings for segments 00295 // 0-1 and 0-2. If y1=y2 (flat-bottomed triangle), the scanline y1 00296 // is included here (and second loop will be skipped, avoiding a /0 00297 // error there), otherwise scanline y1 is skipped here and handled 00298 // in the second loop...which also avoids a /0 error here if y0=y1 00299 // (flat-topped triangle). 00300 if(y1 == y2) last = y1; // Include y1 scanline 00301 else last = y1-1; // Skip it 00302 00303 for(y=y0; y<=last; y++) { 00304 a = x0 + sa / dy01; 00305 b = x0 + sb / dy02; 00306 sa += dx01; 00307 sb += dx02; 00308 /* longhand: 00309 a = x0 + (x1 - x0) * (y - y0) / (y1 - y0); 00310 b = x0 + (x2 - x0) * (y - y0) / (y2 - y0); 00311 */ 00312 if(a > b) swap(a,b); 00313 drawFastHLine(a, y, b-a+1, color); 00314 } 00315 00316 // For lower part of triangle, find scanline crossings for segments 00317 // 0-2 and 1-2. This loop is skipped if y1=y2. 00318 sa = dx12 * (y - y1); 00319 sb = dx02 * (y - y0); 00320 for(; y<=y2; y++) { 00321 a = x1 + sa / dy12; 00322 b = x0 + sb / dy02; 00323 sa += dx12; 00324 sb += dx02; 00325 /* longhand: 00326 a = x1 + (x2 - x1) * (y - y1) / (y2 - y1); 00327 b = x0 + (x2 - x0) * (y - y0) / (y2 - y0); 00328 */ 00329 if(a > b) swap(a,b); 00330 drawFastHLine(a, y, b-a+1, color); 00331 } 00332 } 00333 00334 void Adafruit_GFX::drawBitmap(int16_t x, int16_t y, const uint8_t *bitmap, int16_t w, int16_t h, uint16_t color) 00335 { 00336 int16_t i, j; 00337 for(j=0; j<h; j++) { 00338 for(i=0; i<w; i++ ) { 00339 if(bitmap[i+(j/8)*w]& _BV(j%8) ) 00340 drawPixel(x+i, y+j, color); 00341 } 00342 } 00343 } 00344 00345 size_t Adafruit_GFX::writeChar(uint8_t c) 00346 { 00347 if (c == '\n') { 00348 cursor_y += textsize*8; 00349 cursor_x = 0; 00350 } else if (c == '\r') { 00351 cursor_x = 0; 00352 } else { 00353 drawChar(cursor_x, cursor_y, c, textcolor, textbgcolor, textsize); 00354 cursor_x += textsize*6; 00355 if (wrap && (cursor_x > (_width - textsize*6))) { 00356 cursor_y += textsize*8; 00357 cursor_x = 0; 00358 } 00359 } 00360 return 1; 00361 } 00362 00363 // Draw a character 00364 void Adafruit_GFX::drawChar(int16_t x, int16_t y, unsigned char c, uint16_t color, uint16_t bg, uint8_t size) 00365 { 00366 if((x >= _width)|| // Clip right 00367 (y >= _height)|| // Clip bottom 00368 ((x + 6 * size - 1) < 0) || // Clip left 00369 ((y + 8 * size - 1) < 0))// Clip top 00370 return; 00371 00372 for (int8_t i=0; i<6; i++ ) { 00373 uint8_t line; 00374 if (i == 5) 00375 line = 0x0; 00376 else 00377 line = font[(c*5)+i]; 00378 for (int8_t j = 0; j<8; j++) { 00379 if (line & 0x1) { 00380 if (size == 1) // default size 00381 drawPixel(x+i, y+j, color); 00382 else { // big size 00383 fillRect(x+(i*size), y+(j*size), size, size, color); 00384 } 00385 } else if (bg != color) { 00386 if (size == 1) // default size 00387 drawPixel(x+i, y+j, bg); 00388 else { // big size 00389 fillRect(x+i*size, y+j*size, size, size, bg); 00390 } 00391 } 00392 line >>= 1; 00393 } 00394 } 00395 } 00396 00397 00398 void Adafruit_GFX::invertDisplay(bool i) 00399 { 00400 // Do nothing, must be subclassed if supported 00401 } 00402 00403
Generated on Mon Aug 15 2022 09:37:08 by
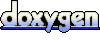