
Question 1 for Embed Lab
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 // My first program did not have an interrupt and as a result sometime it would 00002 // display the button twice. this was resolved by the use on an interrupt. 00003 // A hardware option to control debouncing would be the use of a capacitor 00004 // this will allow for a smooth switch. For a software solution an interrupt is 00005 // used for debouncing. 00006 00007 #include "mbed.h" 00008 Serial pc (USBTX, USBRX); 00009 00010 InterruptIn center(p14); 00011 InterruptIn left(p13); 00012 InterruptIn right(p16); 00013 InterruptIn up(p15); 00014 InterruptIn down(p12); 00015 00016 void center_released() { wait (0.25); 00017 } 00018 void center_pressed() { 00019 pc.printf("center\n\r"); 00020 } 00021 void left_released() { wait (0.25); 00022 } 00023 void left_pressed() { 00024 pc.printf("left\n\r"); 00025 } 00026 void right_released() { wait (0.25); 00027 } 00028 void right_pressed() { 00029 pc.printf("right\n\r"); 00030 } 00031 void up_released() { wait (0.25); 00032 } 00033 void up_pressed() { 00034 pc.printf("up\n\r"); 00035 } 00036 void down_released() { wait (0.25); 00037 } 00038 void down_pressed() { 00039 pc.printf("down\n\r"); 00040 } 00041 int main() { 00042 00043 // Both rise and fall edges generate an interrupt 00044 center.fall(¢er_released); 00045 center.rise(¢er_pressed); 00046 left.fall(&left_released); 00047 left.rise(&left_pressed); 00048 right.fall(&right_released); 00049 right.rise(&right_pressed); 00050 up.fall(&up_released); 00051 up.rise(&up_pressed); 00052 down.fall(&down_released); 00053 down.rise(&down_pressed); 00054 00055 left.mode(PullDown); 00056 right.mode(PullDown); 00057 up.mode(PullDown); 00058 down.mode(PullDown); 00059 00060 while (1) { 00061 } 00062 }
Generated on Sat Jun 10 2023 11:49:12 by
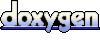