
Pulse measurement; timer; int; to LCD
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 //imp_mess_007.cpp 00002 //*********************** 00003 //* Pulse measurement * 00004 //*********************** 00005 /* D0-ra jel sorozat a USB Flight Simulator-ról, egy npn tranyós jelszint erősítő és fázis fordítón keresztül 00006 * 00007 * min: 420us 00008 * D0 . . . __________[]___[]___[]_[]___[]___[]___[]___[]_______ . . . ___[]___[]___[]___[]___[]___[]___[]______ . . . 00009 ^^ ^^ ^ ^ ^ ^ ^ ^ 00010 trigers Ch3 Ch5 szinkron impulzus 00011 00012 * input = D0(PTA1) 00013 * trigger imp.: ~400 us 00014 * Max. imp.: ~1500 us 00015 * Min. imp.: ~760 us 00016 * Szinkron imp. >8000 us 00017 */ 00018 00019 00020 #include "mbed.h" 00021 #include "TextLCD.h" 00022 00023 InterruptIn int1(D0); 00024 00025 TextLCD lcd(PTB8, PTB9, PTB10, PTB11, PTE2, PTE3); // rs, e, d4-d7 Aruino lábkiosztással 00026 00027 extern int imp = 0; 00028 extern int Ch1 = 0; // Ch1 - Ch7 megjelenítő tároló 00029 extern int Ch2 = 0; 00030 extern int Ch3 = 0; 00031 extern int Ch4 = 0; 00032 extern int Ch5 = 0; 00033 extern int Ch6 = 0; 00034 extern int Ch7 = 0; 00035 extern unsigned Ch_counter = 0; //csatorna számláló 00036 00037 Timer timer1; 00038 00039 00040 DigitalOut K_led(LED1); 00041 DigitalOut Z_led(LED2); 00042 DigitalOut P_led(LED3); 00043 00044 00045 //************************************************ 00046 //************************************************ 00047 void szinkron(){ 00048 //megnézi aktuális Imp szinkron jel-e Imp>3000 00049 //Ch1=imp; 00050 //return; 00051 if (imp>float(5000)){ 00052 Ch_counter = 7; //csatorna számláló 00053 imp = 0; 00054 return; 00055 } 00056 switch(Ch_counter){ 00057 case 7: 00058 //P_led=0; 00059 Ch1 = imp; 00060 Ch_counter--; 00061 break; 00062 case 6: 00063 //P_led=0; 00064 Ch2 = imp; 00065 Ch_counter--; 00066 break; 00067 case 5: 00068 Ch3 = imp; 00069 Ch_counter--; 00070 break; 00071 case 4: 00072 Ch4 = imp; 00073 Ch_counter--; 00074 break; 00075 case 3: 00076 Ch5 = imp; 00077 Ch_counter--; 00078 break; 00079 case 2: 00080 Ch6 = imp; 00081 lcd.locate(0,0); // LCD 00082 lcd.printf(":%d \n", Ch1); 00083 lcd.locate(5,0); 00084 lcd.printf(":%d \n", Ch2); 00085 lcd.locate(11,0); 00086 lcd.printf(":%d \n", Ch3); 00087 lcd.locate(0,1); 00088 lcd.printf(":%d \n", Ch4); 00089 lcd.locate(5,1); 00090 lcd.printf(":%d \n", Ch5); 00091 lcd.locate(11,1); 00092 lcd.printf(":%d \n", Ch6); 00093 Ch_counter--; 00094 break; 00095 default: 00096 Ch7 = imp; 00097 break; 00098 } 00099 } 00100 //************************************************ 00101 void tm1_start() { 00102 //Z_led=0; 00103 timer1.start(); 00104 } 00105 //************************************************ 00106 void tm1_stop() { 00107 //P_led=0; 00108 imp=timer1.read_us(); 00109 timer1.stop(); 00110 timer1.reset(); 00111 szinkron(); 00112 } 00113 //************************************************ 00114 //************************************************ 00115 int main() { 00116 K_led=1; 00117 Z_led=1; 00118 P_led=1; 00119 lcd.cls(); 00120 lcd.printf("Pulse measuremen\n"); 00121 lcd.printf("imp_mess_007.cpp\n"); 00122 wait(1); 00123 lcd.cls(); // TextLCD.h ban leirt fuggveny 00124 00125 int1.fall(&tm1_start); //Piros 00126 int1.rise(&tm1_stop); //Zöld 00127 while(1) { 00128 K_led = !K_led; 00129 wait(0.25); 00130 00131 00132 } 00133 }
Generated on Sun Jul 17 2022 20:11:08 by
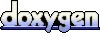