
FRMD KL25Z <=i2c=> NXP PCF8563 (RTC) FRMD KL25Z SPI=> MCP23S17 => (GLCD) T6963C FRMD KL25Z Tsi => 3 button : <--> <enter> <++> FRMD KL25Z => Bip()
Fork of RTC8564NB_Clock by
RTC.h
00001 #ifndef RTC_H 00002 #define RTC_H 00003 00004 #include "mbed.h" 00005 #include "GLCD_spi.h" 00006 #include "TouchMenu.h" 00007 00008 #define RTC8563 0xA2 00009 00010 #define CONTROL1 0x00 00011 #define CONTROL2 0x01 00012 #define SECONDS 0x02 00013 #define MINUTES 0x03 00014 #define HOURS 0x04 00015 #define DAYS 0x05 00016 #define WEEKDAYS 0x06 00017 #define MONTHS 0x07 00018 #define YEARS 0x08 00019 #define MINUTE_ALARM 0x09 00020 #define HOUR_ALARM 0x0A 00021 #define DAY_ALARM 0x0B 00022 #define WEEKDAY_ALARM 0x0C 00023 #define CLOCKOUT_FREQ 0x0D 00024 #define TIMER_CINTROL 0x0E 00025 #define TIMER 0x0F 00026 #define _READ 0x01 00027 00028 I2C i2c(PTE0, PTE1); //SDA, SCL 00029 TouchRun TchR2; 00030 DigitalOut K_LED(LED1), P_LED(LED3), Z_LED(LED2); 00031 00032 00033 char year, month, day, week, hour, minute, sec; 00034 int iyear, imonth, iday, iweek, ihour, iminute, isec; 00035 char ntp_year[3], ntp_month[3], ntp_day[3], ntp_week[4]; 00036 char ntp_hour[3], ntp_minute[3], ntp_sec[3]; 00037 char week_val; 00038 00039 char week_chr[7][4] = {"Vas","Het","Ked","Sze","Csu","Pen","Szo"}; 00040 //------------------------------------------------------------------- 00041 void rtc_write(char address, char value){ 00042 i2c.start(); 00043 i2c.write(RTC8563); 00044 i2c.write(address); 00045 i2c.write(value); 00046 i2c.stop(); 00047 } 00048 //------------------------------------------------------------------- 00049 char rtc_read(char address){ 00050 char value; 00051 i2c.start(); 00052 i2c.write(RTC8563); 00053 i2c.write(address); 00054 i2c.start(); 00055 i2c.write(RTC8563 | _READ); 00056 value = i2c.read(0); 00057 i2c.stop(); 00058 00059 return value; 00060 } 00061 //------------------------------------------------------------------- 00062 void time_just(){ 00063 char _min, _hour; 00064 //test_led = !test_led; 00065 _min = rtc_read(MINUTES); 00066 if (_min >= 0x30) { 00067 _hour = rtc_read(HOURS); 00068 if (_hour == 0x23) 00069 _hour = 0x00; 00070 else if ((_hour & 0x0F) == 0x09) 00071 _hour = (_hour & 0xF0) + 0x10; 00072 else 00073 _hour = _hour + 0x01; 00074 rtc_write(HOURS, _hour); 00075 } 00076 rtc_write(MINUTES, 0x00); 00077 rtc_write(SECONDS, 0x00); 00078 } 00079 //------------------------------------------------------------------ 00080 void rtc_reset(){ 00081 //just_button.rise(&time_just); 00082 00083 lcd_cls(); 00084 lcd_string(2,1,"RTC8563 CLOCK RESET" ); 00085 wait(1); 00086 00087 rtc_write(CONTROL1, 0x20); //stop 00088 rtc_write(CONTROL2, 0x00); 00089 rtc_write(YEARS, 0x36); //((ntp_year[0]-0x30)<<4)+(ntp_year[1]-0x30)); 00090 rtc_write(MONTHS,0x02); //((ntp_month[0]-0x30)<<4)+(ntp_month[1]-0x30)); 00091 rtc_write(DAYS, 0x03); //((ntp_day[0]-0x30)<<4)+(ntp_day[1]-0x30)); 00092 rtc_write(HOURS, 0x04); //((ntp_hour[0]-0x30)<<4)+(ntp_hour[1]-0x30)); 00093 rtc_write(MINUTES,0x05); //((ntp_minute[0]-0x30)<<4)+(ntp_minute[1]-0x30)); 00094 rtc_write(SECONDS,0x00); //((ntp_sec[0]-0x30)<<4)+(ntp_sec[1]-0x30)); 00095 rtc_write(WEEKDAYS, week_val); 00096 rtc_write(CLOCKOUT_FREQ, 0x00); // 0x83 = TE on & 1Hz 00097 rtc_write(TIMER_CINTROL, 0x00); 00098 rtc_write(CONTROL1, 0x00); //start 00099 } 00100 //------------------------------------------------------------------ 00101 void rtc_data_read(){ 00102 i2c.start(); 00103 i2c.write(0xA2); 00104 i2c.write(0x02); 00105 i2c.stop(); 00106 i2c.start(); 00107 i2c.write(0xA3); 00108 sec = i2c.read(SECONDS) & 0x7F; 00109 minute = i2c.read(MINUTES) & 0x7F; 00110 hour = i2c.read(HOURS) & 0x3F; 00111 day = i2c.read(DAYS) & 0x3F; 00112 week = i2c.read(WEEKDAYS) & 0x07; 00113 month = i2c.read(MONTHS) & 0x1F; 00114 year = i2c.read(YEARS); 00115 i2c.stop(); 00116 00117 00118 //lcd.locate(0,0); 00119 sprintf(buffer,"%c%c/%c%c/%c%c %s", 00120 ((year >> 4) & 0x0F) + 0x30, (year & 0x0F) + 0x30, //int((year >> 4)& 0x0F)*10 + int(year & 0x0F), 00121 ((month >> 4) & 0x01) + 0x30, (month & 0x0F) + 0x30, 00122 ((day >> 4) & 0x03)+ 0x30, (day & 0x0F) + 0x30, 00123 week_chr[week & 0x07]); 00124 lcd_string(0,0,buffer); 00125 //lcd.locate(0,1); 00126 sprintf(buffer,"%c%c:%c%c:%c%c", 00127 ((hour >> 4) & 0x03) + 0x30, (hour & 0x0F) + 0x30, 00128 (minute >> 4) + 0x30, (minute & 0x0F) + 0x30, 00129 (sec >> 4) + 0x30, (sec & 0x0F) + 0x30 ); 00130 lcd_string(0,13,buffer); 00131 } 00132 //-------------------------------------------------------------- 00133 void rtc_data_set(){ 00134 00135 rtc_data_read(); 00136 //-------------------------------------------------- 00137 year = (int)((((year >> 4)& 0x0F)*10) + (year & 0x0F)); 00138 00139 sprintf(buffer,"Year: "); 00140 year = SetBox(year, 0, 99); 00141 Bip(1,1); 00142 00143 sprintf(buffer,"%d",year); 00144 if(year > 9){ // két helyiértékesetén 00145 year = buffer[0] & 0x0F; 00146 year = (year<<4) + (buffer[1] & 0x0F); 00147 }else{ // egy helyiérték esetén 00148 year = buffer[0] & 0x0F; 00149 } 00150 // a visszaalakítás azonos mint a beolvasásnál 00151 //sprintf(buffer,"Year:%c%c", ((year >> 4) & 0x0F) + 0x30, (year & 0x0F) + 0x30); 00152 //lcd_string(5,0,buffer); 00153 //-------------------------------------------------- 00154 month = (int)((((month >> 4)& 0x01)*10) + (month & 0x0F)); 00155 00156 sprintf(buffer,"Month: "); 00157 month = SetBox(month, 1, 12); 00158 Bip(1,1); 00159 00160 sprintf(buffer,"%d",month); 00161 if(month > 9){ // két helyiértékesetén 00162 month = buffer[0] & 0x0F; 00163 month = (month<<4) + (buffer[1] & 0x0F); 00164 }else{ // egy helyiérték esetén 00165 month = buffer[0] & 0x0F; 00166 } 00167 //-------------------------------------------------- 00168 day = (int)((((day >> 4)& 0x03)*10) + (day & 0x0F)); 00169 00170 sprintf(buffer,"Day: "); 00171 day = SetBox(day, 1, 31); 00172 Bip(1,1); 00173 00174 sprintf(buffer,"%d",day); 00175 if(day > 9){ // két helyiértékesetén 00176 day = buffer[0] & 0x0F; 00177 day = (day<<4) + (buffer[1] & 0x0F); 00178 }else{ // egy helyiérték esetén 00179 day = buffer[0] & 0x0F; 00180 } 00181 00182 //-------------------------------------------------- 00183 week = (int)((((week >> 4)& 0x03)*10) + (week & 0x0F)); 00184 00185 sprintf(buffer,"Week: "); 00186 week = SetBox(week + 1, 1, 7); 00187 Bip(1,1); 00188 00189 sprintf(buffer,"%d",week - 1); 00190 if(week > 9){ // két helyiértékesetén 00191 week = buffer[0] & 0x0F; 00192 week = (week<<4) + (buffer[1] & 0x0F); 00193 }else{ // egy helyiérték esetén 00194 week = buffer[0] & 0x0F; 00195 } 00196 00197 //-------------------------------------------------- 00198 hour = (int)((((hour >> 4)& 0x03)*10) + (hour & 0x0F)); 00199 00200 sprintf(buffer,"Hour: "); 00201 hour = SetBox(hour, 0, 23); 00202 Bip(1,1); 00203 00204 sprintf(buffer,"%d",hour); 00205 if(hour > 9){ // két helyiértékesetén 00206 hour = buffer[0] & 0x0F; 00207 hour = (hour<<4) + (buffer[1] & 0x0F); 00208 }else{ // egy helyiérték esetén 00209 hour = buffer[0] & 0x0F; 00210 } 00211 00212 //-------------------------------------------------- 00213 minute = (int)((((minute >> 4)& 0x07)*10) + (minute & 0x0F)); 00214 00215 sprintf(buffer,"Minute: "); 00216 minute = SetBox(minute, 0, 23); 00217 Bip(1,1); 00218 00219 sprintf(buffer,"%d",minute); 00220 if(minute > 9){ // két helyiértékesetén 00221 minute = buffer[0] & 0x0F; 00222 minute = (minute<<4) + (buffer[1] & 0x0F); 00223 }else{ // egy helyiérték esetén 00224 minute = buffer[0] & 0x0F; 00225 } 00226 //------------------------------------------------- 00227 00228 rtc_write(CONTROL1, 0x20); //stop 00229 rtc_write(CONTROL2, 0x00); 00230 rtc_write(YEARS, year); //((ntp_year[0]-0x30)<<4)+(ntp_year[1]-0x30)); 00231 rtc_write(MONTHS,month); 00232 rtc_write(DAYS, day); 00233 rtc_write(WEEKDAYS,week); 00234 rtc_write(HOURS, hour); 00235 rtc_write(MINUTES, minute); 00236 rtc_write(SECONDS,0x00); 00237 rtc_write(CONTROL1, 0x00); //start 00238 } 00239 00240 00241 00242 00243 00244 00245 00246 #endif
Generated on Mon Jul 18 2022 01:34:26 by
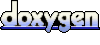