
a
Fork of Modbus by
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /* 00002 * swk 00003 * The program is a sample code. 00004 * It needs run with some NuMaker-PFM-NUC472 boards. 00005 * 00006 * Please remeber to modify global definition to enable RS485 port on board. 00007 * Modify '//#define DEF_RS485_PORT 1' to '#define DEF_RS485_PORT 1' 00008 https://developer.mbed.org/users/wclin/code/modbus-over-rs485-sample/file/f9d748875768/main.cpp 00009 */ 00010 00011 /* ----------------------- System includes --------------------------------*/ 00012 #include "mbed.h" 00013 #include "rtos.h" 00014 /*----------------------- Modbus includes ----------------------------------*/ 00015 #include "mb.h" 00016 #include "mbport.h" 00017 00018 /* ----------------------- Defines ------------------------------------------*/ 00019 // Sharing buffer index 00020 enum { 00021 eData_MBInCounter, 00022 eData_MBOutCounter, 00023 eData_MBError, 00024 eData_DI, 00025 eData_DATA, 00026 eData_Cnt 00027 } E_DATA_TYPE; 00028 00029 #define REG_INPUT_START 1 00030 #define REG_INPUT_NREGS eData_Cnt 00031 /* ----------------------- Static variables ---------------------------------*/ 00032 static USHORT usRegInputStart = REG_INPUT_START; 00033 static USHORT usRegInputBuf[REG_INPUT_NREGS]; 00034 00035 DigitalOut led1(LED1); // For temperature worker. 00036 DigitalOut led2(LED2); // For Modbus worker. 00037 DigitalOut led3(LED3); // For Holder CB 00038 00039 #define DEF_PIN_NUM 6 00040 DigitalIn DipSwitch[DEF_PIN_NUM] = { PG_1, PG_2, PF_9, PF_10, PC_10, PC_11 } ; 00041 00042 unsigned short GetValueOnDipSwitch() 00043 { 00044 int i=0; 00045 unsigned short usDipValue = 0x0; 00046 for ( i=0; i<DEF_PIN_NUM ; i++) 00047 usDipValue |= DipSwitch[i].read() << i; 00048 usDipValue = (~usDipValue) & 0x003F; 00049 return usDipValue; 00050 } 00051 00052 void worker_uart(void const *args) 00053 { 00054 int counter=0; 00055 // For UART-SERIAL Tx/Rx Service. 00056 while (true) 00057 { 00058 //xMBPortSerialPolling(); 00059 if ( counter > 10000 ) 00060 { 00061 led2 = !led2; 00062 counter=0; 00063 } 00064 counter++; 00065 } 00066 } 00067 00068 /* ----------------------- Start implementation -----------------------------*/ 00069 int 00070 main( void ) 00071 { 00072 eMBErrorCode eStatus; 00073 //Thread uart_thread(worker_uart); 00074 unsigned short usSlaveID=GetValueOnDipSwitch(); 00075 00076 // Initialise some registers 00077 for (int i=0; i<REG_INPUT_NREGS; i++) 00078 usRegInputBuf[i] = 0x0; 00079 00080 printf("We will set modbus slave ID-%d(0x%x) for the device.\r\n", usSlaveID, usSlaveID ); 00081 00082 /* Enable the Modbus Protocol Stack. */ 00083 if ( (eStatus = eMBInit( MB_RTU , usSlaveID, 0, 115200, MB_PAR_NONE )) != MB_ENOERR ) 00084 goto FAIL_MB; 00085 else if ( (eStatus = eMBEnable( ) ) != MB_ENOERR ) 00086 goto FAIL_MB_1; 00087 else { 00088 for( ;; ) 00089 { 00090 xMBPortSerialPolling(); 00091 if ( eMBPoll( ) != MB_ENOERR ) break; 00092 } 00093 } 00094 00095 FAIL_MB_1: 00096 eMBClose(); 00097 00098 FAIL_MB: 00099 for( ;; ) 00100 { 00101 led2 = !led2; 00102 Thread::wait(200); 00103 } 00104 } 00105 00106 00107 00108 00109 eMBErrorCode 00110 eMBRegInputCB( UCHAR * pucRegBuffer, USHORT usAddress, USHORT usNRegs ) 00111 { 00112 eMBErrorCode eStatus = MB_ENOERR ; 00113 int iRegIndex; 00114 00115 if( ( usAddress >= REG_INPUT_START ) 00116 && ( usAddress + usNRegs <= REG_INPUT_START + REG_INPUT_NREGS ) ) 00117 { 00118 iRegIndex = ( int )( usAddress - usRegInputStart ); 00119 while( usNRegs > 0 ) 00120 { 00121 *pucRegBuffer++ = 00122 ( unsigned char )( usRegInputBuf[iRegIndex] >> 8 ); 00123 *pucRegBuffer++ = 00124 ( unsigned char )( usRegInputBuf[iRegIndex] & 0xFF ); 00125 iRegIndex++; 00126 usNRegs--; 00127 } 00128 } 00129 else 00130 { 00131 eStatus = MB_ENOREG ; 00132 } 00133 00134 return eStatus; 00135 } 00136 00137 eMBErrorCode 00138 eMBRegHoldingCB( UCHAR * pucRegBuffer, USHORT usAddress, USHORT usNRegs, eMBRegisterMode eMode ) 00139 { 00140 eMBErrorCode eStatus = MB_ENOERR ; 00141 int iRegIndex; 00142 00143 usRegInputBuf[eData_MBInCounter]++; 00144 usRegInputBuf[eData_MBOutCounter]++; 00145 00146 if (eMode == MB_REG_READ ) 00147 { 00148 usRegInputBuf[eData_DI] = GetValueOnDipSwitch(); 00149 00150 if( ( usAddress >= REG_INPUT_START ) 00151 && ( usAddress + usNRegs <= REG_INPUT_START + REG_INPUT_NREGS ) ) 00152 { 00153 iRegIndex = ( int )( usAddress - usRegInputStart ); 00154 while( usNRegs > 0 ) 00155 { 00156 *pucRegBuffer++ = 00157 ( unsigned char )( usRegInputBuf[iRegIndex] >> 8 ); 00158 *pucRegBuffer++ = 00159 ( unsigned char )( usRegInputBuf[iRegIndex] & 0xFF ); 00160 iRegIndex++; 00161 usNRegs--; 00162 } 00163 } 00164 } 00165 00166 if (eMode == MB_REG_WRITE ) 00167 { 00168 if( ( usAddress >= REG_INPUT_START ) 00169 && ( usAddress + usNRegs <= REG_INPUT_START + REG_INPUT_NREGS ) ) 00170 { 00171 iRegIndex = ( int )( usAddress - usRegInputStart ); 00172 while( usNRegs > 0 ) 00173 { 00174 usRegInputBuf[iRegIndex] = ((unsigned int) *pucRegBuffer << 8) | ((unsigned int) *(pucRegBuffer+1)); 00175 pucRegBuffer+=2; 00176 iRegIndex++; 00177 usNRegs--; 00178 } 00179 } 00180 } 00181 00182 led3=!led3; 00183 00184 return eStatus; 00185 } 00186 00187 00188 eMBErrorCode 00189 eMBRegCoilsCB( UCHAR * pucRegBuffer, USHORT usAddress, USHORT usNCoils, 00190 eMBRegisterMode eMode ) 00191 { 00192 return MB_ENOREG ; 00193 } 00194 00195 eMBErrorCode 00196 eMBRegDiscreteCB( UCHAR * pucRegBuffer, USHORT usAddress, USHORT usNDiscrete ) 00197 { 00198 return MB_ENOREG ; 00199 }
Generated on Tue Jul 12 2022 23:11:12 by
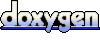