max32630fthr library changed to pointer
Fork of max32630fthr by
Embed:
(wiki syntax)
Show/hide line numbers
max32630fthr.cpp
00001 /******************************************************************************* 00002 * Copyright (C) 2016 Maxim Integrated Products, Inc., All Rights Reserved. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a 00005 * copy of this software and associated documentation files (the "Software"), 00006 * to deal in the Software without restriction, including without limitation 00007 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 * and/or sell copies of the Software, and to permit persons to whom the 00009 * Software is furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included 00012 * in all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00015 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00016 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00017 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00018 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00019 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00020 * OTHER DEALINGS IN THE SOFTWARE. 00021 * 00022 * Except as contained in this notice, the name of Maxim Integrated 00023 * Products, Inc. shall not be used except as stated in the Maxim Integrated 00024 * Products, Inc. Branding Policy. 00025 * 00026 * The mere transfer of this software does not imply any licenses 00027 * of trade secrets, proprietary technology, copyrights, patents, 00028 * trademarks, maskwork rights, or any other form of intellectual 00029 * property whatsoever. Maxim Integrated Products, Inc. retains all 00030 * ownership rights. 00031 ******************************************************************************* 00032 */ 00033 00034 #include "mbed.h" 00035 #include "max3263x.h" 00036 #include "ioman_regs.h" 00037 #include "PinNames.h" 00038 #include "max32630fthr.h" 00039 00040 //****************************************************************************** 00041 MAX32630FTHR::MAX32630FTHR() : i2c(P5_7, P6_0), max14690(&i2c) 00042 { 00043 } 00044 00045 //****************************************************************************** 00046 MAX32630FTHR::MAX32630FTHR(vio_t vio) : i2c(P5_7, P6_0), max14690(&i2c) 00047 { 00048 init(vio); 00049 } 00050 00051 //****************************************************************************** 00052 MAX32630FTHR::~MAX32630FTHR() 00053 { 00054 } 00055 00056 //****************************************************************************** 00057 int MAX32630FTHR::init(vio_t hdrVio) 00058 { 00059 // Override the default values 00060 max14690.ldo2Millivolts = 3300; 00061 max14690.ldo3Millivolts = 3300; 00062 max14690.ldo2Mode = MAX14690::LDO_ENABLED; 00063 max14690.ldo3Mode = MAX14690::LDO_ENABLED; 00064 max14690.monCfg = MAX14690::MON_HI_Z; 00065 // Note that writing the local value does directly affect the part 00066 // The buck-boost regulator will remain off until init is called 00067 00068 // Call init to apply all settings to the PMIC 00069 if (max14690.init() == MAX14690_ERROR) { 00070 printf("Error initializing MAX14690"); 00071 } 00072 00073 // Set micro SD card pins to 3.3V 00074 vddioh(P0_4, VIO_3V3); 00075 vddioh(P0_5, VIO_3V3); 00076 vddioh(P0_6, VIO_3V3); 00077 vddioh(P0_7, VIO_3V3); 00078 // Set LED pins to 3.3V 00079 vddioh(P2_4, VIO_3V3); 00080 vddioh(P2_5, VIO_3V3); 00081 vddioh(P2_6, VIO_3V3); 00082 // Set header pins to hdrVio 00083 vddioh(P3_0, hdrVio); 00084 vddioh(P3_1, hdrVio); 00085 vddioh(P3_2, hdrVio); 00086 vddioh(P3_3, hdrVio); 00087 vddioh(P3_4, hdrVio); 00088 vddioh(P3_5, hdrVio); 00089 vddioh(P4_0, hdrVio); 00090 vddioh(P4_1, hdrVio); 00091 vddioh(P4_2, hdrVio); 00092 vddioh(P4_3, hdrVio); 00093 vddioh(P4_4, hdrVio); 00094 vddioh(P4_5, hdrVio); 00095 vddioh(P4_6, hdrVio); 00096 vddioh(P4_7, hdrVio); 00097 vddioh(P5_0, hdrVio); 00098 vddioh(P5_1, hdrVio); 00099 vddioh(P5_2, hdrVio); 00100 vddioh(P5_3, hdrVio); 00101 vddioh(P5_4, hdrVio); 00102 vddioh(P5_5, hdrVio); 00103 vddioh(P5_6, hdrVio); 00104 00105 return 0; 00106 } 00107 00108 //****************************************************************************** 00109 int MAX32630FTHR::vddioh(PinName pin, vio_t vio) 00110 { 00111 __IO uint32_t *use_vddioh = &((mxc_ioman_regs_t *)MXC_IOMAN)->use_vddioh_0; 00112 00113 if (pin == NOT_CONNECTED) { 00114 return -1; 00115 } 00116 00117 use_vddioh += PINNAME_TO_PORT(pin) >> 2; 00118 if (vio) { 00119 *use_vddioh |= (1 << (PINNAME_TO_PIN(pin) + ((PINNAME_TO_PORT(pin) & 0x3) << 3))); 00120 } else { 00121 *use_vddioh &= ~(1 << (PINNAME_TO_PIN(pin) + ((PINNAME_TO_PORT(pin) & 0x3) << 3))); 00122 } 00123 00124 return 0; 00125 }
Generated on Sun Aug 28 2022 12:52:07 by
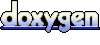