MAX32625PICO Self Test
Dependencies: USBDevice maxim-dev mbed-rtos
Fork of FTHR_self_test by
main.cpp
00001 #include "mbed.h" 00002 #include "rtos.h" 00003 #include "USBKeyboard.h" 00004 00005 // Hardware serial port over DAPLink 00006 Serial daplink(P2_1, P2_0); 00007 00008 DigitalOut rLED(LED1, LED_ON); 00009 DigitalOut gLED(LED2, LED_OFF); 00010 DigitalOut bLED(LED3, LED_OFF); 00011 DigitalIn button(P2_7, PullUp); 00012 DigitalOut en3V3(P3_6, 0); 00013 DigitalOut enIOH(P2_2, 0); 00014 DigitalOut selSWD(P2_3, 0); 00015 AnalogIn dipIOH(AIN_4); 00016 AnalogIn swdIOH(AIN_5); 00017 AnalogIn vddbIn(AIN_6); 00018 AnalogIn vdd18In(AIN_7); 00019 AnalogIn vdd12In(AIN_8); 00020 AnalogIn vrtcIn(AIN_9); 00021 00022 USBKeyboard keyboard; 00023 00024 // Function to report failure 00025 void testFailed() 00026 { 00027 daplink.printf("\r\n! Test Failed !\r\n"); 00028 // rLED = LED_ON; 00029 // gLED = LED_OFF; 00030 // bLED = LED_OFF; 00031 while(1) { 00032 Thread::wait(500); 00033 rLED = !rLED; 00034 } 00035 } 00036 00037 // main() runs in its own thread in the OS 00038 // (note the calls to Thread::wait below for delays) 00039 int main() 00040 { 00041 float aIn; 00042 int ledCnt = 0; 00043 00044 daplink.printf("Checking Supplies\r\n"); 00045 aIn = 2.4f * vdd12In; 00046 daplink.printf("vdd12 = %f\r\n", aIn); 00047 if ((aIn < 1.0f) || (aIn > 1.4f)) { 00048 testFailed(); 00049 } 00050 aIn = 2.4f * vdd18In; 00051 daplink.printf("vdd18 = %f\r\n", aIn); 00052 if ((aIn < 1.6f) || (aIn > 2.0f)) { 00053 testFailed(); 00054 } 00055 aIn = 4.8f * vddbIn; 00056 daplink.printf("vddb = %f\r\n", aIn); 00057 if ((aIn < 3.0f) || (aIn > 3.6f)) { 00058 testFailed(); 00059 } 00060 aIn = 2.4f * vrtcIn; 00061 daplink.printf("vrtc = %f\r\n", aIn); 00062 if ((aIn < 1.6f) || (aIn > 2.0f)) { 00063 testFailed(); 00064 } 00065 00066 en3V3 = 1; 00067 bLED = LED_ON; 00068 Thread::wait(100); 00069 00070 daplink.printf("Checking Switches\r\n"); 00071 aIn = 6.0f * dipIOH; 00072 Thread::wait(100); 00073 aIn = 6.0f * dipIOH; 00074 daplink.printf("dipIOH(open) = %f\r\n", aIn); 00075 if (aIn > 0.1f) { 00076 testFailed(); 00077 } 00078 aIn = 6.0f * swdIOH; 00079 Thread::wait(100); 00080 aIn = 6.0f * swdIOH; 00081 daplink.printf("swdIOH(open) = %f\r\n", aIn); 00082 if (aIn > 0.1f) { 00083 testFailed(); 00084 } 00085 00086 enIOH = 1; 00087 rLED = LED_OFF; 00088 Thread::wait(100); 00089 00090 aIn = 6.0f * dipIOH; 00091 Thread::wait(100); 00092 aIn = 6.0f * dipIOH; 00093 daplink.printf("dipIOH(closed) = %f\r\n", aIn); 00094 // aIn = 6.0f * swdIOH; 00095 // daplink.printf("swdIOH(closed) = %f\r\n", aIn); 00096 if ((aIn < 3.0f) || (aIn > 3.6f)) { 00097 // testFailed(); 00098 } 00099 00100 selSWD = 1; 00101 gLED = LED_ON; 00102 Thread::wait(100); 00103 00104 aIn = 6.0f * swdIOH; 00105 Thread::wait(100); 00106 aIn = 6.0f * swdIOH; 00107 daplink.printf("swdIOH(closed) = %f\r\n", aIn); 00108 // aIn = 6.0f * dipIOH; 00109 // daplink.printf("dipIOH(closed) = %f\r\n", aIn); 00110 if ((aIn < 3.0f) || (aIn > 3.6f)) { 00111 testFailed(); 00112 } 00113 00114 bLED = LED_OFF; 00115 Thread::wait(250); 00116 00117 daplink.printf("Self Test Passed\r\n"); 00118 rLED = LED_ON; 00119 gLED = LED_ON; 00120 bLED = LED_ON; 00121 00122 while (true) { 00123 if (!button) { 00124 keyboard.mediaControl(KEY_MUTE); 00125 ledCnt++; 00126 gLED = (ledCnt & 1); 00127 bLED = (ledCnt & 2); 00128 rLED = gLED ^ bLED; 00129 Thread::wait(500); 00130 } else { 00131 Thread::wait(50); 00132 } 00133 } 00134 } 00135
Generated on Fri Jul 15 2022 08:58:23 by
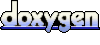