A library for interfacing with the Pixy color recognition camera
Dependents: PixyCamera MbedOS_Robot_Team ManualControlFinal PlayBack ... more
TPixyInterface.h
00001 // 00002 // begin license header 00003 // 00004 // This file is part of Pixy CMUcam5 or "Pixy" for short 00005 // 00006 // All Pixy source code is provided under the terms of the 00007 // GNU General Public License v2 (http://www.gnu.org/licenses/gpl-2.0.html). 00008 // Those wishing to use Pixy source code, software and/or 00009 // technologies under different licensing terms should contact us at 00010 // cmucam@cs.cmu.edu. Such licensing terms are available for 00011 // all portions of the Pixy codebase presented here. 00012 // 00013 // end license header 00014 // 00015 // This file is for defining the SPI-related classes. It's called Pixy.h instead 00016 // of Pixy_SPI.h because it's the default/recommended communication method 00017 // with Arduino. This class assumes you are using the ICSP connector to talk to 00018 // Pixy from your Arduino. For more information go to: 00019 // 00020 //http://cmucam.org/projects/cmucam5/wiki/Hooking_up_Pixy_to_a_Microcontroller_(like_an_Arduino) 00021 // 00022 00023 #ifndef _TPIXY_INTERFACE_H 00024 #define _TPIXY_INTERFACE_H 00025 00026 #include "TPixy.h" 00027 00028 #define PIXY_SYNC_BYTE 0x5a 00029 #define PIXY_SYNC_BYTE_DATA 0x5b 00030 #define PIXY_BUF_SIZE 16 00031 00032 /** An interface for communicating between the Pixy and a specific hardware interface 00033 */ 00034 class TPixyInterface 00035 { 00036 public: 00037 /** Creates a TPixyInterface 00038 */ 00039 TPixyInterface() {} 00040 /** Initializes a TPixyInterface 00041 */ 00042 virtual void init() = 0; 00043 /** Sends the given data to the Pixy with a given data length 00044 * @param data the data to send 00045 * @param len the length of the data to send 00046 * @return the interface return signal 00047 */ 00048 virtual int8_t send(uint8_t *data, uint8_t len) = 0; 00049 /** Sets an argument for the interface to use 00050 * @param arg the argument to use 00051 */ 00052 virtual void setArg(uint16_t arg) = 0; 00053 /** Reads a word from the interface 00054 * @return the word read from the interface 00055 */ 00056 virtual uint16_t getWord() = 0; 00057 /** Reads a byte from the interface 00058 * @return the byte read from the interface 00059 */ 00060 virtual uint8_t getByte() = 0; 00061 }; 00062 00063 00064 template <class BufType> class CircularQ 00065 { 00066 public: 00067 BufType buf[PIXY_BUF_SIZE]; 00068 uint8_t len; 00069 uint8_t writeIndex; 00070 uint8_t readIndex; 00071 00072 CircularQ(); 00073 bool read(BufType *c); 00074 uint8_t freeLen(); 00075 bool write(BufType c); 00076 }; 00077 00078 /** An interface for communicating between the Pixy via an SPI interface 00079 */ 00080 class PixyInterfaceSPI : TPixyInterface 00081 { 00082 public: 00083 SPI* spi; 00084 /** Constructs a PixyInterfaceSPI object 00085 * @param interface the SPI pointer 00086 */ 00087 PixyInterfaceSPI(SPI* interface); 00088 /** Initializes the PixyInterfaceSPI 00089 */ 00090 virtual void init(); 00091 /** Reads a word from the interface 00092 * @return the word read from the interface 00093 */ 00094 virtual uint16_t getWord(); 00095 /** Reads a byte from the interface 00096 * @return the byte read from the interface 00097 */ 00098 virtual uint8_t getByte(); 00099 /** Sends the given data to the Pixy with a given data length 00100 * @param data the data to send 00101 * @param len the length of the data to send 00102 * @return the interface return signal 00103 */ 00104 virtual int8_t send(uint8_t *data, uint8_t len); 00105 /** Sets an argument for the interface to use [unused] 00106 * @param arg the argument to use 00107 */ 00108 virtual void setArg(uint16_t arg); 00109 00110 private: 00111 // we need a little circular queues for both directions 00112 CircularQ<uint8_t> outQ; 00113 CircularQ<uint16_t> inQ; 00114 00115 uint16_t getWordHw(); 00116 void flushSend(); 00117 }; 00118 00119 00120 /* 00121 class PixyInterfaceI2C : TPixyInterface 00122 { 00123 public: 00124 SPI* spi; 00125 PixyInterfaceI2C(I2C* interface); 00126 virtual void init(); 00127 virtual uint16_t getWord(); 00128 virtual uint8_t getByte(); 00129 virtual int8_t send(uint8_t *data, uint8_t len); 00130 virtual void setArg(uint16_t arg); 00131 00132 private: 00133 // we need a little circular queues for both directions 00134 CircularQ<uint8_t> outQ; 00135 CircularQ<uint16_t> inQ; 00136 00137 uint16_t getWordHw(); 00138 void flushSend(); 00139 };*/ 00140 00141 #endif
Generated on Tue Jul 12 2022 18:15:33 by
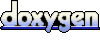