A library for interfacing with the Pixy color recognition camera
Dependents: PixyCamera MbedOS_Robot_Team ManualControlFinal PlayBack ... more
TPixyInterface.cpp
00001 #include "TPixyInterface.h" 00002 00003 PixyInterfaceSPI::PixyInterfaceSPI(SPI* interface) : TPixyInterface(), spi(interface) {} 00004 00005 void PixyInterfaceSPI::init() 00006 { 00007 spi->frequency(1000000); 00008 } 00009 00010 uint16_t PixyInterfaceSPI::getWord() 00011 { 00012 // ordering is different (big endian) because Pixy is sending 16 bits through SPI 00013 // instead of 2 bytes in a 16-bit word as with I2C 00014 uint16_t w; 00015 if (inQ.read(&w)) { 00016 return w; 00017 } 00018 return getWordHw(); 00019 } 00020 00021 uint8_t PixyInterfaceSPI::getByte() 00022 { 00023 //return SPI.transfer(0x00); 00024 return spi->write(0x00); 00025 } 00026 00027 int8_t PixyInterfaceSPI::send(uint8_t *data, uint8_t len) 00028 { 00029 int i; 00030 // check to see if we have enough space in our circular queue 00031 if (outQ.freeLen() < len) { 00032 return -1; 00033 } 00034 for (i = 0; i < len; i++) { 00035 outQ.write(data[i]); 00036 } 00037 flushSend(); 00038 return len; 00039 } 00040 00041 void PixyInterfaceSPI::setArg(uint16_t arg) {} 00042 00043 uint16_t PixyInterfaceSPI::getWordHw() 00044 { 00045 // ordering is different (big endian) because Pixy is sending 16 bits through SPI 00046 // instead of 2 bytes in a 16-bit word as with I2C 00047 uint16_t w; 00048 uint8_t c, c_out = 0; 00049 00050 if (outQ.read(&c_out)) { 00051 w = spi->write(PIXY_SYNC_BYTE_DATA); 00052 //w = SPI.transfer(PIXY_SYNC_BYTE_DATA); 00053 } else { 00054 w = spi->write(PIXY_SYNC_BYTE); 00055 //w = SPI.transfer(PIXY_SYNC_BYTE); 00056 } 00057 w <<= 8; 00058 c = spi->write(c_out); 00059 //c = SPI.transfer(cout); 00060 w |= c; 00061 return w; 00062 } 00063 00064 void PixyInterfaceSPI::flushSend() 00065 { 00066 uint16_t w; 00067 while(outQ.len) { 00068 w = getWordHw(); 00069 inQ.write(w); 00070 } 00071 } 00072 00073 00074 00075 00076 template <class BufType> CircularQ<BufType>::CircularQ() 00077 { 00078 len = 0; 00079 writeIndex = 0; 00080 readIndex = 0; 00081 } 00082 00083 template <class BufType> bool CircularQ<BufType>::read(BufType *c) 00084 { 00085 if (len) { 00086 *c = buf[readIndex++]; 00087 len--; 00088 if (readIndex == PIXY_BUF_SIZE) { 00089 readIndex = 0; 00090 } 00091 return true; 00092 } else { 00093 return false; 00094 } 00095 } 00096 00097 template <class BufType> uint8_t CircularQ<BufType>::freeLen() 00098 { 00099 return PIXY_BUF_SIZE - len; 00100 } 00101 00102 template <class BufType> bool CircularQ<BufType>::write(BufType c) 00103 { 00104 if (freeLen() == 0) { 00105 return false; 00106 } 00107 buf[writeIndex++] = c; 00108 len++; 00109 if (writeIndex == PIXY_BUF_SIZE) { 00110 writeIndex = 0; 00111 } 00112 return true; 00113 }
Generated on Tue Jul 12 2022 18:15:33 by
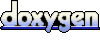