Fully functional driver for the DRV88255 stepper motor driver. Includes microstepping, speed, disable and enable.
Dependents: Lidar_2D_Mapping Water Play Water_pump Lidar_2D_Mapping
DRV8825.cpp
00001 #include "DRV8825.h" 00002 #include "mbed.h" 00003 00004 DigitalOut myled(LED1); 00005 00006 DRV8825::DRV8825(PinName _en, PinName m0, PinName m1, PinName m2, PinName _stepPin, PinName dir):en(_en), 00007 microstepping(m0, m1, m2), 00008 stepPin(_stepPin), 00009 direction(dir) 00010 { 00011 } 00012 00013 void DRV8825::settings(float microstep, int dir, float speed) 00014 { 00015 //Microsteppiing settings 00016 if (microstep == 1) microstepping = 0; 00017 else if (microstep == 1/2) microstepping = 1; 00018 else if (microstep == 1/4) microstepping = 2; 00019 else if (microstep == 1/8) microstepping = 3; 00020 else if (microstep == 1/16) microstepping = 4; 00021 else if (microstep == 1/32) microstepping = 5; 00022 00023 if (dir == 1) { 00024 direction = 0; 00025 } else if (dir == 0) { 00026 direction = 1; 00027 } 00028 00029 // Speeed or times per second 00030 if(stepPin == 1){ 00031 stepPin = 0; 00032 wait(1/speed); 00033 } 00034 else{ 00035 stepPin = 1; 00036 wait(1/speed); 00037 } 00038 myled = stepPin; 00039 00040 00041 } 00042 00043 void DRV8825::enable() 00044 { 00045 en = 0; 00046 } 00047 00048 void DRV8825::disable() 00049 { 00050 en = 1; 00051 }
Generated on Thu Jul 14 2022 23:53:23 by
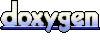