
IoT IR Distance Sensor Robot
Dependencies: Motordriver mbed
Fork of ECE4180Lab4 by
main.cpp
00001 #include "mbed.h" 00002 #include "motordriver.h" 00003 #include "Speaker.h" 00004 Motor A(p22, p6, p5, 1); // pwm, fwd, rev, can brake 00005 Motor B(p22, p6, p5, 1); 00006 Speaker mySpeaker(p21); 00007 Serial pc(USBTX, USBRX); 00008 Serial esp(p28, p27); // tx, rx 00009 00010 //DS18B20 thermom(A0, DS18B20::RES_12_BIT); 00011 00012 // Standard Mbed LED definitions 00013 DigitalOut led1(LED1); // (PTB18) 00014 DigitalOut led2(LED2); // (PTB19) 00015 DigitalOut led3(LED3); // (PTD1) 00016 00017 DigitalOut reset(p26); 00018 00019 AnalogIn motin(p16); 00020 00021 Timer t1; 00022 Timer t2; 00023 00024 struct tm t; 00025 00026 int bufflen, DataRX, count, getcount, replycount, servreq, timeout; 00027 int bufl, ipdLen, linkID, weberror, webcounter; 00028 float temperature, AdcIn, Ht; 00029 float R1=100000, R2=10000; // resistor values to give a 10:1 reduction of measured AnalogIn voltage 00030 char Vcc[10]; 00031 char Temp[10]; 00032 char temp[10]; 00033 char webcount[8]; 00034 char lasthit[30]; 00035 char timebuf[30]; 00036 char type[16]; 00037 char type1[16]; 00038 char channel[2]; 00039 char cmdbuff[32]; 00040 char replybuff[1024]; 00041 char webdata[1024]; // This may need to be bigger depending on WEB browser used 00042 char webbuff[4096]; // Currently using 1986 characters, Increase this if more web page data added 00043 00044 void SendCMD(),getreply(),ReadWebData(),startserver(),sendpage(),SendWEB(),sendcheck(); 00045 void gettime(),getmotor(),setRTC(),beep(); 00046 00047 // manual set RTC values 00048 int minute =00; // 0-59 00049 int hour =12; // 2-23 00050 int dayofmonth =26; // 1-31 00051 int month =8; // 1-12 00052 int year =15; // last 2 digits 00053 00054 int port =80; // set server port 00055 int SERVtimeout =5; // set server timeout in seconds in case link breaks. 00056 00057 // Serial Interrupt read ESP data 00058 void callback() 00059 { 00060 led3=1; 00061 while (esp.readable()) { 00062 webbuff[count] = esp.getc(); 00063 count++; 00064 } 00065 if(strlen(webbuff)>bufflen) { 00066 DataRX=1; 00067 led3=0; 00068 } 00069 } 00070 00071 // Static WEB page 00072 void sendpage() 00073 { 00074 getmotor(); 00075 gettime(); 00076 00077 // WEB page data 00078 strcpy(webbuff, "<!DOCTYPE html>"); 00079 strcat(webbuff, "<html><head><title>ESP8266 Mbed LPC1768</title></head>"); 00080 strcat(webbuff, "<body>"); 00081 strcat(webbuff, "<div style=\"text-align:center; background-color:#F4F4F4; color:#00AEDB;\"><h1>ESP8266 Mbed IoT Web Controller</h1>"); 00082 strcat(webbuff, "Hit Count - "); 00083 strcat(webbuff, webcount); 00084 strcat(webbuff, "<br>Last Hit - "); 00085 strcat(webbuff, lasthit); 00086 strcat(webbuff, "</div><br /><hr>"); 00087 strcat(webbuff, "<h3>Mbed RTC Time -  "); 00088 strcat(webbuff, timebuf); 00089 strcat(webbuff, "</h3>\r\n"); 00090 strcat(webbuff, "<p><form method=\"POST\"><strong> Analog 1:  <input type=\"text\" size=6 value=\""); 00091 strcat(webbuff, Temp); 00092 strcat(webbuff, "\"> </sup>V <form method=\"POST\"> <strong>   Analog 2:  <input type=\"text\" size=4 value=\""); 00093 strcat(webbuff, Vcc); 00094 strcat(webbuff, "\"> </sup>V"); 00095 if(led1==0) { 00096 strcat(webbuff, "<p><input type=\"radio\" name=\"led1\" value=\"0\" checked> LED 1 off"); 00097 strcat(webbuff, "<br><input type=\"radio\" name=\"led1\" value=\"1\" > LED 1 on"); 00098 } else { 00099 strcat(webbuff, "<p><input type=\"radio\" name=\"led1\" value=\"0\" > LED 1 off"); 00100 strcat(webbuff, "<br><input type=\"radio\" name=\"led1\" value=\"1\" checked> LED 1 on"); 00101 } 00102 strcat(webbuff, "</strong><p><input type=\"submit\" value=\"send-refresh\" style=\"background: #3498db;"); 00103 strcat(webbuff, "background-image:-webkit-linear-gradient(top, #3498db, #2980b9);"); 00104 strcat(webbuff, "background-image:linear-gradient(to bottom, #3498db, #2980b9);"); 00105 strcat(webbuff, "-webkit-border-radius:12;border-radius: 12px;font-family: Arial;color:#ffffff;font-size:20px;padding:"); 00106 strcat(webbuff, "10px 20px 10px 20px; border:solid #103c57 3px;text-decoration: none;"); 00107 strcat(webbuff, "background: #3cb0fd;"); 00108 strcat(webbuff, "background-image:-webkit-linear-gradient(top,#3cb0fd,#1a5f8a);"); 00109 strcat(webbuff, "background-image:linear-gradient(to bottom,#3cb0fd,#1a5f8a);"); 00110 strcat(webbuff, "text-decoration:none;\"></form></span>"); 00111 strcat(webbuff, "<p/><h2>How to use:</h2><ul>"); 00112 strcat(webbuff, "<li>Select the Radio buttons to control the digital out pins.</li>"); 00113 strcat(webbuff, "<li>Click 'Send-Refresh' to send.</li>"); 00114 strcat(webbuff, "<li>Use the 'Send-Refresh' button to refresh the data.</li>"); 00115 strcat(webbuff, "</ul>"); 00116 strcat(webbuff, "</body></html>"); 00117 // end of WEB page data 00118 bufl = strlen(webbuff); // get total page buffer length 00119 sprintf(cmdbuff,"AT+CIPSEND=%d,%d\r\n", linkID, bufl); // send IPD link channel and buffer character length. 00120 timeout=200; 00121 getcount=7; 00122 SendCMD(); 00123 getreply(); 00124 SendWEB(); // send web page 00125 memset(webbuff, '\0', sizeof(webbuff)); 00126 sendcheck(); 00127 } 00128 00129 // wait for ESP "SEND OK" reply, then close IP to load web page 00130 void sendcheck() 00131 { 00132 weberror=1; 00133 timeout=500; 00134 getcount=24; 00135 t2.reset(); 00136 t2.start(); 00137 while(weberror==1 && t2.read() <5) { 00138 getreply(); 00139 if (strstr(replybuff, "SEND OK") != NULL) { 00140 weberror=0; // wait for valid SEND OK 00141 } 00142 } 00143 if(weberror==1) { // restart connection 00144 strcpy(cmdbuff, "AT+CIPMUX=1\r\n"); 00145 timeout=500; 00146 getcount=10; 00147 SendCMD(); 00148 getreply(); 00149 pc.printf(replybuff); 00150 sprintf(cmdbuff,"AT+CIPSERVER=1,%d\r\n", port); 00151 timeout=500; 00152 getcount=10; 00153 SendCMD(); 00154 getreply(); 00155 pc.printf(replybuff); 00156 } else { 00157 sprintf(cmdbuff, "AT+CIPCLOSE=%s\r\n",channel); // close current connection 00158 SendCMD(); 00159 getreply(); 00160 pc.printf(replybuff); 00161 } 00162 t2.reset(); 00163 } 00164 00165 // Reads and processes GET and POST web data 00166 void ReadWebData() 00167 { 00168 wait_ms(200); 00169 esp.attach(NULL); 00170 count=0; 00171 DataRX=0; 00172 weberror=0; 00173 memset(webdata, '\0', sizeof(webdata)); 00174 int x = strcspn (webbuff,"+"); 00175 if(x) { 00176 strcpy(webdata, webbuff + x); 00177 weberror=0; 00178 int numMatched = sscanf(webdata,"+IPD,%d,%d:%s", &linkID, &ipdLen, type); 00179 if( strstr(webdata, "led1=1") != NULL ) { 00180 led1=1; 00181 } 00182 if( strstr(webdata, "led1=0") != NULL ) { 00183 led1=0; 00184 } 00185 sprintf(channel, "%d",linkID); 00186 if (strstr(webdata, "GET") != NULL) { 00187 servreq=1; 00188 } 00189 if (strstr(webdata, "POST") != NULL) { 00190 servreq=1; 00191 } 00192 webcounter++; 00193 sprintf(webcount, "%d",webcounter); 00194 } else { 00195 memset(webbuff, '\0', sizeof(webbuff)); 00196 esp.attach(&callback); 00197 weberror=1; 00198 } 00199 } 00200 // Starts and restarts webserver if errors detected. 00201 void startserver() 00202 { 00203 gettime(); 00204 pc.printf("\n\n RTC time %s\r\n\n",timebuf); 00205 pc.printf("++++++++++ Resetting ESP ++++++++++\r\n"); 00206 strcpy(cmdbuff,"AT+RST\r\n"); 00207 timeout=8000; 00208 getcount=1000; 00209 SendCMD(); 00210 getreply(); 00211 pc.printf(replybuff); 00212 pc.printf("%d",count); 00213 if (strstr(replybuff, "OK") != NULL) { 00214 pc.printf("\n++++++++++ Starting Server ++++++++++\r\n"); 00215 strcpy(cmdbuff, "AT+CIPMUX=1\r\n"); // set multiple connections. 00216 timeout=500; 00217 getcount=20; 00218 SendCMD(); 00219 getreply(); 00220 pc.printf(replybuff); 00221 sprintf(cmdbuff,"AT+CIPSERVER=1,%d\r\n", port); 00222 timeout=500; 00223 getcount=20; 00224 SendCMD(); 00225 getreply(); 00226 pc.printf(replybuff); 00227 wait(1); 00228 sprintf(cmdbuff,"AT+CIPSTO=%d\r\n",SERVtimeout); 00229 timeout=500; 00230 getcount=50; 00231 SendCMD(); 00232 getreply(); 00233 pc.printf(replybuff); 00234 wait(5); 00235 pc.printf("\n Getting Server IP \r\n"); 00236 strcpy(cmdbuff, "AT+CIFSR\r\n"); 00237 timeout=2500; 00238 getcount=200; 00239 while(weberror==0) { 00240 SendCMD(); 00241 getreply(); 00242 if (strstr(replybuff, "0.0.0.0") == NULL) { 00243 weberror=1; // wait for valid IP 00244 } 00245 } 00246 pc.printf("\n Enter WEB address (IP) found below in your browser \r\n\n"); 00247 pc.printf("\n The MAC address is also shown below,if it is needed \r\n\n"); 00248 replybuff[strlen(replybuff)-1] = '\0'; 00249 //char* IP = replybuff + 5; 00250 sprintf(webdata,"%s", replybuff); 00251 pc.printf(webdata); 00252 led2=1; 00253 bufflen=200; 00254 count=0; 00255 pc.printf("\n\n++++++++++ Ready ++++++++++\r\n\n"); 00256 esp.attach(&callback); 00257 } else { 00258 pc.printf("\n++++++++++ ESP8266 error, check power/connections ++++++++++\r\n"); 00259 while(1) {} 00260 } 00261 t2.reset(); 00262 t2.start(); 00263 beep(); 00264 } 00265 // ESP Command data send 00266 void SendCMD() 00267 { 00268 esp.printf("%s", cmdbuff); 00269 } 00270 // Large WEB buffer data send 00271 void SendWEB() 00272 { 00273 int i=0; 00274 if(esp.writeable()) { 00275 while(webbuff[i]!='\0') { 00276 esp.putc(webbuff[i]); 00277 i++; 00278 } 00279 } 00280 } 00281 // Get Command and ESP status replies 00282 void getreply() 00283 { 00284 memset(replybuff, '\0', sizeof(replybuff)); 00285 t1.reset(); 00286 t1.start(); 00287 replycount=0; 00288 while(t1.read_ms()< timeout && replycount < getcount) { 00289 if(esp.readable()) { 00290 replybuff[replycount] = esp.getc(); 00291 replycount++; 00292 } 00293 } 00294 t1.stop(); 00295 } 00296 // Analog in example 00297 void getmotor() 00298 { 00299 AdcIn=motin.read(); 00300 Ht = (AdcIn*3.3); // set the numeric to the exact MCU analog reference voltage for greater accuracy 00301 sprintf(Vcc,"%2.3f",Ht); 00302 } 00303 // Get RTC time 00304 void gettime() 00305 { 00306 time_t seconds = time(NULL); 00307 strftime(timebuf,50,"%H:%M:%S %a %d %b %y", localtime(&seconds)); 00308 } 00309 00310 void beep() 00311 { 00312 mySpeaker.PlayNote(2000, 0.1,0.1); 00313 } 00314 00315 void setRTC() 00316 { 00317 t.tm_sec = (0); // 0-59 00318 t.tm_min = (minute); // 0-59 00319 t.tm_hour = (hour); // 0-23 00320 t.tm_mday = (dayofmonth); // 1-31 00321 t.tm_mon = (month-1); // 0-11 "0" = Jan, -1 added for Mbed RCT clock format 00322 t.tm_year = ((year)+100); // year since 1900, current DCF year + 100 + 1900 = correct year 00323 set_time(mktime(&t)); // set RTC clock 00324 } 00325 00326 int main() { 00327 float x; 00328 reset=0; 00329 pc.baud(115200); 00330 00331 pc.printf("\f\n\r------------ ESP8266 Hardware Reset --------------\n\r"); 00332 wait(0.5); 00333 reset=1; 00334 led1=1,led2=0,led3=0; 00335 timeout=6000; 00336 getcount=500; 00337 getreply(); 00338 esp.baud(115200); // ESP8266 baudrate. Maximum on KLxx' is 115200, 230400 works on K20 and K22F 00339 if (time(NULL) < 1420070400) { 00340 setRTC(); 00341 } 00342 beep(); 00343 startserver(); 00344 00345 while(1) { 00346 if(DataRX==1) { //set up wifi stuff 00347 ReadWebData(); 00348 beep(); 00349 if (servreq == 1 && weberror == 0) { 00350 sendpage(); 00351 } 00352 esp.attach(&callback); //more wifi 00353 pc.printf(" IPD Data:\r\n\n Link ID = %d,\r\n IPD Header Length = %d \r\n IPD Type = %s\r\n", linkID, ipdLen, type); 00354 pc.printf("\n\n HTTP Packet: \n\n%s\n", webdata); 00355 pc.printf(" Web Characters sent : %d\n\n", bufl); 00356 pc.printf(" -------------------------------------\n\n"); //outputs for tera term 00357 strcpy(lasthit, timebuf); 00358 servreq=0; 00359 } 00360 x = motin; //set IR sensor reading to x 00361 A.speed(x); //set speed of motors 00362 B.speed(x); 00363 mySpeaker.PlayNote(motin*500, 0.1,0.1);//determine sound based on speed of motors 00364 } 00365 }
Generated on Sun Jul 17 2022 02:38:52 by
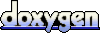