
ECE 4180 Laser Tag
Dependencies: Motordriver mbed SDFileSystem mbed-rtos wave_player
main.cpp
00001 #include "mbed.h" 00002 #include "motordriver.h" 00003 #include "SDFileSystem.h" 00004 #include "wave_player.h" 00005 #include "Speaker.h" 00006 #include "rtos.h" 00007 Motor m1(p22, p6, p5, 1); // pwm, fwd, rev, can brake left most motor (a) 00008 Motor m2(p23, p7, p8, 1); //right most motor (b) 00009 SDFileSystem sd(p11, p12, p13, p14, "sd"); // the pinout on the mbed Cool Components workshop board 00010 AnalogOut DACout(p18); //set up analog out 00011 wave_player waver(&DACout); //set up wave_player, with DACout as input to the funciton 00012 Serial blue(p9,p10); //used p13 and p14 before 00013 00014 //instantiate Ins and Outs 00015 InterruptIn detector(p25); 00016 DigitalOut led1(LED1); 00017 DigitalOut led2(LED2); 00018 DigitalOut led3(LED3); 00019 DigitalOut led4(LED4); 00020 DigitalOut laser(p20); 00021 DigitalIn shot(p26); 00022 00023 00024 volatile bool laserstate = false; 00025 volatile bool downstate = false; 00026 00027 void gothit_isr() { // runs when robot gets shot 00028 downstate = true; 00029 } 00030 //thread deals with shooting/movement (communication with bluetooth chip) 00031 void movement_thread(void const *args) { 00032 while(1) { 00033 char bnum=0; 00034 char bhit=0; 00035 if (blue.getc()=='!') { 00036 if (blue.getc()=='B') { //button data packet 00037 bnum = blue.getc(); //button number 00038 bhit = blue.getc(); //1=hit, 0=release 00039 if (blue.getc()==char(~('!' + 'B' + bnum + bhit))) { //checksum OK? 00040 switch (bnum) { 00041 case '1': //number button 1 00042 if (bhit=='1') { 00043 //led1 = 1; 00044 } else { 00045 //led1 = 0; 00046 } 00047 break; 00048 case '2': //number button 2 00049 if (bhit=='1') { 00050 // led2 = 1; 00051 } else { 00052 // led2 = 0; 00053 } 00054 break; 00055 case '3': //number button 3 00056 if (bhit=='1') { 00057 // led3 = 1; 00058 } else { 00059 // led3 = 0; 00060 } 00061 break; 00062 case '4': //number button 4 00063 if (bhit=='1') { 00064 // led4 = 1; 00065 } else { 00066 //led4 = 0; 00067 } 00068 break; 00069 case '5': //button 5 up arrow 00070 if (bhit=='1') { 00071 //move robot forward when up button is pressed 00072 m1.speed(0.95); 00073 m2.speed(0.95); 00074 } else { 00075 //stop robot when button is released 00076 m1.speed(0.0); 00077 m2.speed(0.0); 00078 } 00079 break; 00080 case '6': //button 6 down arrow 00081 if (bhit=='1') { 00082 //move robot backward when down button is pressed 00083 m1.speed(-0.95); 00084 m2.speed(-0.95); 00085 } else { 00086 //stop robot when button is released 00087 m1.speed(0.0); 00088 m2.speed(0.0); 00089 } 00090 break; 00091 case '7': //button 7 left arrow 00092 if (bhit=='1') { 00093 //move robot to the left by rotating both wheels when left button is pressed 00094 m1.speed(-0.75); 00095 m2.speed(0.75); 00096 } else { 00097 //stop robot when button is released 00098 m1.speed(0.0); 00099 m2.speed(0.0); 00100 } 00101 break; 00102 case '8': //button 8 right arrow 00103 if (bhit=='1') { 00104 //move robot to the right by rotating both wheels when right button is pressed 00105 m1.speed(0.75); 00106 m2.speed(-0.75); 00107 } else { 00108 //stop robot when button is released 00109 m1.speed(0.0); 00110 m2.speed(0.0); 00111 } 00112 break; 00113 default: 00114 break; 00115 } 00116 } 00117 } 00118 } 00119 Thread::yield(); 00120 } 00121 00122 } 00123 00124 int main() { 00125 // main() handles the shooting and getting shot 00126 Thread thread(movement_thread); 00127 detector.rise(&gothit_isr); // rising edge interrupt, used to see if robot got hit 00128 while(1) { 00129 if(shot ==1){ 00130 led4 =1; //turn led4 on if robot got shot 00131 } 00132 else{ 00133 led4 =0; 00134 } 00135 if (downstate) { // robot got hit 00136 led1 = 1; //turn led1 if robot gets hit 00137 Thread::wait(3000); // movement_thread loops meanwhile, allowing for robot to still move when shot 00138 laserstate = false; // resets laser so that laser cannot shoot 00139 downstate = false; //resets downstate so that robot can be hit again 00140 led1 =0; 00141 } 00142 if (laserstate) { // robot is shooting 00143 led2 = 1; //led2 turns on when shooting 00144 laser = 1; 00145 Thread::wait(250); // movement_thread loops meanwhile 00146 laserstate = false; 00147 laser = 0; 00148 led2 = 0; 00149 } 00150 Thread::yield(); // movement_thread runs once 00151 } 00152 }
Generated on Thu Jul 28 2022 01:18:50 by
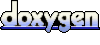