このライブラリはSW認識を行います。 SWは1個から20個まで認識できます。 SWの認識周期は10msです。 SWはmbedの端子とGNDの間に接続します。 This library does the recognition SW. SW, one can recognize up to 20. Recognition of the SW period is 10ms. SW is connected to GND terminal of mbed. ON the SW, OFF (level) data output. Output data from OFF to ON edge of the SW. Output data from ON to OFF edge of the SW. SWのON,OFF(レベル)データを出力します。 SWのOFFからONのエッジデータを出力します。 SWのONからOFFのエッジデータを出力します。
Dependents: kitchenTimer_Clock SwDigitalLibraryExampleProgram
SwDigital.h
00001 /* SwDigital Library 00002 * Copyright (c) 2011 suupen 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a copy 00005 * of this software and associated documentation files (the "Software"), to deal 00006 * in the Software without restriction, including without limitation the rights 00007 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 * copies of the Software, and to permit persons to whom the Software is 00009 * furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included in 00012 * all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00020 * THE SOFTWARE. 00021 */ 00022 00023 /***********************************************************************/ 00024 /* */ 00025 /* SwDigital.h */ 00026 /* */ 00027 /* */ 00028 /* V0.1 : 2011/11/13 */ 00029 /* @001 : 2011/12/11 example program bug fix */ 00030 /***********************************************************************/ 00031 #ifndef _SWDIGITAL_H 00032 #define _SWDIGITAL_H 00033 00034 #include "mbed.h" 00035 00036 /** SWDIGITAL control class, based on a "mbed function" 00037 * 00038 * Example: 00039 * @code 00040 * //============================================ 00041 * // SwDigital Library example program 00042 * // 00043 * // <schematic> 00044 * // 00045 * // mbed 00046 * // sw1 00047 * // ---- 00048 * // p20 --------o o----------- GND 00049 * // 00050 * // sw2 00051 * // ---- 00052 * // p21 --------o o------------GND 00053 * // 00054 * //============================================= 00055 * 00056 * 00057 * #include "mbed.h" 00058 * #include "SwDigital.h" 00059 * 00060 * SwDigital sw(p20,p21); // p20 : sw1 control LED1,LED2 00061 * // p21 : sw2 control LED3,LED4 00062 * 00063 * DigitalOut led1(LED1); 00064 * DigitalOut led2(LED2); 00065 * DigitalOut led3(LED3); 00066 * DigitalOut led4(LED4); 00067 * 00068 * int main() { 00069 * while(1) { 00070 * // sw level and edge data refresh 00071 * sw.refreshEdgeData(); //@001 00072 * 00073 * // tact action (sw level = on : led1 = on) 00074 * led1 = sw.checkLevel(0); 00075 * 00076 * // tact action (sw level = off : led2 = on) 00077 * led2 = !sw.checkLevel(0); 00078 * 00079 * // toggle action (level Off to On) 00080 * if(sw.checkEdgeOn(1) == 1){ 00081 * led3 = !led3; 00082 * } 00083 * 00084 * // toggle action (level On to Off) 00085 * if(sw.checkEdgeOff(1) == 1){ 00086 * led4 = !led4; 00087 * } 00088 * } 00089 * } 00090 * 00091 * @endcode 00092 */ 00093 00094 class SwDigital { 00095 public: 00096 00097 /** Create a sound object connected to the specified PwmOut pin & DigitalOut pin 00098 * 00099 * @param pin sw0 to sw19 : sw input pin 00100 * 00101 * sw can be set from 1 to 20. 00102 * Recognition of the SW period is 10ms 00103 */ 00104 SwDigital(PinName sw0 = NC, PinName sw1 = NC, PinName sw2 = NC, PinName sw3 = NC, PinName sw4 = NC, 00105 PinName sw5 = NC, PinName sw6 = NC, PinName sw7 = NC, PinName sw8 = NC, PinName sw9 = NC, 00106 PinName sw10 = NC, PinName sw11 = NC, PinName sw12 = NC, PinName sw13 = NC, PinName sw14 = NC, 00107 PinName sw15 = NC, PinName sw16 = NC, PinName sw17 = NC, PinName sw18 = NC, PinName sw19 = NC 00108 ); 00109 00110 /** refresh edge data 00111 * 00112 * @param none 00113 * @param return none 00114 * 00115 * main de edge data wo tukau maeni jiko suru 00116 */ 00117 void refreshEdgeData(void); 00118 00119 /** Check Off to On edge 00120 * 00121 * @param uint8_t swNo : check swNo 0 to 19 00122 * @param return uint8_t On edge check 0: edge Nasi 1: edge Ari 00123 * 00124 */ 00125 uint8_t checkEdgeOn(uint8_t swNo); 00126 00127 /** Check On to Off edge 00128 * 00129 * @param uint8_t swNo : check swNo 0 to 19 00130 * @param return uint8_t Off edge check 0 : Nasi 1 : Ari 00131 * 00132 */ 00133 uint8_t checkEdgeOff(uint8_t swNo); 00134 00135 /** Check On to Off edge 00136 * 00137 * @param uint8_t swNo : check swNo 0 to 19 00138 * @param return uint8_t sw level check 0 : Off 1 : On 00139 * 00140 */ 00141 uint8_t checkLevel(uint8_t swNo); 00142 00143 //protected: 00144 private: 00145 DigitalIn _sw0; 00146 DigitalIn _sw1; 00147 DigitalIn _sw2; 00148 DigitalIn _sw3; 00149 DigitalIn _sw4; 00150 00151 DigitalIn _sw5; 00152 DigitalIn _sw6; 00153 DigitalIn _sw7; 00154 DigitalIn _sw8; 00155 DigitalIn _sw9; 00156 00157 DigitalIn _sw10; 00158 DigitalIn _sw11; 00159 DigitalIn _sw12; 00160 DigitalIn _sw13; 00161 DigitalIn _sw14; 00162 00163 DigitalIn _sw15; 00164 DigitalIn _sw16; 00165 DigitalIn _sw17; 00166 DigitalIn _sw18; 00167 DigitalIn _sw19; 00168 00169 Ticker swCheckTimer; 00170 00171 void input(void); 00172 00173 #define Z_matchcycle (10000) // 10000[us](10[ms]) to 100000[us](100[ms]) 1[us]/count 00174 00175 uint8_t D_swSuu; // touroku sareta Sw Suu 1 to 20 00176 #define Z_swSuuMax (20) // SW no saidai suu 00177 00178 uint8_t B_kariLevel[Z_swSuuMax]; // kakutei mae no ninsiki Level 0bit:saisin(t) 1bit:t-1, ... ,7bit:t-7 0:Off 1:On 00179 // match number define 00180 //#define Z_itchiPattern (0x03) // 2kai itch 00181 #define Z_itchiPattern (0x07) // 3kai itchi 00182 //#define Z_itchiPattern (0x0f) // 4kai itchi 00183 //#define Z_itchiPattern (0x1f) // 5kai itchi 00184 //#define Z_itchiPattern (0x3f) // 6kai itchi 00185 //#define Z_itchiPattern (0x7f) // 7kai itchi 00186 //#define Z_itchiPattern (0xff) // 8kai itchi 00187 00188 // sw level data 00189 uint8_t D_nowLevel[Z_swSuuMax]; // saisin no kakutei Level 0:Off 1:On 00190 uint8_t D_oldLevel[Z_swSuuMax]; // zenkai no kakutei Level 0:Off 1:On 00191 #define Z_levelOff (0) 00192 #define Z_levelOn (1) 00193 00194 // sw edge data 00195 // swDigital.c naibu hensu 00196 uint8_t B_edgeOn[Z_swSuuMax]; // off kara on no ninsiki(on edge) 0:Nasi 1:Ari 00197 uint8_t B_edgeOff[Z_swSuuMax]; // on kara off no ninsiki(off edge) 0:Nasi 1:Ari 00198 // user use hensu 00199 uint8_t D_edgeOn[Z_swSuuMax]; // off kara on no ninsiki(on edge) 0:Nasi 1:Ari 00200 uint8_t D_edgeOff[Z_swSuuMax]; // on kara off no ninsiki(off edge) 0:Nasi 1:Ari 00201 #define Z_edgeNasi (0) 00202 #define Z_edgeAri (1) 00203 00204 00205 00206 00207 }; 00208 00209 #endif // _SWDIGITAL_H
Generated on Fri Jul 15 2022 22:51:18 by
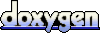