Embed:
(wiki syntax)
Show/hide line numbers
SoundSPI.h
00001 /* mbed Sound Library 00002 * Copyright (c) 2011 suupen 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a copy 00005 * of this software and associated documentation files (the "Software"), to deal 00006 * in the Software without restriction, including without limitation the rights 00007 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 * copies of the Software, and to permit persons to whom the Software is 00009 * furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included in 00012 * all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00020 * THE SOFTWARE. 00021 */ 00022 00023 /***********************************************************************/ 00024 /* */ 00025 /* Sound.h */ 00026 /* */ 00027 /* V0.1 : 2012/xx/xx */ 00028 /***********************************************************************/ 00029 #ifndef _SOUND_H 00030 #define _SOUND_H 00031 00032 #include <string> 00033 #include "types.h" 00034 00035 00036 /** Sound output control class, based on a PwmOut 00037 * 00038 * Example: 00039 * @code 00040 * // Output tone and merody 00041 * 00042 * #include "mbed.h" 00043 * #include "Sound.h" // sound library header 00044 * 00045 * Sound sound(p21, p10); // 1tu me no sound syuturyoku (pwmOut = p21, digitalOut = p10) 00046 * 00047 * 00048 * <scematic> 00049 * 00050 * -------- _/ 00051 * mbed(p21) -----|R:200ohm|----| | speaker 00052 * pwmOut -------- | | 00053 * | | 00054 * mbed(p10) -------------------|_| 00055 * digitalOut \ 00056 * 00057 * //-------------------------------- 00058 * // "westminster chime" merody data 00059 * //-------------------------------- 00060 * const Sound::sound_t WESTMINSTER[] = { 00061 * // hanon siji 0:b(flat) 1:tujo 2:#(sharp) 00062 * // | C1 - B9 kan deno onkai(Gx ha 9x ni okikae te siji) 0xFF=end data 00063 * // | | time (1/1[ms]/count) 00064 * // | | | envelope(yoin) (1/1 [ms]/count) 00065 * // | | | | 00066 * {1,0xA4,1200,1000}, 00067 * {1,0xF4,1200,1000}, 00068 * {1,0x94,1200,1000}, 00069 * {1,0xC4,2400,1000}, 00070 * 00071 * {1,0xC4,1200,1000}, 00072 * {1,0x94,1200,1000}, 00073 * {1,0xA4,1200,1000}, 00074 * {1,0xF4,2400,1000}, 00075 * 00076 * {1,0xFF,1000,0}, // end 00077 * }; 00078 * 00079 * /-------------------------------------- 00080 * * main 00081 * /--------------------------------------- 00082 * int main() { 00083 * 00084 * //--------------------- 00085 * // enso data no settei 00086 * //--------------------- 00087 * // sound.sound_enso("/local/enso.txt"); // mbed local file data "enso.txt" load (sita ni data no rei wo oite oku) 00088 * sound.sound_enso((Sound::sound_t*)WESTMINSTER); 00089 * 00090 * //--------------------------------------------------- 00091 * // output tone 00092 * //--------------------------------------------------- 00093 * // tone1 00094 * Sound::sound_t oto = {1,0x95,200,100}; 00095 * sound.sound_sound(oto); 00096 * while(sound.sound_sound() == true){} 00097 * 00098 * // tone2 00099 * oto.hanon = 1; oto.onkai = 0xA5; oto.time = 2000; oto.envelope = 1000; 00100 * sound.sound_sound(oto); 00101 * while(sound.sound_sound() == true){} 00102 * 00103 * //--------------- 00104 * // output merody 00105 * //-------------- 00106 * sound.sound_enso(true); 00107 * 00108 * while(1) { 00109 * } 00110 *} 00111 * 00112 * @endcode 00113 */ 00114 00115 00116 00117 00118 00119 00120 00121 00122 00123 00124 00125 00126 00127 00128 class Sound { 00129 public: 00130 00131 /** tone data struct 00132 * 00133 * @param uint8_t hanon : hanon siji 0:b(flat) 1:tujo 2:#(sharp) 00134 * @param uint16_t onkai : C1 - B9 kan deno onkai(Gx ha 9x ni okikae te siji) 0x00:hatuon teisi 0xFF:enso syuryo 00135 * @param uint16_t time : hatuon jikan (1/1 [ms]/count) 00136 * @param uint16_t envelope : yoin(envelope) jikan (1/1 [ms]/count) 00137 */ 00138 typedef struct { 00139 uint8_t hanon; // hanon siji 0:b(flat) 1:tujo 2:#(sharp) 00140 uint16_t onkai; // C1 - B9 kan deno onkai(Gx ha 9x ni okikae te siji) 00141 // 0x00:hatuon teisi 0xFF:enso syuryo 00142 uint16_t time; // hatuon jikan (1/1 [ms]/count) 00143 uint16_t envelope; // yoin(envelope) jikan (1/1 [ms]/count) 00144 } sound_t; 00145 00146 /* 00147 // hanon no menber 00148 enum{ 00149 Z_hanonF = 0, // b 00150 Z_hanonN , // tujo 00151 Z_hanonS, // # 00152 }; 00153 */ 00154 00155 /** Create a sound object connected to the specified PwmOut pin & DigitalOut pin 00156 * 00157 * @param pin PwmOut pin to connect to 00158 * @param pin DigitalOut pin to connect to 00159 */ 00160 Sound(PinName pwm , PinName kijun); 00161 00162 /** Check tone 00163 * 00164 * @param return A bool ture : output 00165 * \\false: none 00166 */ 00167 bool sound_sound(void); // oto no syuturyoku jotai wo kakunin 00168 // true : oto ari false : oto nasi 00169 /** output tone 00170 * 00171 * @param sound_t data : tone data set 00172 */ 00173 void sound_sound(sound_t data); // oto no syuturyoku 00174 // para : oto no data 00175 00176 /** merody data set (file data) 00177 * 00178 * @param merody data file path and name (example : "/local/merodyFileName.txt") 00179 */ 00180 bool sound_enso(char *path); // enso data wo file kara yomikomi 00181 // true : data kakunou OK false: data kakunou NG 00182 /** merody data set (data table) 00183 * 00184 * @param merody data table name (example : "(Sound::sound_t*)WESTMINSTER") 00185 */ 00186 void sound_enso(Sound::sound_t* onpudata); // enso data wo program no data table kara yomikomi 00187 00188 /** check merody 00189 * 00190 * @param raturn ture:merody output //false:merody stop 00191 */ 00192 bool sound_enso(void); // enso jyotai check 00193 // true : enso chu false : enso shuryo 00194 /** request merody start or stop 00195 * 00196 * @param ture:start merody //false:stop merody 00197 */ 00198 void sound_enso(bool siji); // enso start / stop 00199 // true : enso start false : enso stop 00200 00201 //protected: 00202 private: 00203 SPI _pwm; 00204 DigitalOut _kijun; 00205 00206 Ticker sound_timer; 00207 Timer hatuon_jikan; 00208 00209 00210 #define Z_pwmSyuuki (1) // PWM syuuki (1/1[us]/count) syokichi = 1 00211 #define Z_pwmBunkaino (16) // PWM bunkaino (1/1 [count]/count) 0:PWM=0% - 15:PWM=100% (SPI miso output bit(16bit) 00212 #define Z_pulseCheckSyuuki (20) // puls check syuuki (1/1 [us]/count) syokichi = 20 (10us ika deha pwm settei ga ijo ni naru) 00213 00214 00215 typedef struct{ 00216 uint32_t syuuki; // oto no syuuki no count suu wo kioku (1/1 [us]/count) 00217 uint32_t jikan; // oto no syuturyoku jikan wo kanri (1/1 [us]/count) 00218 uint32_t envelope; // envelope counter 0 - 1000000[us](1[s]) 00219 uint32_t shokichienvelope; // shokichi envelope counter 0 - 1000000[us](1[s]) 00220 } soundout_t; 00221 00222 00223 soundout_t O_sound; // oto no syuturyoku pwm data 00224 00225 sound_t enso[100]; // mbed drive no enso data kakuno yo 00226 sound_t *ensoDataTable; // enso data no sento address kioku 00227 00228 void sound_out(float siji, int8_t fugo); 00229 void pulseCheck(void); 00230 uint8_t F_pwmSet; // 0:zenhan hansyuuki 1:kohan hansyuuki wo request 00231 uint32_t C_syuukiKeika; 00232 uint8_t f_muonSet; // muon ji no sound_out() syori wo kurikaesu no wo fusegu 00233 uint32_t C_1msProcess; // 1ms syuuki syori counter (Z_pulseCheckSyuuki/1 [us]/count)) 00234 uint16_t D_SPIpwm; // SPI pwm no output data pwm 0 = 0x0000, pwm 1/15 = 0x0001, pwm 2/15 = 0x0003, ... , pwm 14/15 = 0x7FFF, pwm 15/15 = 0xFFFF 00235 00236 00237 void sound_ensoSyori(void); 00238 int keikajikan; // 1tu no oto no keikajikan 00239 sound_t* onpu; // onpu data no sento address 00240 00241 00242 }; 00243 00244 00245 #undef _SOUND_C 00246 #endif // _SOUND_H
Generated on Wed Jul 13 2022 08:40:22 by
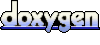