
This sample program is for Arduino TFT Screen module.
Fork of SPI18TFT by
main.cpp
00001 #include "stdio.h" 00002 #include "stdlib.h" 00003 #include "math.h" 00004 #include "mbed.h" 00005 #include "ST7735_TFT.h" 00006 #include "string" 00007 #include "Arial12x12.h" 00008 #include "Arial24x23.h" 00009 #include "Arial28x28.h" 00010 00011 #define NUMBER_OF_STARS 300 00012 #define SCREEN_WIDTH 128 00013 #define SCREEN_HEIGHT 160 00014 00015 /*star struct*/ 00016 typedef struct { 00017 float xpos, ypos; 00018 short zpos, speed; 00019 unsigned int color; 00020 } STAR; 00021 00022 static STAR stars[NUMBER_OF_STARS]; 00023 00024 00025 void init_star(STAR* star, int i) 00026 { 00027 /* randomly init stars, generate them around the center of the screen */ 00028 00029 star->xpos = -10.0 + (20.0 * (rand()/(RAND_MAX+1.0))); 00030 star->ypos = -10.0 + (20.0 * (rand()/(RAND_MAX+1.0))); 00031 00032 star->xpos *= 3072.0; /*change viewpoint */ 00033 star->ypos *= 3072.0; 00034 00035 star->zpos = i; 00036 star->speed = 2 + (int)(2.0 * (rand()/(RAND_MAX+1.0))); 00037 00038 star->color = i*Cyan >> 2; /*the closer to the viewer the brighter*/ 00039 } 00040 00041 00042 void init() 00043 { 00044 int i; 00045 00046 for (i = 0; i < NUMBER_OF_STARS; i++) { 00047 init_star(stars + i, i + 1); 00048 } 00049 } 00050 00051 00052 // the TFT is connected to SPI pin 5-7, CS is p8, RS is p11, reset is p15 00053 ST7735_TFT TFT(p5, p6, p7, p8, p11, p15,"TFT"); // mosi, miso, sclk, cs, rs, reset 00054 00055 Serial pc(USBTX, USBRX); // tx, rx 00056 Timer t; 00057 00058 extern unsigned char p1[]; // the mbed logo 00059 00060 int main() 00061 { 00062 00063 unsigned int centerx, centery; 00064 int i, j, tempx, tempy; 00065 init(); 00066 TFT.set_orientation(1); // original = 1 00067 centerx = TFT.width() >> 1; 00068 centery = TFT.height() >> 1; 00069 00070 00071 TFT.claim(stdout); // send stdout to the TFT display 00072 //TFT.claim(stderr); // send stderr to the TFT display 00073 00074 TFT.background(Black); // set background to black 00075 00076 TFT.foreground(White); // set chars to white 00077 00078 TFT.cls(); 00079 TFT.set_font((unsigned char*) Arial24x23); // select the font 00080 00081 t.start(); 00082 00083 00084 //↓@ss demo 00085 for( i=0; i < 1000; i++){ 00086 TFT.circle(rand()%TFT.width(), rand()%TFT.height(), rand()%50, rand()%0xffff); 00087 } 00088 00089 for( i=0; i < 10000; i++){ 00090 TFT.rect(rand()%TFT.width(), rand()%TFT.height(), rand()%TFT.width(), rand()%TFT.height(), rand()%0xffff); 00091 } 00092 00093 for( i=0; i < 1000; i++){ 00094 TFT.fillcircle(rand()%TFT.width(), rand()%TFT.height(), rand()%50, rand()%0xffff); 00095 00096 for( i=0; i < 1000; i++){ 00097 TFT.fillrect(rand()%TFT.width(), rand()%TFT.height(), rand()%TFT.width(), rand()%TFT.height(), rand()%0xffff); 00098 } 00099 00100 00101 } 00102 //↑@ss demo 00103 00104 00105 ////// demo start 00106 // *************************************************************** 00107 for( j = 0 ; j < 10000; j++) 00108 // for ( j = 0 ; j < 10000; j++ ) 00109 { 00110 00111 00112 // move and draw stars 00113 00114 for (i = 0; i < NUMBER_OF_STARS; i++) 00115 { 00116 tempx = (stars[i].xpos / stars[i].zpos) + centerx; 00117 tempy = (stars[i].ypos / stars[i].zpos) + centery; 00118 TFT.pixel(tempx,tempy,Black); 00119 00120 00121 stars[i].zpos -= stars[i].speed; 00122 00123 if (stars[i].zpos <= 0) 00124 { 00125 init_star(stars + i, i + 1); 00126 } 00127 00128 //compute 3D position 00129 tempx = (stars[i].xpos / stars[i].zpos) + centerx; 00130 tempy = (stars[i].ypos / stars[i].zpos) + centery; 00131 00132 if (tempx < 0 || tempx > TFT.width() - 1 || tempy < 0 || tempy > TFT.height() - 1) //check if a star leaves the screen 00133 { 00134 init_star(stars + i, i + 1); 00135 continue; 00136 } 00137 00138 TFT.pixel(tempx,tempy,stars[i].color); 00139 00140 } 00141 TFT.Bitmap(centerx-60,centery-19,120,38,p1); 00142 } 00143 00144 ///// demo stop 00145 00146 t.stop(); 00147 // *******************************************************************/ 00148 00149 00150 //↓@ss test 00151 #ifndef 1 00152 TFT.set_font((unsigned char*) Arial12x12); // select the font 00153 for(int x = 159; x >= 0; x--) { 00154 // TFT.pixel(-2,-1,0x100*x+0xff); 00155 TFT.rect(0, 0, x,(x-32), 0xffff); 00156 TFT.locate(0,10); 00157 printf("X=%d, y = %d",x,x-32); 00158 wait(0.1); 00159 TFT.rect(0, 0, x,(x-32), 0x0000); 00160 } 00161 #else 00162 // TFT.locate(0,10); 00163 TFT.set_font((unsigned char*) Arial12x12); // select the font 00164 // printf("Time %f s\n", t.read()); 00165 00166 // TFT.fillrect(-2, -1, 157, 126, 0); //clr 00167 00168 TFT.set_font((unsigned char*) Arial12x12); 00169 /* 00170 TFT.rect(0,0,159,127,0xffff); 00171 TFT.rect(10,10,20,20,0x0fff); 00172 TFT.rect(158,126,20,20,0xffff); 00173 TFT.line(0,0, 159,127,0xffff); 00174 TFT.line(159,0,0,127,0xffff); 00175 */ 00176 for(int y = 0; y < 128; y+=1) { 00177 for(int x = 0; x <160; x+=1) { 00178 if(y%2== 0) { 00179 if(x%2==0) { 00180 TFT.pixel(x,y,0xffff); 00181 } else { 00182 TFT.pixel(x,y,0x0ff); 00183 } 00184 } else { 00185 if(x%2==0) { 00186 TFT.pixel(x,y,0xff); 00187 } else { 00188 TFT.pixel(x,y,0xffff); 00189 } 00190 } 00191 //wait_ms(10); 00192 } 00193 } 00194 00195 #endif 00196 //↑@ss test 00197 00198 }
Generated on Wed Jul 13 2022 17:39:07 by
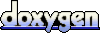