
Uses the Timeout library to create a periodic call to a function that blinks the LEDs.
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 00003 int rtCount; 00004 Timeout timeTick; 00005 DigitalOut led1(LED1); 00006 DigitalOut led2(LED2); 00007 00008 void flip_1() { 00009 led1 = !led1; 00010 } 00011 00012 void flip_2() { 00013 led2 = !led2; 00014 } 00015 00016 void action() { 00017 // Set timer to call back 00018 timeTick.attach(&action, 0.1); // setup flipper to call flip after 2 seconds 00019 rtCount++; // increment realtime counter 00020 if (rtCount == 5) { 00021 flip_1(); 00022 } else if (rtCount == 10) { 00023 flip_1(); 00024 flip_2(); 00025 rtCount = 0; 00026 } 00027 } 00028 00029 int main() { 00030 rtCount = 0; 00031 led1 = 1; 00032 led2 = 1; 00033 timeTick.attach(&action, 0.1); // setup flipper to call flip after 2 seconds 00034 00035 // spin in a main loop. flipper will interrupt it to call flip 00036 while (1) {} 00037 }
Generated on Wed Aug 3 2022 06:46:50 by
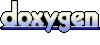