Simple FM Sound Synthesis with touch screen and accelerometer control, based on STM32F746G-DISCO
Dependencies: ADXL345 AUDIO_DISCO_F746NG BSP_DISCO_F746NG LCD_DISCO_F746NG SDRAM_DISCO_F746NG TS_DISCO_F746NG mbed-dev
Fork of Workshop_5 by
sinth.h
00001 00002 // Author: Stefano Fasciani - stefanofasciani@stefanofasciani.com 00003 // Date 7/8/2016 00004 // FM Sinth for DISCO_F746NG and ADXL345 00005 00006 00007 #include "mbed.h" 00008 #include "AUDIO_DISCO_F746NG.h" 00009 #include "SDRAM_DISCO_F746NG.h" 00010 #include "LCD_DISCO_F746NG.h" 00011 #include "TS_DISCO_F746NG.h" 00012 #include "ADXL345.h" 00013 #include <math.h> 00014 00015 #define AUDIO_BLOCK_SIZE 1024 00016 #define TABLE_SIZE 2048 00017 00018 typedef enum{ 00019 BUFFER_OFFSET_NONE = 0, 00020 BUFFER_OFFSET_HALF = 1, 00021 BUFFER_OFFSET_FULL = 2, 00022 }BUFFER_StateTypeDef; 00023 00024 float map(float x, float out_min, float out_max); 00025 void sint_osc(); 00026 void copy_buffer(int offset); 00027 void init_sin_table(); 00028 float get_touch_horizontal(); 00029 float get_touch_vertical(); 00030 float get_acc_horizontal(); 00031 float get_acc_vertical(); 00032 void display_keyboard(); 00033 float get_note_pitch(); 00034 uint8_t SetSysClock_PLL_HSE_200MHz(); 00035 00036 00037 extern float table[]; 00038 extern int16_t out_buffer[]; 00039 extern float osc_buf[]; 00040 extern __IO uint32_t audio_out_buffer_state; 00041 extern float idx_1; 00042 extern float idx_2; 00043 extern float filt_old_samp; 00044 extern float frqTL; 00045 extern float two_pi_48k; 00046 extern int acc_readings[]; 00047 extern int update_round; 00048 extern float amplitude; 00049 extern float pitch; 00050 extern float modrate; 00051 extern float moddepth; 00052 extern float filter; 00053 extern TS_StateTypeDef TS; 00054 extern LCD_DISCO_F746NG lcd;
Generated on Tue Jul 12 2022 16:59:03 by
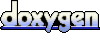