Simple FM Sound Synthesis with touch screen and accelerometer control, based on STM32F746G-DISCO
Dependencies: ADXL345 AUDIO_DISCO_F746NG BSP_DISCO_F746NG LCD_DISCO_F746NG SDRAM_DISCO_F746NG TS_DISCO_F746NG mbed-dev
Fork of Workshop_5 by
main.cpp
00001 // Author: Stefano Fasciani - stefanofasciani@stefanofasciani.com 00002 // Date 7/8/2016 00003 // FM Sinth for DISCO_F746NG and ADXL345 00004 00005 00006 #include "sinth.h" 00007 00008 ADXL345 accelerometer(D11,D12,D13,D10); 00009 TS_DISCO_F746NG ts; 00010 TS_StateTypeDef TS; 00011 AUDIO_DISCO_F746NG audio; 00012 SDRAM_DISCO_F746NG sdram; 00013 LCD_DISCO_F746NG lcd; 00014 Serial pc(USBTX, USBRX); 00015 00016 00017 //FM Synthesis Initial Parameters 00018 float amplitude = 0.5; //numbers between 0.0 and 1.0 00019 float pitch = 400; // numbers between 50 and 5000 00020 float modrate = 0; // numbers between -1000 and 1000 00021 float moddepth = 0; //numbers between -1000 and 1000 00022 float filter = 1.0f; //numbers between 0.0 (full filter) and 1.0 (do not filter) 00023 00024 00025 00026 void get_parameters(){ 00027 00028 char temp_string[20]; 00029 00030 //read input data 00031 ts.GetState(&TS); 00032 accelerometer.getOutput(acc_readings); 00033 00034 00035 if(TS.touchDetected){ 00036 //map to synthesis parameters 00037 amplitude = 0.5; //map(get_touch_vertical(),0.0,1.0); 00038 } 00039 else { 00040 amplitude = 0; 00041 } 00042 00043 00044 pitch = get_note_pitch(); //map(get_touch_horizontal(),50.0,5000.0); 00045 00046 modrate = map(get_acc_horizontal(), //input 00047 0, //modrate range minimum (not less than -1000) 00048 200.0); //modrate range maximum (not higher than 1000) 00049 00050 moddepth = map(get_acc_vertical(), //input 00051 -100.0, //moddepth range minimum (not less than -1000) 00052 100.0); //moddepth range maximum (not higher than 1000) 00053 00054 00055 00056 filter = map(get_touch_vertical(), //input 00057 0.0, //filter range minimum (not less than 0.0) 00058 0.7); //filter range maximum (not higher than 1.0) 00059 00060 00061 //update display 00062 switch(update_round){ 00063 case 0: 00064 sprintf(temp_string,"%f",amplitude); lcd.DisplayStringAt(250, LINE(1),(uint8_t *)temp_string, LEFT_MODE); 00065 case 1: 00066 sprintf(temp_string,"%f",pitch); lcd.DisplayStringAt(250, LINE(3),(uint8_t *)temp_string, LEFT_MODE); 00067 case 2: 00068 sprintf(temp_string,"%f",modrate); lcd.DisplayStringAt(250, LINE(5),(uint8_t *)temp_string, LEFT_MODE); 00069 case 3: 00070 sprintf(temp_string,"%f",moddepth); lcd.DisplayStringAt(250, LINE(7),(uint8_t *)temp_string, LEFT_MODE); 00071 case 4: 00072 sprintf(temp_string,"%f",filter); lcd.DisplayStringAt(250, LINE(9),(uint8_t *)temp_string, LEFT_MODE); 00073 } 00074 update_round++; 00075 if(update_round>4)update_round=0; 00076 00077 return; 00078 } 00079 00080 00081 00082 int main() 00083 { 00084 00085 //initialize touch screen 00086 ts.Init(lcd.GetXSize(), lcd.GetYSize()); 00087 wait(0.2); 00088 00089 // lcd initialization to display synthesis parameter 00090 lcd.Clear(LCD_COLOR_BLACK); 00091 display_keyboard(); 00092 lcd.SetBackColor(LCD_COLOR_BLACK); 00093 lcd.SetTextColor(LCD_COLOR_LIGHTBLUE); 00094 lcd.DisplayStringAt(10, LINE(1),(uint8_t *)"AMPLITUDE :", LEFT_MODE); 00095 lcd.DisplayStringAt(10, LINE(3),(uint8_t *)"PITCH (Hz) :", LEFT_MODE); 00096 lcd.DisplayStringAt(10, LINE(5),(uint8_t *)"MOD.RATE (Hz):", LEFT_MODE); 00097 lcd.DisplayStringAt(10, LINE(7),(uint8_t *)"MOD.DEPTH :", LEFT_MODE); 00098 lcd.DisplayStringAt(10, LINE(9),(uint8_t *)"FILTER :", LEFT_MODE); 00099 00100 wait(0.2); 00101 00102 00103 //initialize accelerometer 00104 accelerometer.getDevId(); 00105 accelerometer.setPowerControl(0x00); 00106 accelerometer.setDataFormatControl(0x0B); 00107 accelerometer.setDataRate(ADXL345_3200HZ); 00108 accelerometer.setPowerControl(0x08); 00109 wait(0.2); 00110 00111 //sound synthesis initialization 00112 init_sin_table(); 00113 SetSysClock_PLL_HSE_200MHz(); 00114 pc.baud(9600); 00115 memset((void *)out_buffer, 0, AUDIO_BLOCK_SIZE*2*sizeof(uint16_t)); 00116 audio_out_buffer_state = BUFFER_OFFSET_NONE; 00117 audio.OUT_SetAudioFrameSlot(CODEC_AUDIOFRAME_SLOT_02); 00118 audio.OUT_Play((uint16_t*)out_buffer, AUDIO_BLOCK_SIZE * 2 * sizeof(int16_t)); 00119 00120 00121 while (1) { 00122 00123 while(audio_out_buffer_state != BUFFER_OFFSET_HALF); 00124 audio_out_buffer_state = BUFFER_OFFSET_NONE; 00125 get_parameters(); 00126 sint_osc(); 00127 copy_buffer(0); 00128 00129 00130 while(audio_out_buffer_state != BUFFER_OFFSET_FULL); 00131 audio_out_buffer_state = BUFFER_OFFSET_NONE; 00132 get_parameters(); 00133 sint_osc(); 00134 copy_buffer(AUDIO_BLOCK_SIZE); 00135 00136 } 00137 } 00138 00139 /* 00140 CONNECTION DISCO_F746NG to ADXL345 00141 D10 - SS - CS 00142 D11 - MOSI - SDA 00143 D12 - MISO - SDO 00144 D13 - SCK - SCL 00145 3V3 - VCC - VCC 00146 GND - GND - GND 00147 */ 00148 00149 00150 00151 00152 00153
Generated on Tue Jul 12 2022 16:59:03 by
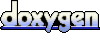