
Simple midi controller for Freedom KL25 board which generates tone (midi signal) according X-axis accelerometer. Modulation wheel is controlled by Y-axis and pitch wheel is controlled by touch slider
Dependencies: MMA8451Q TSI USBDevice mbed
Fork of Midiudelator by
main.cpp
00001 #include "mbed.h" 00002 #include "MMA8451Q.h" 00003 #include "USBMIDI.h" 00004 #include "TSISensor.h" 00005 00006 #define MMA8451_I2C_ADDRESS (0x1d<<1) 00007 00008 #define C1 0,187 00009 #define D1 0.250 00010 #define E1 0.312 00011 #define F1 0.375 00012 #define G1 0.437 00013 #define A1 0.5 00014 #define B1 0.562 00015 #define C2 0.625 00016 #define D2 0.687 00017 #define E2 0.750 00018 #define F2 0.812 00019 #define G2 0.875 00020 #define A2 0.937 00021 #define B2 1 00022 #define C3 1.062 00023 #define D3 1.125 00024 #define E3 1,187 00025 #define F3 1.250 00026 #define G3 1.312 00027 #define A3 1.375 00028 #define B3 1.437 00029 #define C4 1.5 00030 #define D4 1.562 00031 #define E4 1.625 00032 #define F4 1.687 00033 #define G4 1.749 00034 #define A4 1.811 00035 #define B4 1.873 00036 00037 //1.935 00038 00039 00040 00041 #define NC1 48 00042 #define ND1 50 00043 #define NE1 52 00044 #define NF1 53 00045 #define NG1 55 00046 #define NA1 57 00047 #define NB1 59 00048 #define NC2 60 00049 #define ND2 62 00050 #define NE2 64 00051 #define NF2 65 00052 #define NG2 67 00053 #define NA2 69 00054 #define NB2 71 00055 #define NC3 72 00056 #define ND3 74 00057 #define NE3 76 00058 #define NF3 77 00059 #define NG3 79 00060 #define NA3 81 00061 #define NB3 83 00062 #define NC4 84 00063 #define ND4 86 00064 #define NE4 88 00065 #define NF4 89 00066 #define NG4 91 00067 #define NA4 93 00068 #define NB4 95 00069 00070 Serial pc(USBTX,USBRX); 00071 USBMIDI midi; 00072 TSISensor tsi; 00073 00074 00075 void show_message(MIDIMessage msg) { 00076 switch (msg.type()) { 00077 case MIDIMessage::NoteOnType: 00078 printf("NoteOn key:%d, velocity: %d, channel: %d\n", msg.key(), msg.velocity(), msg.channel()); 00079 break; 00080 case MIDIMessage::NoteOffType: 00081 printf("NoteOff key:%d, velocity: %d, channel: %d\n", msg.key(), msg.velocity(), msg.channel()); 00082 break; 00083 case MIDIMessage::ControlChangeType: 00084 printf("ControlChange controller: %d, data: %d\n", msg.controller(), msg.value()); 00085 break; 00086 case MIDIMessage::PitchWheelType: 00087 printf("PitchWheel channel: %d, pitch: %d\n", msg.channel(), msg.pitch()); 00088 break; 00089 default: 00090 printf("Another message\n"); 00091 } 00092 } 00093 00094 00095 00096 int main() { 00097 00098 int note=48, n=48; 00099 float notechng; 00100 int pitch; 00101 int modulation; 00102 float m; 00103 00104 MMA8451Q acc(PTE25, PTE24, MMA8451_I2C_ADDRESS); 00105 00106 midi.attach(show_message); // call back for messages received 00107 00108 while (1) { 00109 #if 0 00110 /* TEST CODE */ 00111 /* test pitch - commented in in - endif*/ 00112 midi.write(MIDIMessage::NoteOn(57)); 00113 for(int c=0; c<127; c= c+1){ 00114 00115 midi.write(MIDIMessage::ControlChange(1, c)); 00116 //midi.write(MIDIMessage::PitchWheel(c)); 00117 pc.printf("Pitch: %d \n", c); 00118 } 00119 midi.write(MIDIMessage::NoteOff(57)); 00120 wait(0.5); 00121 #endif 00122 #if 1 00123 /* read note */ 00124 notechng = 1 - acc.getAccX(); 00125 00126 /* get modulatio */ 00127 m = ((1 + acc.getAccY()) * 63); 00128 modulation = int(m); 00129 00130 /* get pitch */ 00131 pitch = (int(tsi.readPercentage() * 8192) - 8192); 00132 00133 if(notechng > C1) note= NC1; 00134 if(notechng > D1) note= ND1; 00135 if(notechng > E1) note= NE1; 00136 if(notechng > F1) note= NF1; 00137 if(notechng > G1) note= NG1; 00138 if(notechng > A1) note= NA1; 00139 if(notechng > B1) note= NB1; 00140 if(notechng > C2) note= NC2; 00141 if(notechng > D2) note= ND2; 00142 if(notechng > E2) note= NE2; 00143 if(notechng > F2) note= NF2; 00144 if(notechng > G2) note= NG2; 00145 if(notechng > A2) note= NA2; 00146 if(notechng > B2) note= NB2; 00147 if(notechng > C3) note= NC3; 00148 if(notechng > D3) note= ND3; 00149 // if(notechng > E3) note= NE3; 00150 if(notechng > F3) note= NF3; 00151 if(notechng > G3) note= NG3; 00152 if(notechng > A3) note= NA3; 00153 if(notechng > B3) note= NB3; 00154 if(notechng > C4) note= NC4; 00155 if(notechng > D4) note= ND4; 00156 if(notechng > E4) note= NE4; 00157 if(notechng > F4) note= NF4; 00158 if(notechng > G4) note= NG4; 00159 if(notechng > A4) note= NA4; 00160 if(notechng > B4) note= NB4; 00161 00162 pc.printf("note: %d \n", note); 00163 pc.printf("notechng: %f \n", notechng); 00164 00165 if (note != n) { 00166 midi.write(MIDIMessage::NoteOff(n)); 00167 n=note; 00168 midi.write(MIDIMessage::NoteOn(n)); 00169 } 00170 00171 midi.write(MIDIMessage::ControlChange(1, modulation)); 00172 midi.write(MIDIMessage::PitchWheel(pitch)); 00173 00174 wait(0.1); 00175 #endif 00176 } 00177 }
Generated on Tue Jul 19 2022 06:41:41 by
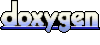