A simple Oscilloscope that log each pin's data change Please dump log and analyze using Excel x-y chart type!
Scope.h
00001 /* 00002 * BreathLed.h 00003 * 00004 * Created on: 2015/4/6 00005 * Author: steeven@gmail.com 00006 */ 00007 00008 #ifndef SCOPE_H_ 00009 #define SCOPE_H_ 00010 00011 #include "mbed.h" 00012 #include "BufferedSerial/BufferedSerial.h" 00013 00014 #define SCOPE_PINS 16 00015 00016 namespace steeven { 00017 00018 /** A simple Oscilloscope that log each pin's data change 00019 * Please dump log and analyze using excel x-y chart type! 00020 */ 00021 00022 typedef struct { 00023 char id; //bit 7: val, bit 0-6:id 00024 float time; 00025 } ScopeData; 00026 00027 class Scope { 00028 public: 00029 Scope(int buf_len); 00030 00031 void add(PinName pin, const char *name); 00032 00033 void log(char id, int v); 00034 00035 void dump( BufferedSerial *logger); 00036 00037 protected: 00038 const char *_names[SCOPE_PINS]; 00039 int _cnt; 00040 00041 Timer _timer; 00042 00043 ScopeData *_buf; 00044 int _buf_len; 00045 int _buf_cnt; 00046 }; 00047 00048 class ScopePin { 00049 00050 public: 00051 ScopePin(PinName pin, char id, Scope *scope); 00052 00053 protected: 00054 void on_fall(); 00055 void on_rise(); 00056 00057 InterruptIn _pin; 00058 char _id; 00059 Scope *_scope; 00060 }; 00061 00062 } 00063 ; 00064 00065 #endif
Generated on Wed Jul 27 2022 10:47:02 by
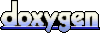