A simple Oscilloscope that log each pin's data change Please dump log and analyze using Excel x-y chart type!
Scope.cpp
00001 #include "Scope.h" 00002 00003 namespace steeven { 00004 00005 ScopePin::ScopePin(PinName pin, char id, Scope *scope) : 00006 _pin(pin), _id(id), _scope(scope) { 00007 _pin.fall(this, &ScopePin::on_fall); 00008 _pin.rise(this, &ScopePin::on_rise); 00009 } 00010 00011 void ScopePin::on_fall() { 00012 _scope->log(_id, 0); 00013 } 00014 void ScopePin::on_rise() { 00015 _scope->log(_id, 1); 00016 } 00017 00018 Scope::Scope(int buf_len) : 00019 _buf_len(buf_len) { 00020 _cnt = 0; 00021 _buf_cnt = 0; 00022 _buf = new ScopeData[buf_len]; 00023 _timer.start(); 00024 } 00025 00026 void Scope::add(PinName pin, const char *name) { 00027 new ScopePin(pin, _cnt, this); 00028 _names[_cnt] = name; 00029 // printf("%s ", _names[_cnt]); 00030 _cnt++; 00031 } 00032 00033 void Scope::log(char id, int v) { 00034 if (_buf_cnt >= _buf_len) 00035 error("Scope buffer overflow!\n"); 00036 _buf[_buf_cnt].id = (char) (v << 7) + id; 00037 _buf[_buf_cnt].time = _timer.read(); 00038 _buf_cnt++; 00039 } 00040 00041 void Scope::dump(BufferedSerial *logger) { 00042 int i, j, k; 00043 00044 logger->putc('\n'); 00045 00046 // channel names; 00047 logger->puts("time\t"); 00048 for (i = 0; i < _cnt; ++i) { 00049 logger->puts(_names[i]); 00050 logger->putc('\t'); 00051 } 00052 logger->putc('\n'); 00053 00054 // channel data 00055 for (j = 0; j < _cnt; ++j) { // buy channel 00056 for (i = 0; i < _buf_cnt; ++i) { // find in all data 00057 if ((_buf[i].id & 0x7f) == j) { // if this pin 00058 logger->printf("%f\t", _buf[i].time); 00059 for (k = 0; k < j; ++k) 00060 logger->putc('\t'); 00061 logger->printf("%d", ((_buf[i].id & 0x80) > 0 ? 1 : 0) + j * 2); // add offset 00062 logger->putc('\n'); 00063 } 00064 } 00065 } 00066 00067 //clear data 00068 _buf_cnt = 0; 00069 } 00070 }
Generated on Wed Jul 27 2022 10:47:02 by
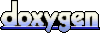