Tuned for OS2 to reduce flash code size for smaller MCU's. Added further functions since 2018 v1.7 firmware. Improved faster large web page sending. Built in fast NTP Client time and RTC setting function. ATParser message handling improvements and updated.
ESP8266.h
00001 00002 #ifndef ESP8266_H 00003 #define ESP8266_H 00004 00005 #include "ATParser.h" 00006 00007 00008 class ESP8266 00009 { 00010 public: 00011 ESP8266(PinName tx, PinName rx, bool debug=false); 00012 00013 /** 00014 * Startup the ESP8266 00015 * 00016 * @param mode of WIFI 1-client, 2-host, 3-both 00017 * @return true only if ESP8266 was setup correctly 00018 */ 00019 bool startup(int mode); 00020 00021 /** 00022 * Start the ESP8266 in server mode 00023 * 00024 * @param mode of WIFI 1-client, 2-host, 3-both 00025 * @param port 00026 * @param timeout 00027 * @return true only if ESP8266 was setup correctly 00028 */ 00029 bool startServer(int mode,int port,int timeout); 00030 00031 /** 00032 * Reset ESP8266 00033 * 00034 * @return true only if ESP8266 resets successfully 00035 */ 00036 bool reset(void); 00037 00038 /** 00039 * Get ESP8266 firmware version. 00040 * 00041 * @return firmware version. 00042 */ 00043 const char *getFirmware(void); 00044 00045 /** 00046 * Enable/Disable DHCP 00047 * 00048 * @param enabled DHCP enabled when true 00049 * @param mode mode of DHCP 0-softAP, 1-station, 2-both 00050 * @return true only if ESP8266 enables/disables DHCP successfully 00051 */ 00052 00053 bool dhcp(bool enabled, int mode); 00054 00055 /** 00056 * Connect ESP8266 to AP 00057 * 00058 * @param ap the name of the AP 00059 * @param passPhrase the password of AP 00060 * @return true only if ESP8266 is connected successfully 00061 */ 00062 bool connect(const char *ap, const char *passPhrase); 00063 00064 /** 00065 * Disconnect ESP8266 from AP 00066 * 00067 * @return true only if ESP8266 is disconnected successfully 00068 */ 00069 bool disconnect(void); 00070 00071 /** 00072 * Get the IP address of ESP8266 00073 * 00074 * @return null-teriminated IP address or null if no IP address is assigned 00075 */ 00076 const char *getIPAddress(void); 00077 00078 /** 00079 * Get the MAC address of ESP8266 00080 * 00081 * @return null-terminated MAC address or null if no MAC address is assigned 00082 */ 00083 const char *getMACAddress(void); 00084 00085 /** 00086 * Check if ESP8266 is conenected 00087 * 00088 * @return true only if the chip has an IP address 00089 */ 00090 bool isConnected(void); 00091 00092 /** 00093 * Open a socketed connection 00094 * 00095 * @param type the type of socket to open "UDP" or "TCP" 00096 * @param id id to give the new socket, valid 0-4 00097 * @param port port to open connection with 00098 * @param addr the IP address of the destination 00099 * @return true only if socket opened successfully 00100 */ 00101 bool open(const char *type, int id, const char* addr, int port); 00102 00103 /** 00104 * Sends data to an open socket 00105 * 00106 * @param id id of socket to send to 00107 * @param data data to be sent 00108 * @param amount amount of data to be sent - max 2048 00109 * @return true only if data sent successfully 00110 */ 00111 bool send(int id, const void *data, uint32_t amount); 00112 00113 /** 00114 * Sends larger web page data to a connection 00115 * more controlled with comms failure recovery 00116 * 00117 * @param id id of socket to send to 00118 * @param data web page data to be sent 00119 * @param amount amount of data to be sent. 00120 * No theoretical limit, use for large data pages. 00121 * Sends in 2048kbit chunks. 00122 * @return true only if data sent successfully 00123 */ 00124 bool sendWebPage(int id, const char* page, uint32_t amount); 00125 00126 /** 00127 * Receives data from an open socket 00128 * 00129 * @param id id to receive from 00130 * @param data placeholder for returned information 00131 * @param amount number of bytes to be received 00132 * @return the number of bytes received 00133 */ 00134 int32_t recv(int id, void *data, uint32_t amount); 00135 00136 /** 00137 * Receives data from an open web socket 00138 * 00139 * @param id id to receive from 00140 * @param data placed in 4 containers 00141 * @param char requestType; 00142 * @param char request; 00143 * @param int linkId; 00144 * @param int ipdLen; 00145 * @return the number of bytes received 00146 */ 00147 const char *recvWebRequest(void); 00148 00149 /** 00150 * Closes a socket 00151 * 00152 * @param id id of socket to close, valid only 0-4 00153 * @return true only if socket is closed successfully 00154 */ 00155 bool close(int id); 00156 00157 /* Return RSSI for active connection 00158 * 00159 * @return Measured RSSI 00160 */ 00161 int8_t getRSSI(); 00162 00163 /** 00164 * Gets NTP time in seconds since 1970. 00165 * 00166 * @param NTPpool url for server e.g. "1.nl.pool.ntp.org" 00167 * @param tzoffset +/- seconds offset for required time zone e.g. 3600 to add one hour 00168 * @param setRTC If true, Set's MCU internal RTC to the received seconds since 1970 including offset 00169 * @return seconds since 1970 00170 */ 00171 int32_t getNTP(char * NTPpool, int tzoffset, int setRTC); 00172 00173 00174 /** 00175 * Allows timeout to be changed between commands 00176 * 00177 * @param timeout_ms timeout of the connection 00178 */ 00179 void setTimeout(uint32_t timeout_ms); 00180 00181 /** 00182 * Checks if data is available 00183 */ 00184 bool readable(); 00185 00186 /** 00187 * Checks if data can be written 00188 */ 00189 bool writeable(); 00190 00191 char requestType[6]; 00192 char request[50]; 00193 int linkId; 00194 int ipdLen; 00195 int _con_status; 00196 char _ssid[32]; 00197 char _APIP_buffer[16]; 00198 char _APMAC_buffer[18]; 00199 char _STAIP_buffer[16]; 00200 char _STAMAC_buffer[18]; 00201 char _firmware[200]; 00202 00203 00204 private: 00205 BufferedSerial _serial; 00206 ATParser _parser; 00207 Timer t; 00208 }; 00209 00210 #endif
Generated on Tue Jul 19 2022 11:38:05 by
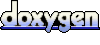