
DS1820, DS18B20 single and multi sensor OS6 compliant. Based on Erik's original code. Tested on ST's F767 and H743 platforms. Using single and multiple sensors up to 15 meters of cable length.
main.cpp
00001 #include "mbed.h" 00002 #include "DS1820.h" 00003 00004 DigitalOut led(LED1); 00005 00006 #define DATA_PIN A0 00007 #define MAX_PROBES 16 00008 DS1820* probe[MAX_PROBES]; 00009 00010 Timer t; 00011 00012 int DS; 00013 float Temp[16]; 00014 00015 int main(){ 00016 00017 printf("\033[0m\033[2J\033[HInitialise...!\n\n"); 00018 00019 while (DS1820::unassignedProbe(DATA_PIN)) { 00020 probe[DS] = new DS1820(DATA_PIN); 00021 DS++; 00022 if (DS == MAX_PROBES) { 00023 break; 00024 } 00025 } 00026 00027 if (!DS) { 00028 printf("No Sensors found!\n\n"); 00029 ThisThread::sleep_for(chrono::milliseconds(1000)); 00030 NVIC_SystemReset(); 00031 } 00032 00033 // set each probe resolution, default is 12bit (750ms) 00034 probe[0]->setResolution(9); 00035 // probe[0]->setResolution(10); 00036 // probe[0]->setResolution(11); 00037 // probe[0]->setResolution(12); 00038 // probe[1]->setResolution(9); 00039 // probe[2]->setResolution(10); 00040 00041 t.start(); 00042 00043 while(1) { 00044 00045 printf("\033[0m\033[2J\033[HDS Sensor data..\n\n"); 00046 00047 int DS_error = 0; 00048 for (int i = 0; i < DS; i++) { 00049 Temp[i] = probe[i]->temperature(); 00050 if(Temp[i]==-1000) { 00051 Temp[i] = probe[i]->temperature(); // get read temp again if error 00052 DS_error++; 00053 } 00054 printf("Probe %d: %3.2f %cc\r\n",i,Temp[i],0xb0); 00055 } 00056 printf("\nDS errors: %d\n\n", DS_error); 00057 00058 printf("Start conversion\n"); 00059 t.reset(); 00060 // don't wait for conversion, but do something that takes at least 750ms before reading the sensors 00061 //if(DS>0){probe[0]->convertTemperature(0, DS1820::all_devices);} 00062 00063 // wait for conversion, can take up to 750ms(12 bit mode) 00064 if(DS>0){probe[0]->convertTemperature(1, DS1820::all_devices);} 00065 00066 printf("\nConvert process time: %0.6f Seconds\n", chrono::duration<float>(t.elapsed_time()).count()); 00067 00068 ThisThread::sleep_for(chrono::milliseconds(1000)); 00069 } 00070 }
Generated on Thu Jul 28 2022 11:04:48 by
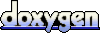