
Module 1 ECEN 5803 Project 1 Submitted by 1. Rahul Yamasani 2. Srivishnuj Alvakonda
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 Serial pc(USBTX, USBRX); //serial channel over HDK USB interface 00003 Timer timer; 00004 /************************************************************************//** 00005 * \file main.c 00006 * \brief LAB EXERCISE 5.2 - SQUARE ROOT APPROXIMATION 00007 * 00008 * Write an assembly code subroutine to approximate the square root of an 00009 * argument using the bisection method. All math is done with integers, so the 00010 * resulting square root will also be an integer 00011 ****************************************************************************** 00012 * GOOD LUCK! 00013 ****************************************************************************/ 00014 00015 #include "stdint.h" 00016 00017 00018 /** @cond DO_NOT_INCLUDE_WITH_DOXYGEN */ 00019 #include "string.h" 00020 /** @endcond */ 00021 00022 /** 00023 * @brief 5863 Write a brief description of the function here 00024 * 00025 * Detailed description of the function after one line gap 00026 * 00027 * @note You can also add a note this way 00028 * 00029 * @param[in] 00030 * You can also start writing on a new line. 00031 * And continue on the next line. This is how you describe an input parameter 00032 * 00033 * @return 00034 * This is how you describe the return value 00035 * 00036 */ 00037 00038 __asm int my_sqrt(int x){ 00039 00040 00041 PUSH {R4-R7} ; 00042 LDR R1, =0 ;done = 0 00043 LDR R7, =0 ;a=0 00044 LDR R2, =0xFFFF ;b=2^31 -1 00045 LDR R3, =0xFFFFFFFF ;c=-1 00046 DO_WHILE 00047 MOV R4, R3 ;c_old = c HERE R4 is being considered as c_old 00048 ADDS R5, R2, R7 ;R5 = a+b 00049 ASRS R5, R5, #1 ;R5 >> 1 which is nothing but (a+b/2) 00050 MOV R3, R5 ;c = (a+b)/2 00051 MOV R6,R3 00052 MULS R6, R3, R6 ;R6 = c*c 00053 CMP R6,R0 ;compare c*c and x (input integer) 00054 BEQ DONE 00055 BLT LESS_THAN 00056 MOV R2, R3 ;setting b=c 00057 B FINAL 00058 00059 LESS_THAN 00060 MOV R7,R3 ;setting a=c 00061 B FINAL 00062 00063 00064 DONE 00065 LDR R1, =1 ;setting done = 1 00066 B FINAL ;return C 00067 00068 00069 FINAL 00070 CMP R1, #1 ;compare done = 1 00071 BEQ END 00072 BNE COMPARE 00073 00074 00075 COMPARE 00076 CMP R4, R3 ;compare c_old and c 00077 BNE DO_WHILE 00078 BEQ END 00079 00080 00081 END 00082 MOV R0, R3 00083 POP {R4-R7} 00084 BX LR 00085 } 00086 00087 void printCpuCycles(double readValue, int number, int sqrt) 00088 { 00089 double cpuCycles = 0; 00090 00091 cpuCycles = (readValue * 48000000); // read value * frequqncy of clock 00092 pc.printf("Sqrt of %d using bisection menthod is %d \n\r", number, sqrt); 00093 pc.printf("Total time of execution for sqrt of(%d)is %f \n\r", number, readValue); 00094 pc.printf("Number of CPU cycles for sqrt(%d): %d\n\r\n\r", number, (int)cpuCycles); 00095 } 00096 /*---------------------------------------------------------------------------- 00097 MAIN function 00098 *----------------------------------------------------------------------------*/ 00099 /** 00100 * @brief Main function 00101 * 00102 * Detailed description of the main 00103 */ 00104 int main(void){ 00105 00106 volatile int r, j=0; 00107 00108 double timer_read = 0.0; 00109 double sum = 0; 00110 00111 pc.printf("/*----------------------------------------------------------------------------*/\r\n\r\n"); 00112 pc.printf("Frequency of KL25Z (ARM Cortex M0+): 48 MHZ\n\r\n\r"); 00113 00114 timer.start(); 00115 00116 timer.reset(); 00117 r = my_sqrt(2); // should be 1 00118 timer_read = timer.read(); 00119 printCpuCycles(timer_read, 2, r); 00120 sum = sum + timer_read; 00121 timer.reset(); 00122 00123 00124 00125 r = my_sqrt(4); // should be 2 00126 timer_read = timer.read(); 00127 printCpuCycles(timer_read, 4, r); 00128 sum = sum + timer_read; 00129 timer.reset(); 00130 00131 00132 00133 r = my_sqrt(22); // should be 4 00134 timer_read = timer.read(); 00135 printCpuCycles(timer_read, 22, r); 00136 sum = sum + timer_read; 00137 timer.reset(); 00138 00139 r = my_sqrt(121); // should be 11 00140 timer_read = timer.read(); 00141 printCpuCycles(timer_read, 121, r); 00142 sum = sum + timer_read; 00143 00144 pc.printf("Total time of operation: %f\n\r\n\r", sum); 00145 sum = sum * 48000000; 00146 pc.printf("CPU cycles: %d\n\r\n\r", (int)(sum)); 00147 00148 pc.printf("/*----------------------------------------------------------------------------*/\r\n\r\n"); 00149 00150 } 00151 00152 // *******************************ARM University Program Copyright © ARM Ltd 2014*************************************/ 00153
Generated on Sun Jul 24 2022 19:26:35 by
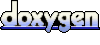