
Heart rate monitor keeps you informed and indeed helps you monitor your heart rate all the time with which you can protect yourself from being prone to heart attacks or any abnormal heart functionality.
Dependencies: ESP8266 GroveEarbudSensor mbed
Fork of Heart-rate-monitor by
main.cpp
00001 #include "mbed.h" 00002 #include "ESP8266.h" 00003 #include <string> 00004 #include <stdio.h> 00005 #include "GroveEarbudSensor.h" 00006 #define APIKEY SJYFHXQEDBQ75N91 //Put "Write key" of your channel in thingspeak.com 00007 #define IP "184.106.153.149" // IP Address of "api.thingspeak.com\" 00008 #define WIFI_SSID "vivo 1601" 00009 #define WIFI_PASS "bschitta" 00010 00011 00012 char snd[255],rcv[1000],snd_Data[255]; 00013 float hrt; 00014 00015 ESP8266 esp(PTC17, PTC16, 115200); // baud rate for wifi 00016 Serial pc(USBTX, USBRX); 00017 00018 // callback for receiving heartrate values 00019 void heartrateCallback(float heartrate,void *data) 00020 { 00021 printf("Callback: heartrate = %.1f\r\n",heartrate); 00022 } 00023 00024 // Blinky 00025 DigitalOut led(LED1); 00026 00027 // Our sensor as an InterruptIn 00028 InterruptIn sensor(D2); 00029 00030 GroveEarbudSensor earbud(&sensor); 00031 00032 void esp_initialize(void); 00033 void esp_send(void); 00034 00035 int main(void) 00036 { 00037 pc.baud(115200); 00038 esp_initialize(); 00039 00040 // announce 00041 printf("Grove Earbud Sensor Example v1.0.0\r\n"); 00042 00043 // allocate the earbud sensor 00044 printf("Allocating earbud sensor instance...\r\n"); 00045 00046 // register our callback function 00047 printf("registering callback...\r\n"); 00048 earbud.registerCallback(heartrateCallback); 00049 00050 // begin main loop 00051 printf("Beginning main loop...\r\n"); 00052 while (true) 00053 { 00054 // blink... 00055 led = !led; 00056 wait(1); 00057 00058 // we can also call directly 00059 printf("Direct: heartrate = %.1f\r\n", earbud.getHeartRate()); 00060 00061 esp_send(); 00062 } 00063 } 00064 00065 void esp_initialize(void) 00066 { 00067 //AT+CWJAP="vivo 1601","bschitta" ; 00068 00069 pc.printf("Initializing ESP\r\n"); 00070 pc.printf("Reset ESP\r\n"); 00071 esp.Reset(); //RESET ESP 00072 esp.RcvReply(rcv, 400); //receive a response from ESP 00073 wait(2); 00074 00075 strcpy(snd,"AT"); 00076 esp.SendCMD(snd); 00077 pc.printf(snd); 00078 esp.RcvReply(rcv, 400); 00079 pc.printf(rcv); 00080 wait(2); 00081 00082 strcpy(snd,"AT+CWMODE=1"); 00083 esp.SendCMD(snd); 00084 pc.printf(snd); 00085 wait(2); 00086 00087 strcpy(snd,"AT+CWJAP=\""); 00088 strcat(snd,WIFI_SSID); 00089 strcat(snd,"\",\""); 00090 strcat(snd,WIFI_PASS); 00091 strcat(snd,"\""); 00092 00093 esp.SendCMD(snd); 00094 pc.printf(snd); 00095 wait(5); 00096 esp.RcvReply(rcv, 400); 00097 pc.printf("\n %s \n", rcv); 00098 00099 strcpy(snd,"AT+CIPMUX=1"); 00100 esp.SendCMD(snd); 00101 pc.printf(snd); 00102 esp.RcvReply(rcv, 400); 00103 pc.printf("\n %s \n", rcv); 00104 } 00105 00106 void esp_send(void) 00107 { 00108 //ESP updates the Status of Thingspeak channel// 00109 00110 strcpy(snd,"AT+CIPSTART="); 00111 strcat(snd,"\"TCP\",\""); 00112 strcat(snd,IP); 00113 strcat(snd,"\",80"); 00114 00115 esp.SendCMD(snd); 00116 pc.printf("Send\r\n%s",snd); 00117 esp.RcvReply(rcv, 1000); 00118 pc.printf("Receive\r\n%s",rcv); 00119 wait(2); 00120 00121 hrt = earbud.getHeartRate(); 00122 pc.printf("Sending this information to thingspeak.com \r\n"); 00123 sprintf(snd,"GET https://api.thingspeak.com/update?api_key=SJYFHXQEDBQ75N91&field1=%f\r\n", hrt); 00124 //wait(5); 00125 //https://api.thingspeak.com/update?api_key=SJYFHXQEDBQ75N91&field1=%f\r\n", hrt 00126 00127 int i=0; 00128 for(i=0; snd[i]!='\0'; i++); 00129 i++; 00130 char cmd[255]; 00131 00132 sprintf(cmd,"AT+CIPSEND=%d",i); //Send Number of open connection and Characters to send 00133 esp.SendCMD(cmd); 00134 pc.printf("Send\r\n%s",cmd); 00135 while(i<=20 || rcv == ">") 00136 { 00137 esp.RcvReply(rcv, 1000); 00138 wait(100); 00139 i++; 00140 } 00141 pc.printf("Receive\r\n%s",rcv); 00142 00143 esp.SendCMD(snd); //Post value to thingspeak channel 00144 pc.printf("Send\r\n%s",snd); 00145 00146 while(i<=20 || rcv == "OK") 00147 { 00148 esp.RcvReply(rcv, 1000); 00149 wait(100); 00150 i++; 00151 } 00152 pc.printf("Receive\r\n%s",rcv); 00153 00154 } 00155 00156
Generated on Sun Jul 17 2022 19:21:45 by
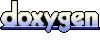