Library to wrapper most of the functions on the MPL3115A2 pressure and temperature sensor.
Dependents: WeatherBalloon4180 WeatherBalloon4180 mbed_rifletool Smart_Watch_4180_Final_Design ... more
Pressure.cpp
00001 #include "Pressure.h" 00002 #include "mbed.h" 00003 00004 Pressure::Pressure() 00005 { 00006 _pressure = 0.0; 00007 00008 _compressed[0] = 0; 00009 _compressed[1] = 0; 00010 _compressed[2] = 0; 00011 } 00012 00013 Pressure::Pressure(float a, unitsType units) 00014 { 00015 setPressure(a, units); 00016 } 00017 00018 Pressure::Pressure(const char* compressed) 00019 { 00020 setPressure(compressed); 00021 } 00022 00023 Pressure::Pressure(const char msb, const char csb, const char lsb) 00024 { 00025 setPressure(msb, csb, lsb); 00026 } 00027 00028 void Pressure::setPressure() 00029 { 00030 setPressure(_compressed[0], _compressed[1], _compressed[2]); 00031 } 00032 00033 void Pressure::setPressure(float a, unitsType units) 00034 { 00035 // TODO: 00036 } 00037 00038 void Pressure::setPressure(const char* compressed) 00039 { 00040 setPressure(compressed[0], compressed[1], compressed[2]); 00041 } 00042 00043 void Pressure::setPressure(const char msb, const char csb, const char lsb) 00044 { 00045 long pressure_whole = (long)msb<<16 | (long)csb<<8 | (long)lsb; 00046 pressure_whole >>= 6; // Pressure is an 18 bit number with 2 bits of decimal. Get rid of decimal portion. 00047 00048 float pressure_decimal = (float)((lsb&0x30)>>4)/4.0; // Turn it into fraction 00049 00050 _pressure = (float)pressure_whole + pressure_decimal; 00051 } 00052 00053 float Pressure::pressure(unitsType units) 00054 { 00055 // http://www.asknumbers.com/ 00056 switch (units) 00057 { 00058 case PSI: 00059 return _pressure * 0.000145037738; 00060 case INHG: 00061 return _pressure * 0.00029529983071; 00062 case MMHG: 00063 return _pressure * 0.007500615613; 00064 } 00065 00066 return _pressure; 00067 } 00068 00069 const char* Pressure::print(unitsType units) 00070 { 00071 sprintf(_printBuffer, "%.0f", pressure(units)); 00072 return _printBuffer; 00073 }
Generated on Tue Jul 12 2022 19:49:10 by
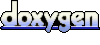